How do I make network requests using Retrofit in Android?
Making network requests using Retrofit in Android is a breeze, thanks to its intuitive API and seamless integration with RESTful APIs. Here’s a step-by-step guide on how to leverage Retrofit to perform network requests in your Android application:
Add Retrofit Dependency: Start by adding the Retrofit dependency to your app’s build.gradle file. Ensure that you include the Retrofit library along with any additional converters or adapters you may need, such as Gson converter for JSON serialization/deserialization.
implementation 'com.squareup.retrofit2:retrofit:2.9.0' implementation 'com.squareup.retrofit2:converter-gson:2.9.0' // Replace with the converter you need
Define API Interface: Create a Java interface that defines your RESTful API endpoints using Retrofit annotations. Annotate each method with the HTTP request method (GET, POST, PUT, DELETE, etc.) and the relative URL path.
public interface ApiService { @GET("posts/{id}") Call<Post> getPost(@Path("id") int postId); }
Create Retrofit Instance: Instantiate a Retrofit object with the base URL of your API and configure it with any necessary converters, interceptors, or client settings.
Retrofit retrofit = new Retrofit.Builder() .baseUrl("https://jsonplaceholder.typicode.com/") .addConverterFactory(GsonConverterFactory.create()) .build();
Create API Service Instance: Use the Retrofit object to create an instance of your API interface.
ApiService apiService = retrofit.create(ApiService.class);
Make Network Requests: Use the methods defined in your API interface to make network requests asynchronously. Retrofit uses Call objects to represent asynchronous HTTP requests and responses.
Call<Post> call = apiService.getPost(1); call.enqueue(new Callback<Post>() { @Override public void onResponse(Call<Post> call, Response<Post> response) { if (response.isSuccessful()) { // Handle successful response Post post = response.body(); } else { // Handle error response } } @Override public void onFailure(Call<Post> call, Throwable t) { // Handle network failure } });
Handle Response: Implement onResponse() and onFailure() methods to handle successful responses and network failures, respectively. Process the response data as needed and update your app’s UI or perform any necessary business logic.
And that’s it! With these simple steps, you can harness the power of Retrofit to perform network requests and interact with RESTful APIs in your Android application. Retrofit’s elegant API design and seamless integration make it a popular choice among Android developers for building robust and efficient network communication layers in their apps.
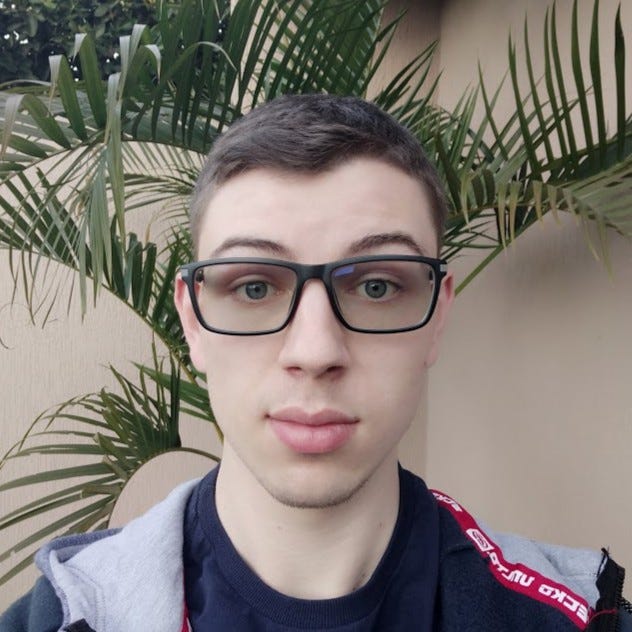
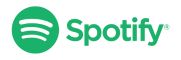