Networking in Android: Making HTTP Requests and Handling Responses
Networking plays a crucial role in modern mobile applications, enabling seamless data exchange between the user’s device and remote servers. In Android development, making HTTP requests and handling responses efficiently is essential for providing a smooth user experience. This blog post will guide you through the process of making HTTP requests and effectively managing the responses in an Android application. We will explore various techniques and provide code samples to help you integrate networking capabilities into your Android app.
1. Introduction to Android Networking
Android provides robust networking capabilities that allow developers to communicate with remote servers using various protocols like HTTP, HTTPS, and WebSocket. Networking operations are typically performed asynchronously to avoid blocking the main UI thread and ensure a responsive user interface. This blog post will focus on HTTP requests, which are widely used for data exchange in Android apps.
2. Performing HTTP Requests
2.1 Using HttpURLConnection:
The HttpURLConnection class is a built-in Java API that provides basic HTTP functionality. It allows developers to establish connections, set request headers, send request bodies, and retrieve response data. Here’s an example of making an HTTP GET request using HttpURLConnection:
java try { URL url = new URL("https://api.example.com/data"); HttpURLConnection connection = (HttpURLConnection) url.openConnection(); connection.setRequestMethod("GET"); int responseCode = connection.getResponseCode(); if (responseCode == HttpURLConnection.HTTP_OK) { InputStream inputStream = connection.getInputStream(); // Process the response } else { // Handle error cases } } catch (IOException e) { e.printStackTrace(); }
2.2 Utilizing OkHttp Library:
OkHttp is a popular open-source HTTP client library for Android. It provides a simpler and more concise API compared to HttpURLConnection. To use OkHttp, add the following dependency to your app’s build.gradle file:
groovy implementation 'com.squareup.okhttp3:okhttp:4.9.1'
Here’s an example of making an HTTP GET request using OkHttp:
java OkHttpClient client = new OkHttpClient(); Request request = new Request.Builder() .url("https://api.example.com/data") .build(); try (Response response = client.newCall(request).execute()) { if (response.isSuccessful()) { String responseBody = response.body().string(); // Process the response } else { // Handle error cases } } catch (IOException e) { e.printStackTrace(); }
2.3 Apache HttpClient:
Apache HttpClient is another popular library for making HTTP requests in Android apps. It provides a rich set of features and customization options. To use Apache HttpClient, add the following dependency to your app’s build.gradle file:
groovy android { useLibrary 'org.apache.http.legacy' } Here's an example of making an HTTP GET request using Apache HttpClient: java HttpClient httpClient = new DefaultHttpClient(); HttpGet httpGet = new HttpGet("https://api.example.com/data"); try { HttpResponse response = httpClient.execute(httpGet); if (response.getStatusLine().getStatusCode() == HttpStatus.SC_OK) { HttpEntity entity = response.getEntity(); String responseBody = EntityUtils.toString(entity); // Process the response } else { // Handle error cases } } catch (IOException e) { e.printStackTrace(); }
2.4 Retrofit Library:
Retrofit is a widely used HTTP client library that simplifies the process of making network requests by providing a high-level, declarative API. It also supports request/response serialization and deserialization using converters like Gson or Moshi. To use Retrofit, add the following dependency to your app’s build.gradle file:
groovy implementation 'com.squareup.retrofit2:retrofit:2.9.0' Here's an example of making an HTTP GET request using Retrofit: java public interface ApiService { @GET("data") Call<DataResponse> getData(); } Retrofit retrofit = new Retrofit.Builder() .baseUrl("https://api.example.com/") .build(); ApiService apiService = retrofit.create(ApiService.class); Call<DataResponse> call = apiService.getData(); call.enqueue(new Callback<DataResponse>() { @Override public void onResponse(Call<DataResponse> call, Response<DataResponse> response) { if (response.isSuccessful()) { DataResponse data = response.body(); // Process the response } else { // Handle error cases } } @Override public void onFailure(Call<DataResponse> call, Throwable t) { t.printStackTrace(); } });
3. Handling HTTP Responses
3.1 Parsing JSON Responses:
JSON is a commonly used format for transmitting structured data over the network. To parse JSON responses in Android, you can use libraries like Gson or Moshi. Here’s an example using Gson:
java implementation 'com.google.code.gson:gson:2.8.8' java Gson gson = new Gson(); DataResponse data = gson.fromJson(responseBody, DataResponse.class);
3.2 Handling Error Cases:
HTTP responses can include error codes and messages to indicate various failure scenarios. It’s important to handle these cases gracefully in your Android app. You can check the HTTP response code or parse the error message from the response body to determine the appropriate action.
3.3 Asynchronous Response Handling:
Performing network operations on the main UI thread can cause the app to become unresponsive. To avoid this, Android provides various mechanisms for asynchronous response handling, such as AsyncTask, Handler, or using libraries like RxJava or Coroutines. These approaches help keep the UI thread free while the network operation is in progress.
4. Advanced Networking Techniques
4.1 Managing Network Connectivity:
It’s crucial to handle changes in network connectivity to ensure a reliable and uninterrupted user experience. You can use the ConnectivityManager class to monitor network state and adapt your app’s behavior accordingly.
4.2 Implementing Request Caching:
Caching network responses can significantly improve app performance and reduce bandwidth usage. Android provides a built-in caching mechanism that you can enable and configure for your app’s HTTP requests.
4.3 Securing Network Communication:
Secure communication over the network is vital for protecting user data and preventing unauthorized access. Android supports various security mechanisms like SSL/TLS and certificate pinning to establish secure connections between the app and the server.
Conclusion
In this blog post, we explored the essentials of networking in Android and learned how to make HTTP requests and handle responses efficiently. We covered different approaches using built-in APIs and popular libraries like OkHttp and Retrofit. Additionally, we discussed advanced techniques such as parsing JSON responses, handling errors, and implementing asynchronous response handling. By incorporating these techniques into your Android app, you can create robust networking capabilities and provide a seamless data communication experience for your users.
Table of Contents
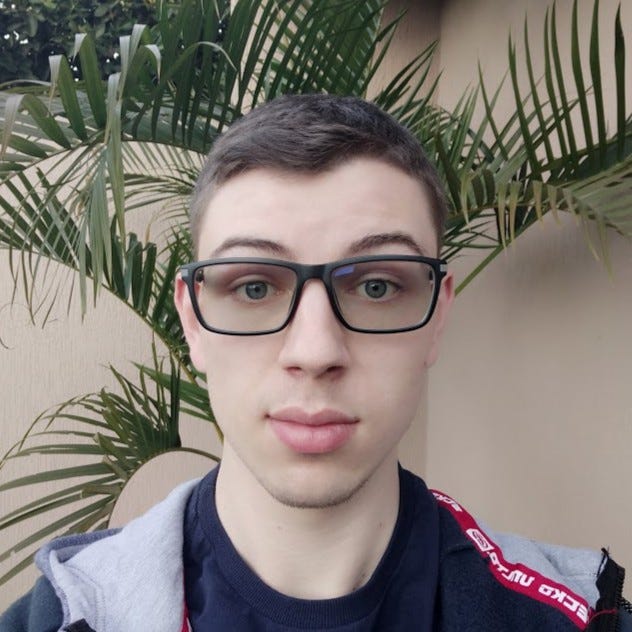
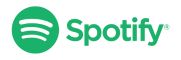