Android Notifications: Engaging Users with Timely Updates
Android notifications are a vital tool for engaging users with timely updates. In today’s fast-paced digital world, users expect to be informed and alerted in real-time about important events, messages, and updates from their favorite apps. Android notifications provide a seamless way to deliver these updates directly to users’ devices, ensuring that they stay connected and engaged.
1. Anatomy of an Android Notification
To understand how to create engaging notifications, let’s first explore the different components of an Android notification.
1.1 Notification Content
The content of a notification includes the title, text, and other relevant information that the user sees when the notification is displayed. It should be concise, clear, and provide relevant information that captures the user’s attention. The content should be crafted in a way that entices the user to take action or engage with the notification further.
1.2 Notification Actions
Notification actions allow users to perform specific actions directly from the notification itself, without having to open the app. Common examples include replying to a message, dismissing a reminder, or playing/pausing media. By providing actionable buttons, you can enhance the user experience and make it more convenient for users to interact with your app.
1.3 Notification Styles
Android offers various notification styles to customize the appearance of your notifications. Some popular styles include Big Picture, Big Text, Inbox, and Messaging. These styles allow you to present the information in a visually appealing way, increasing the chances of capturing the user’s attention and encouraging engagement.
2. Implementing Basic Android Notifications
Now that we understand the components of an Android notification, let’s dive into the implementation.
2.1 Setting Up the Notification Channel
Starting from Android Oreo (API level 26) and above, notifications are grouped into channels, allowing users to have granular control over their notification preferences. To set up a notification channel, you need to create an instance of the NotificationChannel class and specify its properties, such as the channel ID, name, description, and importance level. Then, register the channel with the NotificationManager system service.
java // Create the notification channel NotificationChannel channel = new NotificationChannel( CHANNEL_ID, "My Channel", NotificationManager.IMPORTANCE_DEFAULT ); // Set additional properties channel.setDescription("My Channel Description"); // Register the channel with the system NotificationManager notificationManager = getSystemService(NotificationManager.class); notificationManager.createNotificationChannel(channel);
2.2 Creating a Simple Notification
To create a simple notification, use the NotificationCompat.Builder class and set the essential properties such as the title, text, and icon. Finally, call the build() method to obtain the Notification object and use the NotificationManager to display it.
java NotificationCompat.Builder builder = new NotificationCompat.Builder(this, CHANNEL_ID) .setSmallIcon(R.drawable.notification_icon) .setContentTitle("New Message") .setContentText("You have a new message.") .setPriority(NotificationCompat.PRIORITY_DEFAULT); NotificationManagerCompat notificationManager = NotificationManagerCompat.from(this); notificationManager.notify(notificationId, builder.build());
2.3 Customizing the Notification Appearance
To customize the appearance of your notification, you can modify various attributes such as the color, large icon, and sound. For example, to set the notification color, use the setColor() method:
java NotificationCompat.Builder builder = new NotificationCompat.Builder(this, CHANNEL_ID) .setSmallIcon(R.drawable.notification_icon) .setContentTitle("New Message") .setContentText("You have a new message.") .setColor(ContextCompat.getColor(this, R.color.notification_color)) .setPriority(NotificationCompat.PRIORITY_DEFAULT); // ... Additional customization notificationManager.notify(notificationId, builder.build());
3. Enhancing Notifications with Advanced Features
While basic notifications are useful, incorporating advanced features can significantly enhance the user experience.
3.1 Expanding Notifications
Expanding notifications allow you to show more content to users directly from the notification. By default, notifications are collapsed, showing only basic information. However, by adding a large icon or an expandable text area, you can provide users with additional details without requiring them to open the app.
java NotificationCompat.Builder builder = new NotificationCompat.Builder(this, CHANNEL_ID) .setSmallIcon(R.drawable.notification_icon) .setContentTitle("New Message") .setContentText("You have a new message.") .setStyle(new NotificationCompat.BigTextStyle() .bigText("This is a long message that can be expanded to show more content.")); // ... Additional customization notificationManager.notify(notificationId, builder.build());
3.2 Adding Action Buttons
Action buttons enable users to perform specific actions directly from the notification. For instance, you can include buttons for “Reply,” “Dismiss,” or “Play/Pause.” By providing these options upfront, you streamline the user’s interaction with your app.
