Redefining Data Handling: Advanced SQLite Techniques for Android Devs
SQLite is an embedded database library that provides a lightweight disk-based database. Android uses the SQLite database system, which allows developers to store structured data in a private database. While many developers are familiar with basic database operations such as `CREATE`, `INSERT`, `UPDATE`, and `DELETE`, this article delves into more advanced data manipulation techniques.
Table of Contents
1. Batch Insertion
The standard approach to insert data is to use a single `INSERT` statement. However, if you have a significant amount of data to insert, executing one statement at a time can be inefficient. Instead, batch insertion can be used.
```java SQLiteDatabase db = getWritableDatabase(); try { db.beginTransaction(); for (YourDataObject data : dataList) { ContentValues values = new ContentValues(); values.put("column1", data.value1); values.put("column2", data.value2); // ... add more values db.insert("YourTableName", null, values); } db.setTransactionSuccessful(); } finally { db.endTransaction(); } ```
2. Using `REPLACE`
SQLite offers the `REPLACE` command, which is a powerful tool. When you use `REPLACE`, if the row is new, it is inserted. If the row already exists (based on a UNIQUE constraint or PRIMARY KEY), it is deleted and then inserted.
```java ContentValues values = new ContentValues(); values.put("id", 1); values.put("name", "John"); values.put("age", 25); db.replace("YourTableName", null, values); ```
3. Using `CASE` with `UPDATE`
You can conditionally update data in your database by using the `CASE` statement in conjunction with `UPDATE`.
```java String query = "UPDATE YourTableName " + "SET age = CASE " + "WHEN id = 1 THEN 26 " + "WHEN id = 2 THEN 27 " + "ELSE age " + "END"; db.execSQL(query); ```
4. Database Views
A View is a virtual table that provides a different perspective on data from one or more tables. You can create a view from one or more base tables by tying them together using `JOIN` operations.
```java String createView = "CREATE VIEW IF NOT EXISTS user_with_address AS " + "SELECT u.name, u.age, a.street, a.city " + "FROM users u JOIN address a ON u.id = a.user_id"; db.execSQL(createView); ```
5. Using `GROUP BY` and `HAVING`
While `GROUP BY` groups rows that have the same values in specified columns, `HAVING` filters the results of a `GROUP BY`.
```java String query = "SELECT name, COUNT(*) " + "FROM users " + "GROUP BY name " + "HAVING COUNT(*) > 1"; Cursor cursor = db.rawQuery(query, null); ```
6. Database Triggers
Triggers are actions that are executed automatically when certain events occur. You can create a trigger to handle actions like updating a timestamp column whenever data in a particular row changes.
```java String createTrigger = "CREATE TRIGGER update_time_trigger " + "AFTER UPDATE ON YourTableName " + "BEGIN " + "UPDATE YourTableName SET last_modified = CURRENT_TIMESTAMP " + "WHERE id = old.id; " + "END;"; db.execSQL(createTrigger); ```
7. Compound `SELECT` operations
These are used to combine the result of two queries using union, union all, intersect, or except.
```java String query = "SELECT name FROM users WHERE age > 30 " + "UNION ALL " + "SELECT name FROM archived_users WHERE age > 30"; Cursor cursor = db.rawQuery(query, null); ```
8. Using the `ATTACH` command
If you’re working with multiple databases and you want to perform cross-database operations, `ATTACH` is the command you need.
```java String attachDb = "ATTACH DATABASE 'path_to_other_database' AS otherDb"; db.execSQL(attachDb); ```
Then, you can perform operations across databases:
```java String query = "INSERT INTO main.YourTableName SELECT * FROM otherDb.OtherTableName"; db.execSQL(query); ```
9. Pragmas
SQLite provides PRAGMA statements to modify the operational characteristics of the database or to query the database for internal (non-table) data.
Example: Checking the user_version:
```java Cursor cursor = db.rawQuery("PRAGMA user_version;", null); ```
10. Common Table Expressions (CTEs)
CTEs are temporary result sets that you can reference in a `SELECT`, `INSERT`, `UPDATE`, or `DELETE` statement.
```java String query = "WITH temp_users AS (SELECT * FROM users WHERE age > 30) " + "SELECT * FROM temp_users WHERE name LIKE 'J%'"; Cursor cursor = db.rawQuery(query, null); ```
Conclusion
Advanced techniques in SQLite can help developers handle data more effectively and efficiently, ensuring robust and responsive applications. By understanding and implementing these advanced methods, you can unlock the full potential of SQLite in your Android applications.
Whether you’re dealing with batch insertions, leveraging triggers, or exploring compound `SELECT` operations, mastering these techniques can give you an edge when developing sophisticated apps on the Android platform.
Table of Contents
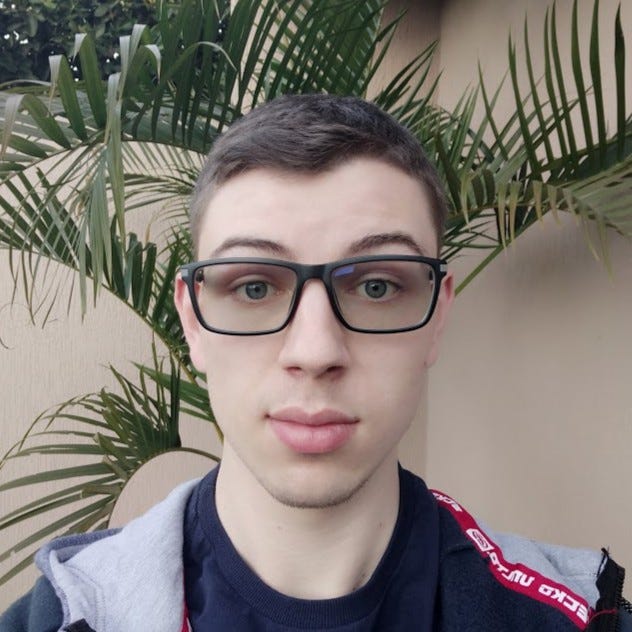
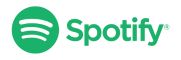