Give Your Android App a Voice with Speech Recognition
Voice technology has progressed by leaps and bounds in recent years. From voice-controlled assistants such as Google Assistant and Amazon Alexa to voice-operated apps, the demand for voice interaction is ever-growing. Android, being a front-runner in mobile technology, offers a powerful Speech Recognition API that developers can use to build voice-controlled interfaces. In this post, we’ll delve into Android’s Speech Recognition capabilities, providing examples and insights into how you can integrate this feature into your apps.
Table of Contents
1. What is Speech Recognition?
Speech Recognition, also referred to as Automatic Speech Recognition (ASR), involves the conversion of spoken language into text. It is the technology behind voice typing on a smartphone or voice commands on smart devices.
2. Android Speech Recognition API
Android’s Speech Recognition API is a part of the Android `Speech` package. It allows developers to incorporate speech-to-text capabilities in their applications without requiring internet connectivity. The Android platform offers the `RecognizerIntent` class, which communicates with the speech recognition service.
2.1 Setting up the Basics
- Permissions: Firstly, you need to declare permissions in your AndroidManifest.xml.
```xml <uses-permission android:name="android.permission.RECORD_AUDIO" /> ```
- Intent for Speech Recognition: Use the `RecognizerIntent` to invoke the speech recognition.
```java Intent intent = new Intent(RecognizerIntent.ACTION_RECOGNIZE_SPEECH); intent.putExtra(RecognizerIntent.EXTRA_LANGUAGE_MODEL, RecognizerIntent.LANGUAGE_MODEL_FREE_FORM); intent.putExtra(RecognizerIntent.EXTRA_PROMPT, "Speak now!"); startActivityForResult(intent, REQUEST_CODE); ```
- Retrieve Results: Handle the speech recognition results in `onActivityResult()`.
```java @Override protected void onActivityResult(int requestCode, int resultCode, Intent data) { super.onActivityResult(requestCode, resultCode, data); if (requestCode == REQUEST_CODE && resultCode == RESULT_OK) { ArrayList<String> matches = data.getStringArrayListExtra(RecognizerIntent.EXTRA_RESULTS); if (matches != null && matches.size() > 0) { String result = matches.get(0); // Use the result in your application } } } ```
3. Advanced Features and Tips
- Continuous Speech Recognition: Android doesn’t natively support continuous speech recognition in background services. But, third-party libraries like CMU Sphinx can help. It’s beneficial for applications that require ongoing listening.
- Multiple Language Support: The `RecognizerIntent` supports multiple languages. Specify the desired language using the `EXTRA_LANGUAGE` parameter.
```java intent.putExtra(RecognizerIntent.EXTRA_LANGUAGE, "es-ES"); // For Spanish ```
- Offline Recognition: While Android does support offline recognition, the user needs to download the necessary language packs from Settings.
4. Example: Building a Voice-controlled Note-taking App
Here’s a simple demonstration on how to build a voice-controlled note-taking app using the Android Speech Recognition API.
4.1. Setup Layout
Create a layout with a Button to start listening and a TextView to display the transcribed text.
```xml <Button android:id="@+id/btn_listen" android:text="Start Listening" android:layout_width="wrap_content" android:layout_height="wrap_content" /> <TextView android:id="@+id/tv_transcription" android:layout_width="match_parent" android:layout_height="wrap_content" /> ```
4.2. Handle Button Click
```java Button listenButton = findViewById(R.id.btn_listen); listenButton.setOnClickListener(v -> { Intent intent = new Intent(RecognizerIntent.ACTION_RECOGNIZE_SPEECH); intent.putExtra(RecognizerIntent.EXTRA_LANGUAGE_MODEL, RecognizerIntent.LANGUAGE_MODEL_FREE_FORM); intent.putExtra(RecognizerIntent.EXTRA_PROMPT, "Start speaking..."); startActivityForResult(intent, REQUEST_CODE); }); ```
4.3. Handle Results
```java @Override protected void onActivityResult(int requestCode, int resultCode, Intent data) { super.onActivityResult(requestCode, resultCode, data); if (requestCode == REQUEST_CODE && resultCode == RESULT_OK) { ArrayList<String> matches = data.getStringArrayListExtra(RecognizerIntent.EXTRA_RESULTS); if (matches != null && matches.size() > 0) { String transcription = matches.get(0); TextView transcriptionView = findViewById(R.id.tv_transcription); transcriptionView.setText(transcription); } } } ```
With these simple steps, you’ve created a basic voice-controlled note-taking app! From here, you can extend the functionalities such as saving notes, categorizing them, or even sharing them via different mediums.
Conclusion
The Android Speech Recognition API provides developers with a powerful tool to create voice-controlled interfaces, enriching the user experience. The opportunities are endless—from simple transcription services to advanced voice-controlled applications. With a touch of creativity, the sky is the limit when it comes to integrating voice commands into Android applications. Embrace the power of voice and craft exceptional user experiences!
Table of Contents
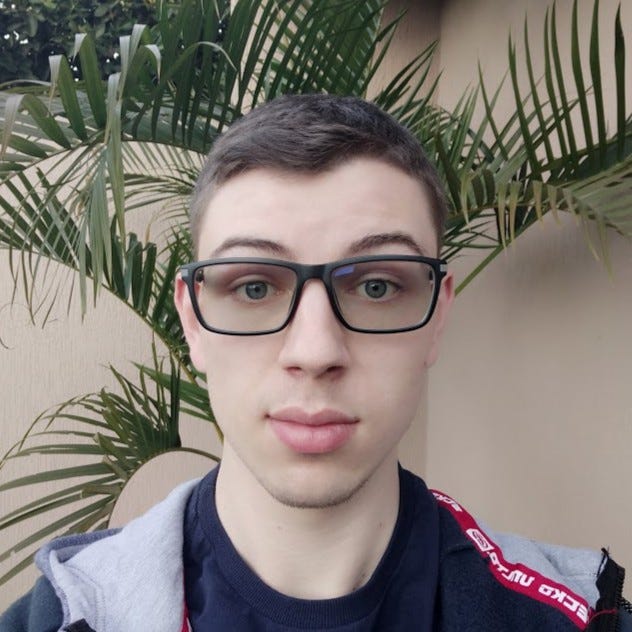
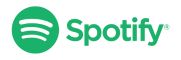