Working with Android Libraries: Enhancing App Functionality
As the Android ecosystem continues to evolve, developers have more tools at their disposal to create powerful and feature-rich mobile applications. One of the key advantages of Android development is the extensive collection of libraries available, which offer pre-built solutions for common tasks and challenges. These libraries can significantly enhance app functionality, streamline development, and save time and effort.
In this blog, we will delve into the world of Android libraries, exploring their benefits and how to integrate them into your projects effectively. We’ll cover a range of libraries, from UI components to networking, database management, and more. Additionally, we’ll provide code samples and best practices to help you harness the true potential of these libraries.
I. Understanding Android Libraries
Before we dive into the implementation details, let’s understand what Android libraries are and why they are valuable for app development.
1.1. What are Android Libraries?
Android libraries are collections of pre-written code that solve specific problems or offer functionalities commonly needed in Android app development. They serve as reusable components that you can easily integrate into your project, saving you from reinventing the wheel and accelerating development.
1.2. Benefits of Using Android Libraries
- Accelerated Development: By using well-established libraries, you can significantly speed up the development process, allowing you to focus on the unique aspects of your app.
- Reliable Solutions: Popular Android libraries are widely used and tested by the community, making them reliable and less prone to bugs.
- Community Support: Many libraries have active communities around them, ensuring continuous updates, bug fixes, and improvements.
- Reduced Code Complexity: Libraries abstract complex functionalities, simplifying your codebase and making it easier to maintain.
2. Popular Android Libraries and How to Use Them
Now that we understand the importance of Android libraries, let’s explore some popular ones and learn how to integrate them into your projects.
2.1. Picasso – Image Loading Made Easy
Picasso is a powerful library for image loading and caching. It simplifies the process of loading images from local storage or URLs into ImageView widgets. To use Picasso, follow these steps:
Add the following dependency to your app-level build.gradle file:
gradle implementation 'com.squareup.picasso:picasso:2.71828'
In your code, load an image into an ImageView:
java Picasso.get() .load("https://example.com/image.jpg") .into(imageView);
2.2. Retrofit – Effortless Networking
Retrofit is a widely-used library for making network requests in Android apps. It streamlines the process of communicating with web services and APIs. To get started with Retrofit:
Add the following dependency to your app-level build.gradle file:
gradle implementation 'com.squareup.retrofit2:retrofit:2.9.0'
Define an interface that specifies the API endpoints:
java public interface ApiService { @GET("users/{username}") Call<User> getUser(@Path("username") String username); }
Create a Retrofit instance and make network requests:
java Retrofit retrofit = new Retrofit.Builder() .baseUrl("https://api.example.com/") .addConverterFactory(GsonConverterFactory.create()) .build(); ApiService apiService = retrofit.create(ApiService.class); Call<User> call = apiService.getUser("john_doe"); call.enqueue(new Callback<User>() { @Override public void onResponse(Call<User> call, Response<User> response) { if (response.isSuccessful()) { User user = response.body(); // Handle the user object } else { // Handle error } } @Override public void onFailure(Call<User> call, Throwable t) { // Handle network failure } });
2.3. Room – Simplified Database Management
Room is an Android Architecture Component that provides an abstraction layer over SQLite, making database management easier and more efficient. Follow these steps to integrate Room into your project:
Add the following dependencies to your app-level build.gradle file:
gradle implementation 'androidx.room:room-runtime:2.3.0' annotationProcessor 'androidx.room:room-compiler:2.3.0'
Define your entity class representing a table in the database:
java @Entity(tableName = "users") public class User { @PrimaryKey public long id; @ColumnInfo(name = "name") public String name; // Other fields, getters, and setters }
Create a Room database and DAO (Data Access Object) interface:
java @Database(entities = {User.class}, version = 1) public abstract class AppDatabase extends RoomDatabase { public abstract UserDao userDao(); } @Dao public interface UserDao { @Insert void insert(User user); @Query("SELECT * FROM users WHERE id = :userId") User getUserById(long userId); // Other queries and operations }
Initialize the Room database in your application class:
java public class MyApp extends Application { public static AppDatabase appDatabase; @Override public void onCreate() { super.onCreate(); appDatabase = Room.databaseBuilder(getApplicationContext(), AppDatabase.class, "my-database") .build(); } }
Now you can use the UserDao methods to interact with the database easily.
3. Best Practices for Using Android Libraries
While Android libraries offer immense value, it’s essential to use them wisely to ensure a smooth development experience and app performance.
3.1. Choose Reputable Libraries:
Select well-maintained libraries with a substantial user base and regular updates. This ensures the library is reliable, secure, and compatible with the latest Android versions.
3.2. Mind Your App Size:
Each library you add contributes to your app’s size. Be mindful of the overall size, as larger apps might deter users from installing or updating them.
3.3. Keep Libraries Updated:
Stay up-to-date with library versions to take advantage of bug fixes, performance improvements, and new features.
3.4. Optimize Proguard Rules:
If using Proguard or R8 for code shrinking and obfuscation, make sure to optimize the rules to avoid accidentally removing critical code from libraries.
3.5. Test Thoroughly:
While libraries are tested by many developers, it’s crucial to thoroughly test them in your specific app context to identify any compatibility or performance issues.
Conclusion
Android libraries are powerful tools that can significantly enhance your app’s functionality, improve development efficiency, and provide solutions to common challenges. By intelligently selecting and integrating reputable libraries, you can elevate your Android app to new heights. Remember to stay updated, test rigorously, and always strive to provide the best user experience possible.
In this blog, we covered a few popular libraries, including Picasso for image loading, Retrofit for networking, and Room for database management. However, the Android ecosystem offers a vast array of libraries, so explore and experiment to discover the ones that best fit your project’s needs.
Happy coding and may your Android apps become even more impressive with the power of Android libraries!
Table of Contents
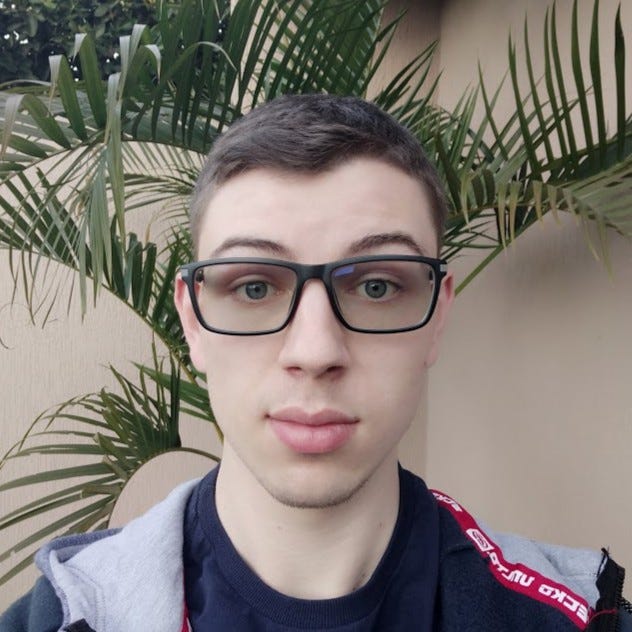
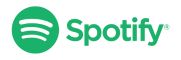