Android Push Notifications: Engaging Users with Firebase Cloud Messaging
In today’s mobile-dominated world, user engagement is the key to a successful app. Whether you have a news app, e-commerce platform, or a social media application, keeping users informed and engaged is crucial for retaining and growing your user base. One of the most effective ways to achieve this is by implementing push notifications. Android Push Notifications, powered by Firebase Cloud Messaging (FCM), allow you to deliver timely and personalized content directly to your users’ devices, enhancing their overall app experience.
In this blog, we will explore the importance of push notifications for Android apps and guide you through the process of integrating Firebase Cloud Messaging into your Android application. By the end of this article, you will have a solid understanding of how to engage users effectively using FCM push notifications, along with relevant code samples and step-by-step instructions.
1. Why Push Notifications Matter:
Before delving into the technical aspects of implementing push notifications in your Android app, it’s essential to understand why they matter and how they can benefit your business.
1.1 Enhance User Retention:
Push notifications act as reminders for users to open and use your app regularly. They keep your app on their radar and improve user retention rates by encouraging repeat visits.
1.2 Personalized User Experience:
With FCM push notifications, you can deliver personalized content based on user preferences, behavior, and location. This personal touch makes users feel valued, leading to a more engaging app experience.
1.3 Timely Updates:
Push notifications enable you to deliver real-time updates, such as breaking news, limited-time offers, or social media notifications. Users appreciate receiving timely and relevant information, which can lead to increased app usage.
1.4 Re-engage Inactive Users:
Through targeted push notifications, you can re-engage users who haven’t interacted with your app for a while. This strategy can bring back dormant users and breathe new life into your app.
2. Setting Up Firebase Cloud Messaging (FCM):
Now that we understand the significance of push notifications, let’s dive into the process of setting up Firebase Cloud Messaging for your Android app.
2.1 Create a Firebase Project:
To get started, navigate to the Firebase Console (https://console.firebase.google.com) and create a new project. Once your project is created, you will receive a unique project ID, which you will need to include in your Android app.
2.2 Add Firebase to Your Android App:
In Android Studio, open your project and go to “Tools” > “Firebase.” Choose “Cloud Messaging” from the list of available Firebase features and follow the on-screen instructions to integrate Firebase Cloud Messaging into your Android app.
2.3 Retrieve FCM Server Key:
To send push notifications to your Android app, you need the FCM Server Key. Go to the Firebase Console, select your project, and navigate to “Project settings” > “Cloud Messaging.” Here, you will find your FCM Server Key, which you’ll use later to send push notifications from your server.
3. Handling Push Notifications in Your Android App:
Now that you have FCM integrated into your Android app, let’s explore how to handle incoming push notifications.
3.1 Set Up the Android Manifest:
To receive push notifications, you need to register your app with FCM. Add the following code snippet to your AndroidManifest.xml file:
xml <manifest ...> <uses-permission android:name="android.permission.INTERNET" /> <application ...> <!-- Add the following service to handle push notifications --> <service android:name=".MyFirebaseMessagingService" android:exported="false"> <intent-filter> <action android:name="com.google.firebase.MESSAGING_EVENT" /> </intent-filter> </service> <!-- Add your FCM Sender ID here --> <meta-data android:name="com.google.firebase.messaging.default_notification_channel_id" android:value="@string/default_notification_channel_id" /> </application> </manifest>
3.2 Create a FirebaseMessagingService:
Next, create a class that extends FirebaseMessagingService to handle incoming push notifications:
java import com.google.firebase.messaging.FirebaseMessagingService; import com.google.firebase.messaging.RemoteMessage; public class MyFirebaseMessagingService extends FirebaseMessagingService { @Override public void onMessageReceived(RemoteMessage remoteMessage) { // Handle incoming push notifications here } }
3.3 Displaying Notifications:
When your app receives a push notification, you can create and display a notification in the notification tray. Here’s a code snippet to get you started:
java private void showNotification(String title, String message) { // Create a unique notification channel ID for Android Oreo and above String channelId = "my_channel_id"; NotificationManager notificationManager = getSystemService(NotificationManager.class); if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.O) { NotificationChannel channel = new NotificationChannel( channelId, "My Channel", NotificationManager.IMPORTANCE_DEFAULT ); notificationManager.createNotificationChannel(channel); } NotificationCompat.Builder builder = new NotificationCompat.Builder(this, channelId) .setSmallIcon(R.drawable.ic_notification) .setContentTitle(title) .setContentText(message) .setPriority(NotificationCompat.PRIORITY_DEFAULT); notificationManager.notify(0, builder.build()); }
4. Sending Push Notifications from Your Server:
To send push notifications to your Android app users, you’ll need to use the FCM Server Key we retrieved earlier.
