Using SQLite Database in Android: Performing CRUD Operations
When it comes to developing robust Android applications, data management is a critical aspect. Android provides developers with a powerful and lightweight relational database management system called SQLite. SQLite is a self-contained, serverless, and transactional database engine that is integrated with the Android framework. It offers a simple and efficient way to store, retrieve, update, and delete data in Android applications. In this blog, we will dive into the world of SQLite and explore how to perform CRUD (Create, Read, Update, Delete) operations using SQLite Database in Android.
1. What is SQLite Database?
SQLite is a software library that provides a relational database management system. It is embedded in the Android operating system and does not require any separate installation or configuration. SQLite stores data in a single file, making it easy to manage and transfer. It supports standard SQL syntax and offers various features such as transactions, indexes, and triggers.
2. Setting Up the Environment
Before we start working with SQLite Database in Android, we need to set up the development environment. Follow these steps:
Step 1: Open Android Studio and create a new Android project.
Step 2: Ensure that the necessary dependencies are added to your project’s build.gradle file. Include the following line in the dependencies section:
arduino implementation 'androidx.sqlite:sqlite:2.2.0'
Step 3: Sync the project with Gradle files to ensure the dependencies are properly downloaded and added to your project.
3. Creating a Database Helper Class
To interact with the SQLite Database in Android, we need to create a Database Helper class. This class will handle the creation, opening, and upgrading of the database. It will also provide methods for performing CRUD operations.
Create a new Java class called DatabaseHelper and extend it from SQLiteOpenHelper. Override the onCreate() and onUpgrade() methods as shown below:
java public class DatabaseHelper extends SQLiteOpenHelper { private static final String DATABASE_NAME = "mydatabase.db"; private static final int DATABASE_VERSION = 1; public DatabaseHelper(Context context) { super(context, DATABASE_NAME, null, DATABASE_VERSION); } @Override public void onCreate(SQLiteDatabase db) { // Create tables if they don't exist String createTableQuery = "CREATE TABLE IF NOT EXISTS mytable (id INTEGER PRIMARY KEY AUTOINCREMENT, name TEXT)"; db.execSQL(createTableQuery); } @Override public void onUpgrade(SQLiteDatabase db, int oldVersion, int newVersion) { // Upgrade the database if needed String upgradeTableQuery = "DROP TABLE IF EXISTS mytable"; db.execSQL(upgradeTableQuery); onCreate(db); } }
4. Creating a Table
Before performing CRUD operations, we need to create a table in the database. In the onCreate() method of the DatabaseHelper class, we have executed an SQL query to create a table called mytable with two columns: id and name.
5. Performing CRUD Operations
5.1 Inserting Data:
To insert data into the SQLite Database, we will create a method in the DatabaseHelper class. Add the following method to the DatabaseHelper class:
java public long insertData(String name) { SQLiteDatabase db = this.getWritableDatabase(); ContentValues values = new ContentValues(); values.put("name", name); long id = db.insert("mytable", null, values); db.close(); return id; }
To insert data, call this method and pass the name as a parameter. It will return the ID of the inserted row.
5.2 Reading Data:
To read data from the SQLite Database, create a method in the DatabaseHelper class. Add the following method:
java public List<String> getAllData() { List<String> data = new ArrayList<>(); SQLiteDatabase db = this.getReadableDatabase(); Cursor cursor = db.rawQuery("SELECT * FROM mytable", null); if (cursor.moveToFirst()) { do { String name = cursor.getString(cursor.getColumnIndex("name")); data.add(name); } while (cursor.moveToNext()); } cursor.close(); db.close(); return data; }
Call this method to retrieve all the data stored in the mytable table.
5.3 Updating Data:
To update data in the SQLite Database, create a method in the DatabaseHelper class. Add the following method:
java public int updateData(long id, String newName) { SQLiteDatabase db = this.getWritableDatabase(); ContentValues values = new ContentValues(); values.put("name", newName); int rowsAffected = db.update("mytable", values, "id=?", new String[]{String.valueOf(id)}); db.close(); return rowsAffected; }
Pass the ID of the row to be updated along with the new name as parameters to this method. It will return the number of rows affected.
5.4 Deleting Data:
To delete data from the SQLite Database, create a method in the DatabaseHelper class. Add the following method:
java public int deleteData(long id) { SQLiteDatabase db = this.getWritableDatabase(); int rowsDeleted = db.delete("mytable", "id=?", new String[]{String.valueOf(id)}); db.close(); return rowsDeleted; }
Pass the ID of the row to be deleted as a parameter to this method. It will return the number of rows deleted.
6. Closing the Database
It is important to close the database connection after performing CRUD operations. Open connections can lead to memory leaks and other issues. Call the close() method on the SQLiteDatabase instance after performing the necessary database operations.
7. Best Practices for Using SQLite Database in Android
- Use transactions when performing multiple database operations to improve performance and ensure data integrity.
- Always close the database connection after performing CRUD operations.
- Use proper error handling and exception catching to handle database-related exceptions.
- Implement proper data validation and sanitization to prevent SQL injection attacks.
- Consider using an ORM (Object-Relational Mapping) library like Room to simplify database operations and improve code maintainability.
Conclusion
In this blog, we have explored the usage of SQLite Database in Android for performing CRUD operations efficiently. We learned how to set up the development environment, create a database helper class, create a table, and perform CRUD operations such as inserting, reading, updating, and deleting data. By following the best practices, you can effectively manage data in your Android applications using SQLite Database.
Table of Contents
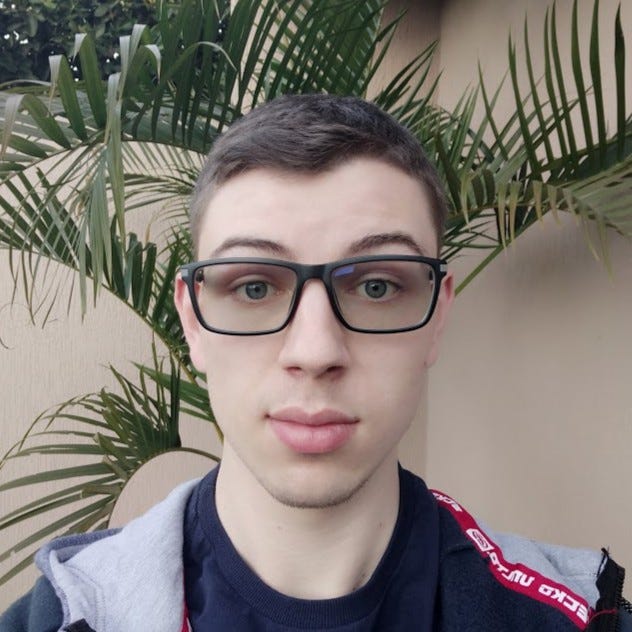
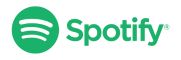