Android Sensors: Transforming the Way You Develop Apps
Modern Android devices are equipped with a multitude of sensors that can provide data about various aspects of the device’s environment and user activities. From accelerometers and gyroscopes to temperature sensors and barometers, the wealth of data available from these sensors presents a unique opportunity for app developers, including those looking to hire Android developers. These professionals can use this data to create more engaging, context-aware apps that adapt to the user’s environment and behavior. This blog post will explore the different types of sensors available on Android devices, shedding light on why hiring skilled Android developers is crucial. We’ll provide examples of how these sensors can be leveraged in app development to truly unlock a device’s potential.
Table of Contents
1. Understanding Android Sensors
Android sensors can be categorized into three types:
- Motion Sensors: These sensors measure acceleration forces and rotational forces along three axes. This category includes accelerometers, gravity sensors, gyroscopes, and rotational vector sensors.
- Environmental Sensors: These sensors measure various environmental parameters such as ambient air temperature, pressure, humidity, and illuminance. This category includes barometers, thermometers, and humidity sensors.
- Position Sensors: These sensors measure the physical position of a device. This category includes orientation sensors and magnetometers.
Every Android device supports a different set of sensors. To see the list of sensors supported by a device, you can use the `getSensorList()` method of the `SensorManager` class, which returns a list of `Sensor` objects representing all the sensors available on the device.
2. Using Android Sensors in Your Apps
Now, let’s look at some practical examples of how you can harness the power of Android sensors in your apps.
Example 1: Detecting Device Shakes with the Accelerometer
The accelerometer measures the acceleration force in m/s² that is applied to a device on all three physical axes (x, y, and z), including the force of gravity. It is one of the most commonly used sensors in mobile devices and has various applications, including shake detection.
To use the accelerometer in an Android app, you will need to do the following steps:
- Get a Reference to the Sensor Service
The first step is to get a reference to the system’s sensor service, which you can do using the `getSystemService()` method:
```java SensorManager sensorManager = (SensorManager) getSystemService(Context.SENSOR_SERVICE); ```
- Get a Reference to the Accelerometer Sensor
Next, get a reference to the accelerometer sensor:
```java Sensor accelerometer = sensorManager.getDefaultSensor(Sensor.TYPE_ACCELEROMETER); ```
- Register a Sensor Event Listener
The next step is to register a sensor event listener for the accelerometer sensor. The sensor event listener is an interface for receiving notifications from the SensorManager when sensor values have changed:
```java sensorManager.registerListener(this, accelerometer, SensorManager.SENSOR_DELAY_NORMAL); ```
- Implement the `onSensorChanged()` Method
Finally, implement the `onSensorChanged()` method of the SensorEventListener interface to detect shakes:
```java @Override public void onSensorChanged(SensorEvent event) { // Shake detection logic goes here } ```
This method is called whenever the sensor’s data changes, and it receives a SensorEvent object, which contains the new sensor data.
Example 2: Creating a Compass App with the Magnetometer and Accelerometer
A magnetometer measures the Earth’s magnetic field, and in conjunction with an accelerometer, it can be used to create a compass that displays the device’s current orientation. Here are the steps to do this:
- Get References to the Sensor Service, the Magnetometer, and the Accelerometer
```java SensorManager sensorManager = (SensorManager) getSystemService(Context.SENSOR_SERVICE); Sensor accelerometer = sensorManager.getDefaultSensor(Sensor.TYPE_ACCELEROMETER); Sensor magnetometer = sensorManager.getDefaultSensor(Sensor.TYPE_MAGNETIC_FIELD); ```
- Register Sensor Event Listeners
```java sensorManager.registerListener(this, accelerometer, SensorManager.SENSOR_DELAY_NORMAL); sensorManager.registerListener(this, magnetometer, SensorManager.SENSOR_DELAY_NORMAL); ```
- Implement the `onSensorChanged()` Method
```java float[] accelData = null; float[] magData = null; @Override public void onSensorChanged(SensorEvent event) { if (event.sensor.getType() == Sensor.TYPE_ACCELEROMETER) accelData = event.values; if (event.sensor.getType() == Sensor.TYPE_MAGNETIC_FIELD) magData = event.values; if (accelData != null && magData != null) { float R[] = new float[9]; float I[] = new float[9]; boolean success = SensorManager.getRotationMatrix(R, I, accelData, magData); if (success) { float orientation[] = new float[3]; SensorManager.getOrientation(R, orientation); // orientation contains: azimuth, pitch and roll } } } ```
In this method, we first check the type of the sensor that triggered the event and save the sensor data accordingly. If we have data from both the accelerometer and the magnetometer, we calculate the rotation matrix and then get the device’s orientation from the rotation matrix. The orientation is an array that contains the azimuth (orientation around the z-axis), pitch (orientation around the x-axis), and roll (orientation around the y-axis).
Conclusion
These examples are just the beginning when it comes to harnessing the power of Android sensors. From health monitoring apps that use the heart rate sensor to gaming apps that use the gyroscope for motion control, there are countless possibilities for creating innovative and engaging apps. This is where the decision to hire Android developers with expertise in sensor technology can be pivotal. With the right understanding and creativity, these professionals can leverage these sensors to craft applications that truly stand out from the crowd, offering an unparalleled user experience.
Table of Contents
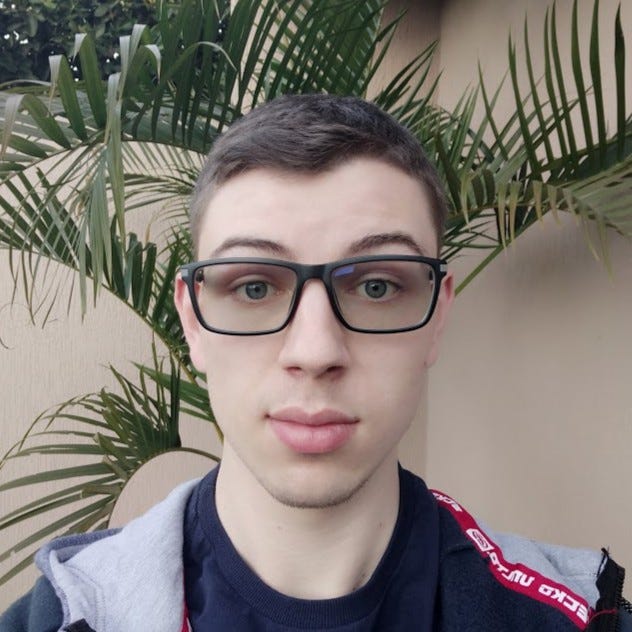
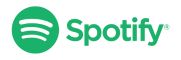