Optimizing Data Display: A Comprehensive Guide to Mastering RecyclerView in Android
For businesses looking to hire Android developers, understanding the fundamentals of components like RecyclerView is essential. Android developers are frequently tasked with displaying large data sets that users can seamlessly scroll and interact with. Their proficiency with RecyclerView, a crucial component that facilitates this task, directly influences the performance and user experience of your application.
In this blog post, we will delve into RecyclerView’s intricacies, offering examples on its usage and optimization for both list and grid displays. This will provide a snapshot of what expertise you should expect when you hire Android developers for your projects.
Understanding RecyclerView
Introduced in Android 5.0 (Lollipop), RecyclerView is a more advanced and flexible version of ListView. It’s a container for displaying large sets of data that can be scrolled efficiently by maintaining a limited number of views. By recycling the view that just went off-screen and using it to display the next data, RecyclerView enhances app performance and responsiveness.
Setup and Basic Usage of RecyclerView
Before delving into our example, ensure you have RecyclerView in your project. This step, which involves adding a specific line to your module-level `build.gradle` file, is fundamental knowledge that any expert Android developer should possess. Therefore, when you’re looking to hire Android developers, their familiarity with such tasks can serve as an effective gauge of their proficiency.
By paying attention to these seemingly small but crucial details, you can ensure you hire Android developers who are capable of efficiently leveraging tools like RecyclerView for your projects.
```java implementation 'androidx.recyclerview:recyclerview:1.2.1'
For basic use, RecyclerView requires an `Adapter` and a `LayoutManager`.
– Adapter: Provides the views for items and binds them to their data.
– LayoutManager: Positions the items.
Let’s take a look at an example using a list of books:
```java public class Book { private String title; private String author; // Getters, Setters, and Constructors are here }
For our `RecyclerView`, we will create a `ViewHolder` and an `Adapter`:
```java public class BookViewHolder extends RecyclerView.ViewHolder { TextView title, author; public BookViewHolder(View itemView) { super(itemView); title = itemView.findViewById(R.id.book_title); author = itemView.findViewById(R.id.book_author); } } public class BookAdapter extends RecyclerView.Adapter<BookViewHolder> { List<Book> bookList; public BookAdapter(List<Book> bookList) { this.bookList = bookList; } @Override public BookViewHolder onCreateViewHolder(ViewGroup parent, int viewType) { View view = LayoutInflater.from(parent.getContext()).inflate(R.layout.book_item, parent, false); return new BookViewHolder(view); } @Override public void onBindViewHolder(BookViewHolder holder, int position) { Book book = bookList.get(position); holder.title.setText(book.getTitle()); holder.author.setText(book.getAuthor()); } @Override public int getItemCount() { return bookList.size(); } }
Finally, in your `Activity` or `Fragment`, initialize the RecyclerView:
```java List<Book> books = getBooks(); // Your method to get books RecyclerView recyclerView = findViewById(R.id.recycler_view); recyclerView.setLayoutManager(new LinearLayoutManager(this)); recyclerView.setAdapter(new BookAdapter(books));
Grid Layout with RecyclerView
To display items in a grid, we use the `GridLayoutManager` instead of the `LinearLayoutManager`. You can specify the number of columns as a parameter:
RecyclerView recyclerView = findViewById(R.id.recycler_view); recyclerView.setLayoutManager(new GridLayoutManager(this, 2)); recyclerView.setAdapter(new BookAdapter(books));
Optimizing RecyclerView
A well-optimized RecyclerView will improve your app’s performance, especially when handling large data sets. Below are some best practices to follow:
1. Use DiffUtil for data updates
Traditionally, if the dataset changes, you call `notifyDataSetChanged()`. However, this refreshes the entire list. Instead, use `DiffUtil`, which calculates the changes and updates only those items:
```java DiffUtil.DiffResult diffResult = DiffUtil.calculateDiff(new BookDiffCallback(oldList, newList)); bookAdapter.setBookList(newList); diffResult.dispatchUpdatesTo(bookAdapter);
2. Use View Binding
View Binding is a safer and more efficient way to access views:
```java public class BookViewHolder extends RecyclerView.ViewHolder { ItemBookBinding binding; public BookViewHolder(ItemBookBinding binding) { super(binding.getRoot()); this.binding = binding; } } @Override public BookViewHolder onCreateViewHolder(ViewGroup parent, int viewType) { ItemBookBinding binding = ItemBookBinding.inflate(LayoutInflater.from(parent.getContext()), parent, false); return new BookViewHolder(binding); } @Override public void onBindViewHolder(BookViewHolder holder, int position) { Book book = bookList.get(position); holder.binding.bookTitle.setText(book.getTitle()); holder.binding.bookAuthor.setText(book.getAuthor()); }
3. Implement ViewHolder patterns
Make your list scrolling smoother by avoiding findViewById() calls in `onBindViewHolder()` by using a `ViewHolder`.
4. Enable HasFixedSize
If you know the RecyclerView’s size doesn’t change, you can improve performance by setting `setHasFixedSize(true)`.
```java recyclerView.setHasFixedSize(true);
5. Use `onFailedToRecycleView`
When a ViewHolder isn’t recyclable, override `onFailedToRecycleView()` in the adapter and return `true` to force recycling.
Conclusion
RecyclerView is a vital component in Android, offering a flexible and efficient way to display large sets of data. Android developers, especially those you might seek to hire, should be well-versed in practices such as using `DiffUtil`, view binding, and ViewHolder patterns to optimize its performance. Mastery over RecyclerView directly translates to the ability to create responsive, smooth-running Android apps – a skill that businesses should look for when they hire Android developers. This component’s mastery is a clear indication of a developer’s potential to create high-performing apps, making it a crucial area of focus when you are looking to hire Android developers.
Table of Contents
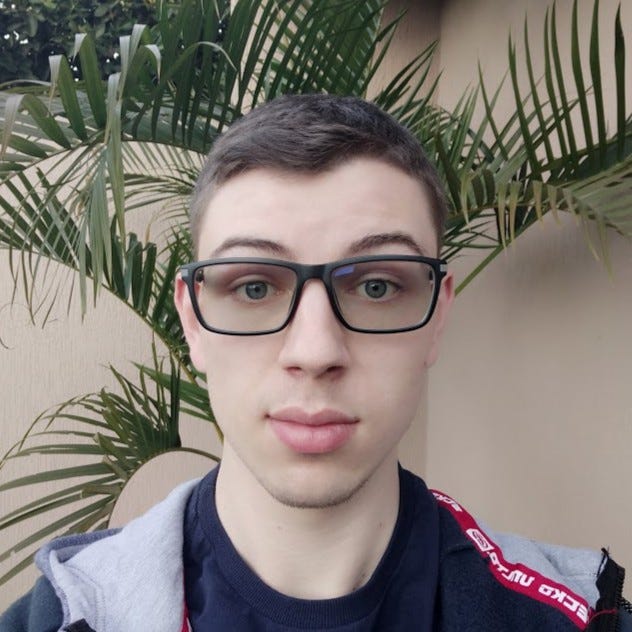
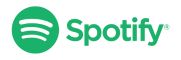