Exploring Android Fragments: Reusable UI Components
In the world of Android app development, building efficient and flexible user interfaces is crucial. Android Fragments are a key tool in achieving this goal, enabling developers to create reusable UI components that can be combined and rearranged to fit various screen sizes and device orientations. This blog will delve into the concept of Android Fragments, their benefits, and how to implement them effectively in your Android app.
1. Introduction to Android Fragments
Android Fragments were introduced in Android 3.0 (Honeycomb) to address the challenge of creating responsive user interfaces for various screen sizes and orientations. A Fragment represents a reusable portion of an activity’s UI, allowing you to build more modular and flexible designs. Unlike activities, which are tied to a specific screen, fragments can be combined and reassembled to adapt to different layouts, making them ideal for supporting both smartphones and tablets with a consistent user experience.
2. Benefits of Using Android Fragments
2.1. Reusability and Modularity
Fragments encapsulate UI elements and their behavior, making them highly reusable across different activities and layouts. This modularity promotes cleaner code architecture and enhances code maintainability.
2.2. Screen Adaptation
By utilizing fragments, you can create adaptive layouts that automatically adjust based on the available screen space and orientation, providing a seamless experience across various devices.
2.3. Code Separation and Organization
Using fragments, you can divide your UI logic into smaller pieces, promoting a clear separation of concerns, easier debugging, and a more organized codebase.
2.4. Efficient Memory Management
Fragments have their own lifecycle, allowing the system to handle memory more efficiently. When a fragment is no longer needed, it can be removed or detached, freeing up resources for other components.
3. Anatomy of an Android Fragment
Before diving into the implementation, let’s understand the key components that make up an Android Fragment.
3.1. Lifecycle of Fragments
Fragments have their lifecycle, similar to activities, consisting of several states like onCreate(), onStart(), onResume(), onPause(), onStop(), and onDestroy(). Understanding these lifecycle methods is essential for managing fragment behavior effectively.
4. Creating and Implementing Fragments
Now that we have a grasp of the basics, let’s create and implement a fragment in an Android app.
4.1. Defining a Fragment in XML
Fragments can be defined using XML layouts, just like activities. The XML layout will contain the UI elements and design for the fragment.
xml <!-- fragment_example.xml --> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="match_parent" android:orientation="vertical"> <!-- Add UI elements here --> </LinearLayout>
4.2. Fragment Java Class
Next, we’ll create the Java class for the fragment, where we can handle the fragment’s behavior and interact with its UI elements.
java // ExampleFragment.java public class ExampleFragment extends Fragment { @Override public View onCreateView(LayoutInflater inflater, ViewGroup container, Bundle savedInstanceState) { // Inflate the fragment's layout View view = inflater.inflate(R.layout.fragment_example, container, false); // Perform any additional setup or UI interactions here return view; } }
4.3. Fragment Transaction
To display a fragment within an activity, you need to use FragmentManager and FragmentTransaction to perform the fragment transaction.
java // ExampleActivity.java public class ExampleActivity extends AppCompatActivity { @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_example); // Obtain the FragmentManager FragmentManager fragmentManager = getSupportFragmentManager(); // Start a new FragmentTransaction FragmentTransaction fragmentTransaction = fragmentManager.beginTransaction(); // Replace the fragment_container with the ExampleFragment fragmentTransaction.replace(R.id.fragment_container, new ExampleFragment()); // Commit the transaction fragmentTransaction.commit(); } }
5. Communicating Between Fragments
Often, fragments need to communicate with each other or with their parent activity. Let’s explore how to achieve this communication.
5.1. Sending Data from Activity to Fragment
Passing data from an activity to a fragment can be accomplished using arguments.
java // ExampleActivity.java public class ExampleActivity extends AppCompatActivity { @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_example); String data = "Hello, Fragment!"; FragmentManager fragmentManager = getSupportFragmentManager(); FragmentTransaction fragmentTransaction = fragmentManager.beginTransaction(); // Create an instance of ExampleFragment and set the data as arguments ExampleFragment fragment = new ExampleFragment(); Bundle args = new Bundle(); args.putString("data_key", data); fragment.setArguments(args); fragmentTransaction.replace(R.id.fragment_container, fragment); fragmentTransaction.commit(); } } // ExampleFragment.java public class ExampleFragment extends Fragment { @Override public View onCreateView(LayoutInflater inflater, ViewGroup container, Bundle savedInstanceState) { View view = inflater.inflate(R.layout.fragment_example, container, false); // Retrieve the data from arguments String data = getArguments().getString("data_key"); // Do something with the data return view; } }
5.2. Sending Data from Fragment to Activity
To send data back from a fragment to its parent activity, you can define an interface in the fragment and implement it in the activity.
