Android Data Persistence: Storing and Retrieving Data
Data persistence is a crucial aspect of modern Android application development. Whether it’s user preferences, cached data, or app-specific information, storing and retrieving data efficiently and securely is essential. In this blog post, we will explore various techniques for data persistence in Android, including shared preferences, file storage, and SQLite databases. By the end of this guide, you’ll have a solid understanding of different persistence options and be able to choose the right one for your application’s needs.
1. Shared Preferences: Storing Simple Data
1.1 Introduction to Shared Preferences:
Shared Preferences is a lightweight storage option provided by the Android framework for storing simple data types such as booleans, integers, floats, and strings. It offers a key-value pair-based storage system and is commonly used for storing user preferences.
1.2 Storing and Retrieving Data with Shared Preferences:
To store data using shared preferences, you need to obtain an instance of the SharedPreferences class using the getSharedPreferences() method. You can then use the editor() method to get an instance of the SharedPreferences.Editor class, which provides methods for adding key-value pairs to the preferences.
Code Sample:
java // Storing data in shared preferences SharedPreferences preferences = getSharedPreferences("MyPreferences", Context.MODE_PRIVATE); SharedPreferences.Editor editor = preferences.edit(); editor.putString("username", "JohnDoe"); editor.putInt("age", 25); editor.apply(); // Retrieving data from shared preferences String username = preferences.getString("username", ""); int age = preferences.getInt("age", 0);
1.3 Example: Saving and Retrieving User Preferences:
Let’s say we want to allow the user to save their name and email address in the app. We can use shared preferences to store this information.
Code Sample:
java // Saving user preferences SharedPreferences preferences = getSharedPreferences("UserPrefs", Context.MODE_PRIVATE); SharedPreferences.Editor editor = preferences.edit(); editor.putString("name", "John Doe"); editor.putString("email", "johndoe@example.com"); editor.apply(); // Retrieving user preferences String name = preferences.getString("name", ""); String email = preferences.getString("email", "");
2. File Storage: Storing Complex Data
2.1 Using Internal Storage:
Internal storage is a private storage space for an app to store files that are accessible only by the app. It is commonly used for storing sensitive data or files specific to the application.
To write data to internal storage, you can use the openFileOutput() method to get a FileOutputStream, and then write the data to the file using standard Java I/O operations.
Code Sample:
java // Writing data to a file in internal storage String filename = "mydata.txt"; String data = "Hello, World!"; try { FileOutputStream fos = openFileOutput(filename, Context.MODE_PRIVATE); fos.write(data.getBytes()); fos.close(); } catch (IOException e) { e.printStackTrace(); } // Reading data from a file in internal storage try { FileInputStream fis = openFileInput(filename); InputStreamReader isr = new InputStreamReader(fis); BufferedReader br = new BufferedReader(isr); StringBuilder sb = new StringBuilder(); String line; while ((line = br.readLine()) != null) { sb.append(line); } fis.close(); String fileData = sb.toString(); } catch (IOException e) { e.printStackTrace(); }
2.2 Using External Storage:
External storage provides a shared storage space that can be accessed by multiple applications. It is commonly used for storing large files, such as media files or downloaded content.
To write data to external storage, you need to request the WRITE_EXTERNAL_STORAGE permission in your app’s manifest file. You can then use standard Java I/O operations to write data to the desired location.
Code Sample:
java // Writing data to a file in external storage String filename = "mydata.txt"; String data = "Hello, World!"; File file = new File(Environment.getExternalStorageDirectory(), filename); try { FileWriter writer = new FileWriter(file); writer.write(data); writer.close(); } catch (IOException e) { e.printStackTrace(); } // Reading data from a file in external storage File file = new File(Environment.getExternalStorageDirectory(), filename); try { FileReader reader = new FileReader(file); BufferedReader br = new BufferedReader(reader); StringBuilder sb = new StringBuilder(); String line; while ((line = br.readLine()) != null) { sb.append(line); } reader.close(); String fileData = sb.toString(); } catch (IOException e) { e.printStackTrace(); }
3. SQLite Databases: Structured Data Storage
3.1 Introduction to SQLite Databases:
SQLite is a lightweight and embedded database engine that comes bundled with the Android framework. It provides a reliable and efficient way to store structured data in a relational database format.
