Elevate Your Android App: Essential Guide to Music & Video Streaming
The digital age has redefined how we consume content. With the rise of smartphones, we now have the convenience to watch videos and listen to music from anywhere. For businesses looking to tap into this trend, it’s crucial to hire Android developers. These professionals can seamlessly integrate media playback into apps, significantly enhancing the user experience. Let’s delve into building music and video streaming apps using Android’s `MediaPlayer` class.
Table of Contents
1. Understanding the MediaPlayer class
The Android platform provides a set of APIs in the form of the `MediaPlayer` class to play audio and video files. This class can be used to play both local files and streams over the network.
Some of its capabilities include:
- Playback of audio/video.
- Pausing, resuming, and stopping playback.
- Fetching metadata.
- Handling streaming from online sources.
2. Building a Basic Music Streaming App
Step 1: Set Up Permissions
Before integrating the media player, ensure you have the necessary permissions in the `AndroidManifest.xml`:
```xml <uses-permission android:name="android.permission.INTERNET" /> <uses-permission android:name="android.permission.READ_EXTERNAL_STORAGE" /> ```
Step 2: Initialize the MediaPlayer
```java MediaPlayer mediaPlayer = new MediaPlayer(); mediaPlayer.setAudioStreamType(AudioManager.STREAM_MUSIC); ```
Step 3: Set the Data Source
To play a remote file:
```java mediaPlayer.setDataSource("http://example.com/sample.mp3"); ```
To play a local file:
```java mediaPlayer.setDataSource(getApplicationContext(), Uri.parse("file:///path/to/local/file.mp3")); ```
Step 4: Prepare and Start Playback
```java mediaPlayer.prepare(); mediaPlayer.start(); ```
Step 5: Handle Lifecycle Methods
Ensure you release resources when not in use:
```java @Override protected void onDestroy() { if(mediaPlayer != null) { mediaPlayer.release(); mediaPlayer = null; } super.onDestroy(); } ```
3. Building a Video Streaming App
Much of the process for building a video player is similar. However, for video playback, you also need a `SurfaceView` or `TextureView` for rendering.
Step 1: Add the SurfaceView in your Layout
```xml <SurfaceView android:id="@+id/surfaceView" android:layout_width="match_parent" android:layout_height="wrap_content" /> ```
Step 2: Initialize MediaPlayer and Set SurfaceView
```java MediaPlayer mediaPlayer = new MediaPlayer(); SurfaceView surfaceView = findViewById(R.id.surfaceView); SurfaceHolder holder = surfaceView.getHolder(); holder.addCallback(new SurfaceHolder.Callback() { @Override public void surfaceCreated(SurfaceHolder holder) { mediaPlayer.setDisplay(holder); } @Override public void surfaceChanged(SurfaceHolder holder, int format, int width, int height) {} @Override public void surfaceDestroyed(SurfaceHolder holder) {} }); ```
The subsequent steps, like setting the data source, preparing the media player, and starting playback, remain the same.
Examples
- Custom Controls: Enhance user experience by adding play, pause, and stop buttons. Handle their onClick events to control the `MediaPlayer`.
- Buffering Feedback: Especially for streaming, it’s a good idea to show a buffering spinner. Use `mediaPlayer.setOnBufferingUpdateListener` to monitor the buffering process.
- Error Handling: Use `mediaPlayer.setOnErrorListener` to handle potential playback errors gracefully.
4. Advanced Tips
– ExoPlayer: For more complex media tasks, consider using ExoPlayer. It’s a more powerful, flexible alternative to `MediaPlayer`, offering features like adaptive streaming.
– Background Playback: If you want your audio to play in the background, consider using a foreground service along with `MediaPlayer`.
– Caching: For improved performance, especially in cases of repeated playback, consider caching media files.
Conclusion
Building music and video streaming apps on Android is straightforward with the `MediaPlayer` class. With the basic foundation covered in this blog, those who hire Android developers will find it easier to further expand upon these concepts, adding features and enhancing user experience. The potential for creating a truly immersive media experience on Android is vast, so let your creativity and technical expertise shine!
Table of Contents
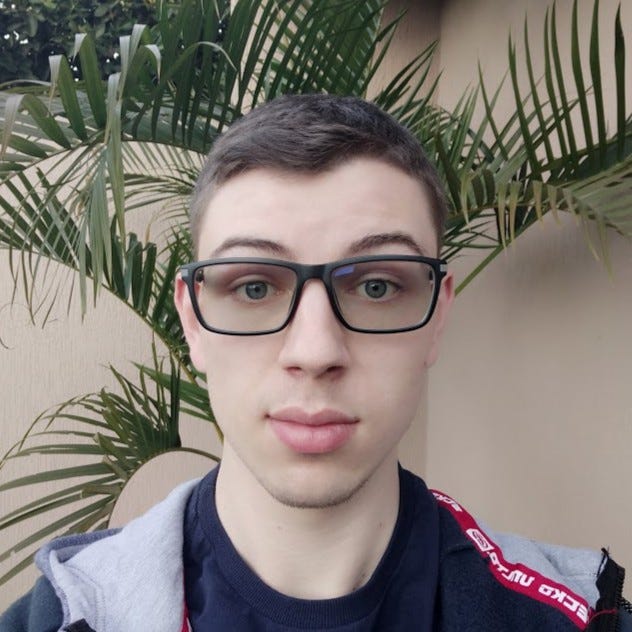
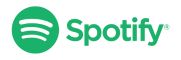