java // ExampleFragment.java public class ExampleFragment extends Fragment { public interface OnDataChangeListener { void onDataChanged(String newData); } private OnDataChangeListener dataChangeListener; @Override public void onAttach(@NonNull Context context) { super.onAttach(context); try { dataChangeListener = (OnDataChangeListener) context; } catch (ClassCastException e) { throw new ClassCastException(context.toString() + " must implement OnDataChangeListener"); } } // Method to update data and notify the activity private void updateData(String newData) { dataChangeListener.onDataChanged(newData); } //... } // ExampleActivity.java public class ExampleActivity extends AppCompatActivity implements ExampleFragment.OnDataChangeListener { //... @Override public void onDataChanged(String newData) { // Handle the new data received from the fragment }
5.3. Fragment to Fragment Communication
To communicate between two fragments directly, you can use the parent activity as a communication bridge.
java // ExampleFragmentA.java public class ExampleFragmentA extends Fragment { private void sendDataToFragmentB(String data) { ExampleActivity activity = (ExampleActivity) getActivity(); if (activity != null) { ExampleFragmentB fragmentB = (ExampleFragmentB) activity.getSupportFragmentManager().findFragmentByTag("fragment_b_tag"); if (fragmentB != null) { fragmentB.receiveData(data); } } } //... } // ExampleFragmentB.java public class ExampleFragmentB extends Fragment { public void receiveData(String data) { // Handle the data received from Fragment A } //... }
6. Fragment Backstack and Navigation
When dealing with multiple fragments, it’s important to manage the backstack to provide smooth navigation and user experience.
java // To add a fragment to the backstack while performing a transaction FragmentTransaction fragmentTransaction = fragmentManager.beginTransaction(); fragmentTransaction.replace(R.id.fragment_container, new ExampleFragment()); fragmentTransaction.addToBackStack(null); // Add this transaction to the backstack fragmentTransaction.commit();
7. Handling Fragment Lifecycles
Understanding the fragment lifecycle is essential to manage UI state and resources efficiently. Properly handling lifecycle events can prevent crashes and memory leaks.
8. Fragment Best Practices
- Keep fragments independent and modular.
- Use interfaces for fragment communication.
- Optimize fragment transactions and backstack usage.
- Handle configuration changes effectively.
Conclusion
In this blog, we explored the world of Android Fragments, understanding their importance and benefits. We learned how to create fragments, implement communication between fragments and activities, and manage fragment lifecycles. By leveraging Android Fragments, you can build more adaptable and reusable UI components, leading to a smoother and more delightful user experience in your Android applications. Happy coding!
Table of Contents
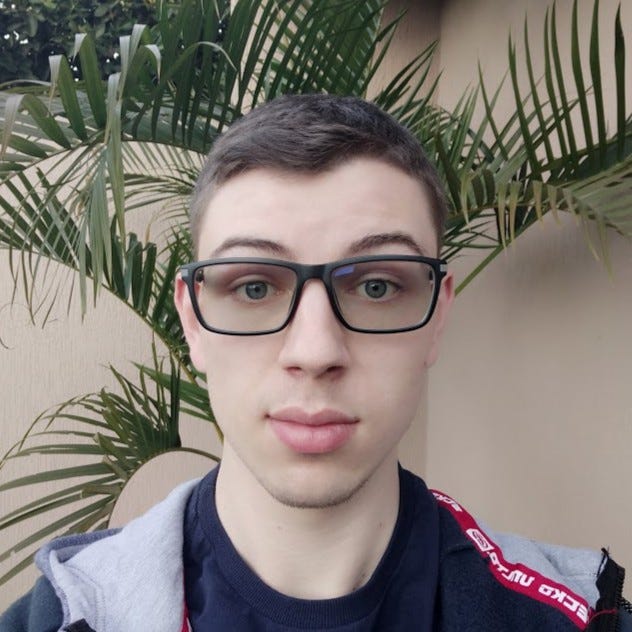
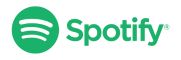