3.2 Creating and Managing SQLite Databases:
To work with SQLite databases in Android, you’ll use the SQLiteOpenHelper class, which provides methods for creating, upgrading, and managing the database. You define your database schema using SQL statements and use the SQLiteDatabase class to perform CRUD (Create, Read, Update, Delete) operations.
Code Sample:
java // Creating a SQLite database public class MyDatabaseHelper extends SQLiteOpenHelper { private static final String DATABASE_NAME = "mydatabase.db"; private static final int DATABASE_VERSION = 1; public MyDatabaseHelper(Context context) { super(context, DATABASE_NAME, null, DATABASE_VERSION); } @Override public void onCreate(SQLiteDatabase db) { // Create tables and define schema here db.execSQL("CREATE TABLE users (id INTEGER PRIMARY KEY, name TEXT)"); } @Override public void onUpgrade(SQLiteDatabase db, int oldVersion, int newVersion) { // Upgrade database schema here } } // Performing CRUD operations MyDatabaseHelper dbHelper = new MyDatabaseHelper(context); SQLiteDatabase db = dbHelper.getWritableDatabase(); // Inserting data into a table ContentValues values = new ContentValues(); values.put("name", "John Doe"); long newRowId = db.insert("users", null, values); // Querying data from a table Cursor cursor = db.query("users", null, null, null, null, null, null); while (cursor.moveToNext()) { int id = cursor.getInt(cursor.getColumnIndex("id")); String name = cursor.getString(cursor.getColumnIndex("name")); } // Closing the database db.close();
3.3 Example: Storing and Retrieving Data from a SQLite Database:
Let’s say we want to create a simple app that allows the user to save and view a list of contacts. We can use a SQLite database to store the contact information.
Code Sample:
java // Creating a SQLite database for contacts public class ContactsDatabaseHelper extends SQLiteOpenHelper { private static final String DATABASE_NAME = "contacts.db"; private static final int DATABASE_VERSION = 1; public ContactsDatabaseHelper(Context context) { super(context, DATABASE_NAME, null, DATABASE_VERSION); } @Override public void onCreate(SQLiteDatabase db) { // Create contacts table db.execSQL("CREATE TABLE contacts (id INTEGER PRIMARY KEY, name TEXT, phone TEXT)"); } @Override public void onUpgrade(SQLiteDatabase db, int oldVersion, int newVersion) { // Upgrade database schema here } } // Storing a contact in the database ContactsDatabaseHelper dbHelper = new ContactsDatabaseHelper(context); SQLiteDatabase db = dbHelper.getWritableDatabase(); ContentValues values = new ContentValues(); values.put("name", "John Doe"); values.put("phone", "1234567890"); long newRowId = db.insert("contacts", null, values); // Retrieving contacts from the database Cursor cursor = db.query("contacts", null, null, null, null, null, null); while (cursor.moveToNext()) { int id = cursor.getInt(cursor.getColumnIndex("id")); String name = cursor.getString(cursor.getColumnIndex("name")); String phone = cursor.getString(cursor.getColumnIndex("phone")); } // Closing the database db.close();
4. Room Persistence Library: Simplifying SQLite Database Operations
4.1 Introduction to Room Persistence Library:
Room is a SQLite object mapping library provided by the Android Architecture Components. It simplifies the process of working with SQLite databases by providing an abstraction layer over raw SQL queries.
4.2 Setting Up Room in Your Project:
To use Room in your project, you need to include the necessary dependencies in your app’s build.gradle file. You define your database schema using annotated Java or Kotlin classes, and Room generates the necessary boilerplate code for you.
4.3 Example: Using Room to Store and Retrieve Data:
Let’s continue with our contacts app example and see how we can use Room to simplify the database operations.
Code Sample:
java // Define the entity class @Entity(tableName = "contacts") public class Contact { @PrimaryKey(autoGenerate = true) public int id; @ColumnInfo(name = "name") public String name; @ColumnInfo(name = "phone") public String phone; } // Define the DAO (Data Access Object) interface @Dao public interface ContactDao { @Insert void insert(Contact contact); @Query("SELECT * FROM contacts") List<Contact> getAllContacts(); } // Create the Room database @Database(entities = {Contact.class}, version = 1) public abstract class ContactsDatabase extends RoomDatabase { public abstract ContactDao contactDao(); } // Storing a contact using Room ContactsDatabase contactsDb = Room.databaseBuilder(context, ContactsDatabase.class, "contacts.db") .build(); ContactDao contactDao = contactsDb.contactDao(); Contact contact = new Contact(); contact.name = "John Doe"; contact.phone = "1234567890"; contactDao.insert(contact); // Retrieving contacts using Room List<Contact> contacts = contactDao.getAllContacts(); // Closing the database contactsDb.close();
5. Content Providers: Data Sharing and Access
5.1 Understanding Content Providers:
Content Providers are Android components that allow you to share data between different applications or access data from other applications. They provide a standardized way of exposing data and offer a high level of data security.