4.1 HTTP Request to FCM Endpoint:
You can send HTTP POST requests to the FCM endpoint to deliver push notifications. The following code sample demonstrates how to send a notification using cURL:
bash curl -X POST -H "Authorization: key=<YOUR_FCM_SERVER_KEY>" -H "Content-Type: application/json" -d '{ "to": "<DEVICE_TOKEN>", "data": { "title": "New Message", "body": "You have a new message!" } }' "https://fcm.googleapis.com/fcm/send"
Replace <YOUR_FCM_SERVER_KEY> with your actual FCM Server Key and <DEVICE_TOKEN> with the device token of the recipient’s device, which you obtain when registering for push notifications on the client-side.
5. Advanced Push Notification Strategies:
To maximize the impact of your push notifications, consider implementing the following strategies:
5.1 Segmentation and Personalization:
Segment your user base and send personalized notifications based on user behavior, preferences, or location. Personalized content resonates better with users, leading to increased engagement.
5.2 A/B Testing:
Perform A/B testing with different notification styles, timings, and content to determine the most effective combinations. This will help you refine your notification strategy over time.
5.3 Opt-in and Opt-out Mechanisms:
Respect user preferences by providing opt-in and opt-out mechanisms for push notifications. This ensures that users receive notifications they find relevant while avoiding potential annoyance.
Conclusion
Firebase Cloud Messaging is a powerful tool that enables Android app developers to engage users effectively through push notifications. By providing timely and personalized content, you can enhance user retention, re-engage inactive users, and offer an overall superior app experience. In this blog, we covered the significance of push notifications, the steps to integrate FCM into your Android app, handling incoming notifications, and sending push notifications from your server. Implement these strategies and best practices to keep your users informed, engaged, and coming back for more. Happy coding!
Table of Contents
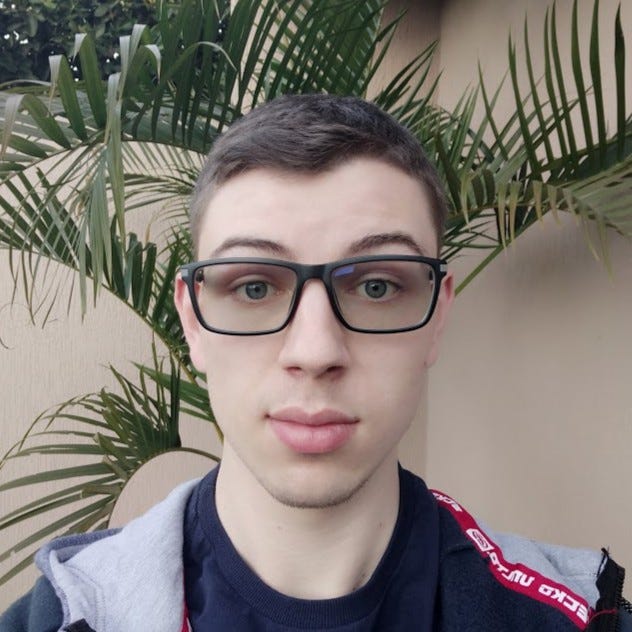
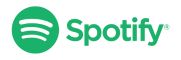