java NotificationCompat.Builder builder = new NotificationCompat.Builder(this, CHANNEL_ID) .setSmallIcon(R.drawable.notification_icon) .setContentTitle("New Message") .setContentText("You have a new message.") .addAction(R.drawable.ic_reply, "Reply", replyPendingIntent) .addAction(R.drawable.ic_dismiss, "Dismiss", dismissPendingIntent); // ... Additional customization notificationManager.notify(notificationId, builder.build());
3.3 Using Big Picture and Big Text Styles
Big Picture and Big Text styles are powerful tools for visually engaging users with rich media and expanded text content.
java NotificationCompat.Builder builder = new NotificationCompat.Builder(this, CHANNEL_ID) .setSmallIcon(R.drawable.notification_icon) .setContentTitle("New Picture") .setContentText("Check out this beautiful picture!") .setStyle(new NotificationCompat.BigPictureStyle() .bigPicture(pictureBitmap) .bigLargeIcon(null)); // ... Additional customization notificationManager.notify(notificationId, builder.build());
4. Delivering Timely Updates with Scheduled Notifications
To keep users engaged, it’s essential to deliver timely updates. Android provides the AlarmManager and NotificationManager classes to schedule and deliver notifications at specific times or intervals.
4.1 Setting Up the AlarmManager
First, set up the AlarmManager to trigger a notification at the desired time. Use the set() method to specify the trigger time and the PendingIntent that will be fired when the alarm is triggered.
java AlarmManager alarmManager = (AlarmManager) getSystemService(Context.ALARM_SERVICE); Intent intent = new Intent(this, MyNotificationReceiver.class); PendingIntent pendingIntent = PendingIntent.getBroadcast(this, 0, intent, 0); // Set the alarm to trigger after a specific time alarmManager.set(AlarmManager.RTC_WAKEUP, triggerTimeMillis, pendingIntent);
4.2 Scheduling Notifications with the NotificationManager
Inside the MyNotificationReceiver class, implement the onReceive() method, where you can create and display the scheduled notification using the NotificationManager.
java public class MyNotificationReceiver extends BroadcastReceiver { @Override public void onReceive(Context context, Intent intent) { // Create and display the scheduled notification // ... } }
5. Best Practices for Engaging Notifications
To make the most of Android notifications and truly engage users, consider the following best practices:
5.1 Personalization and Context
Tailor your notifications to the user’s preferences and provide contextually relevant information. Use user data and behavior patterns to personalize the content and timing of your notifications.
5.2 Timing and Frequency
Be mindful of when you send notifications to avoid interrupting users during important tasks or moments. Respect the user’s preferences for quiet hours and allow them to customize the frequency and type of notifications they receive.
5.3 Clear and Actionable Content
Craft concise and clear notification messages that provide relevant information upfront. Make sure the content is actionable and provides users with a reason to engage with your app.
Conclusion
Android notifications are a powerful tool for engaging users with timely updates. By understanding the components of a notification, implementing basic and advanced features, and following best practices, you can create compelling notifications that enhance the user experience and drive engagement with your app. Remember to always consider the user’s preferences and strive to provide personalized, valuable content through your notifications. Harness the potential of Android notifications, and watch your user engagement soar!
Table of Contents
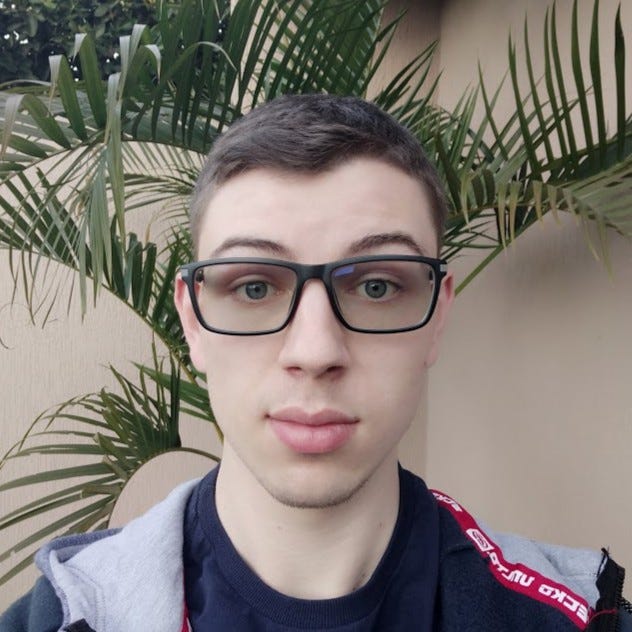
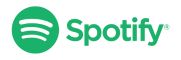