5.2 Creating a Content Provider:
To create a content provider, you need to define a subclass of the ContentProvider class and implement several methods, such as onCreate(), query(), insert(), update(), and delete(), to handle data operations.
5.3 Example: Using a Content Provider to Share Data:
Let’s say we want to create a content provider to share contact information with other applications. We can use a content provider to expose the contact data.
Code Sample:
java // Creating a content provider for contacts public class ContactsProvider extends ContentProvider { // Implement necessary methods here } // Registering the content provider in the manifest <provider android:name=".ContactsProvider" android:authorities="com.example.contactsprovider" android:exported="true" /> // Querying contacts using the content provider Uri uri = Uri.parse("content://com.example.contactsprovider/contacts"); Cursor cursor = getContentResolver().query(uri, null, null, null, null); while (cursor.moveToNext()) { String name = cursor.getString(cursor.getColumnIndex("name")); String phone = cursor.getString(cursor.getColumnIndex("phone")); }
6. Network Caching: Storing Data from Web Services
6.1 Caching Data for Offline Use:
Caching data from web services is a common requirement in Android applications. It allows users to access previously retrieved data when offline or to reduce network requests for frequently accessed data.
6.2 Example: Implementing Network Caching:
To implement network caching, you can use libraries like Retrofit or OkHttp, which provide built-in caching mechanisms. By configuring caching headers and specifying cache policies, you can control how data is stored and retrieved from the cache.
Code Sample (using OkHttp):
java // Creating an OkHttpClient with caching enabled Cache cache = new Cache(getCacheDir(), 10 * 1024 * 1024); // 10 MB cache size OkHttpClient client = new OkHttpClient.Builder() .cache(cache) .build(); // Creating a request with caching headers Request request = new Request.Builder() .url("https://api.example.com/data") .build(); // Making the request try { Response response = client.newCall(request).execute(); String responseData = response.body().string(); // Process the response data } catch (IOException e) { e.printStackTrace(); }
Conclusion
In this blog post, we explored various techniques for data persistence in Android applications. We covered shared preferences for storing simple data, file storage for complex data, SQLite databases for structured data storage, Room Persistence Library for simplifying database operations, content providers for data sharing and access, and network caching for storing data from web services. Understanding these persistence options will enable you to design robust and efficient data storage solutions for your Android applications.
Table of Contents
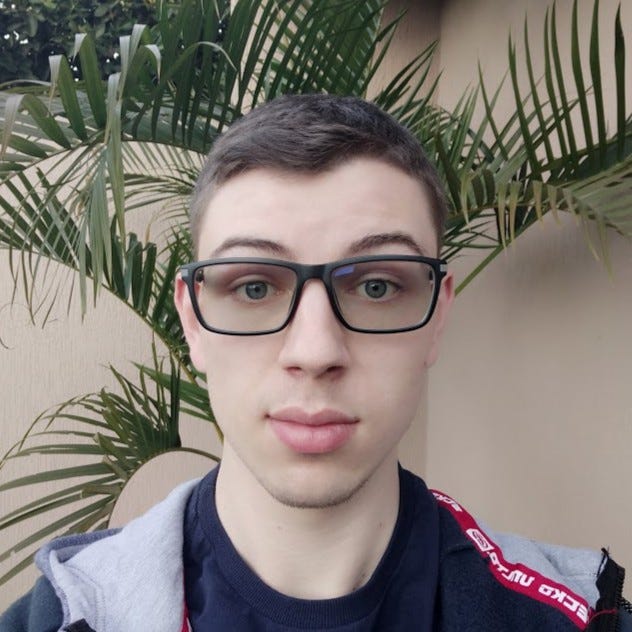
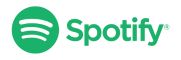