Mastering Android User Interface Design: Implementing Best Practices for Enhanced User Experiences
User interface design is a critical aspect of Android application development. It is the primary conduit for interaction between users and your app. Employing skilled Android developers can ensure the creation of a user-friendly, intuitive interface, significantly influencing your app’s success.
This blog post will outline the best practices that professional Android developers follow when building user interfaces in Android, providing practical examples to illuminate these concepts.
Understand the Basics
Understanding the basics of Android’s user interface components is the first step. Android provides a rich set of views and widgets (like buttons, text fields, checkboxes), layouts (like linear, relative, constraint), and compound views (like recycler views, card views) for building your app’s UI. Know how to use these components to create clean and easy-to-use interfaces.
Example: To use a button in your app, you should define it in your XML layout file.
```xml <Button android:id="@+id/button_submit" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Submit" />
And then reference it in your Java or Kotlin file:
```kotlin val button = findViewById<Button>(R.id.button_submit) button.setOnClickListener { // Your action here }
Use Proper Naming Conventions
Proper naming conventions make your code easier to read and maintain, which is a key reason to hire Android developers who understand this practice. Good Android developers recognize the importance of naming conventions in Android development and apply them consistently in their code. For instance, a Button for submission could be aptly named `button_submit`, indicating its function and type simultaneously. This clarity enhances code maintenance and readability, integral aspects of successful app development.
```xml <Button android:id="@+id/button_submit" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Submit" />
Follow Material Design Guidelines
Material Design is a design system developed by Google to create a visual language that synthesizes classic principles of good design with technology and science’s innovation. Following these guidelines will provide a consistent and delightful experience for your users.
Example: Using a Floating Action Button (FAB) for a primary action in your app.
```xml <com.google.android.material.floatingactionbutton.FloatingActionButton android:id="@+id/fab" android:layout_width="wrap_content" android:layout_height="wrap_content" android:contentDescription="Add Icon" app:srcCompat="@android:drawable/ic_input_add" />
Use ConstraintLayout
ConstraintLayout allows you to create large and complex layouts with a flat view hierarchy (no nested view groups). It’s a flexible layout manager for your app that allows you to position and size widgets in a free-form way.
Example: Centering a button on the screen using ConstraintLayout.
```xml <androidx.constraintlayout.widget.ConstraintLayout android:layout_width="match_parent" android:layout_height="match_parent"> <Button android:id="@+id/button_submit" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Submit" app:layout_constraintBottom_toBottomOf="parent" app:layout_constraintLeft_toLeftOf="parent" app:layout_constraintRight_toRightOf="parent" app:layout_constraintTop_toTopOf="parent" /> </androidx.constraintlayout.widget.ConstraintLayout>
Handle Different Screen Sizes
Remember, Android runs on a variety of devices that offer different screen sizes and densities. You need to ensure your UI is flexible and adapts to different screen sizes and orientations. Use `wrap_content`, `match_parent`, or weight in LinearLayout for views instead of hard-coded sizes. Use different layout folders like `layout-small`, `layout-large`, etc., for adapting to various screen sizes.
```xml <TextView android:layout_width="0dp" android:layout_height="wrap_content" android:layout_weight="1" android:text="This TextView fills the available space" />
Use Styles and Themes
Use styles and themes for a consistent design language throughout the app. Define common attributes like colors, fonts, and button styles in a base style, and apply the base style to your app theme.
```xml <!-- Base application theme --> <style name="AppTheme" parent="Theme.AppCompat.Light.DarkActionBar"> <!-- Customize your theme here --> <item name="colorPrimary">@color/colorPrimary</item> <item name="colorPrimaryDark">@color/colorPrimaryDark</item> <item name="colorAccent">@color/colorAccent</item> </style>
Design for Accessibility
Ensure your app is usable by as many people as possible by making it accessible. Provide `contentDescription` for images and icons, ensure sufficient color contrast, and ensure all interactive elements are large enough to be easily tapped.
```xml <ImageView android:layout_width="wrap_content" android:layout_height="wrap_content" android:src="@drawable/ic_launcher" android:contentDescription="@string/launcher_icon" />
Test Your UI
Finally, don’t forget to test your user interface. Use Android’s Espresso UI testing framework to simulate user interactions and verify that the UI reacts correctly.
```kotlin @Test fun buttonClick_GoesToNewActivity() { // Click on the button onView(withId(R.id.button_submit)).perform(click()) // Check if the new activity is displayed onView(withId(R.id.new_activity_layout)).check(matches(isDisplayed())) }
Conclusion
Building user interfaces in Android requires a deep understanding of various components and design principles, a skill set that professional Android developers possess. By following best practices, or choosing to hire Android developers who are adept in these, you can create user interfaces that are not only visually appealing but also highly functional and intuitive. This results in a superior user experience, enhancing the overall impact and effectiveness of your app.
Table of Contents
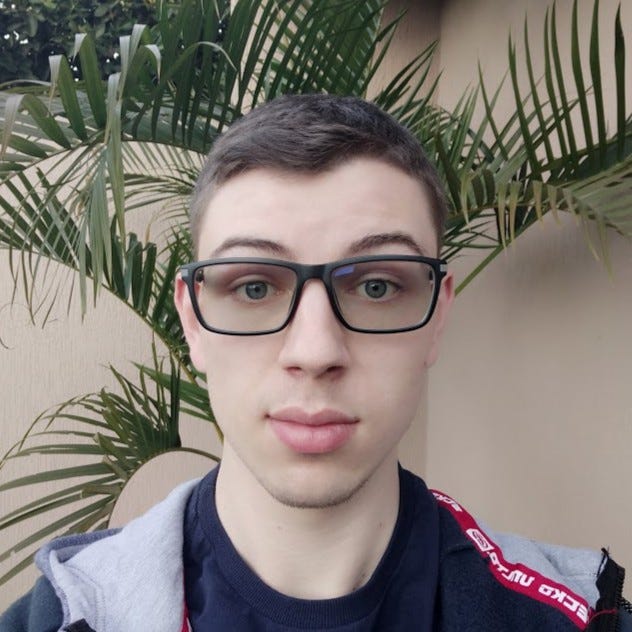
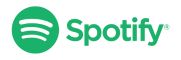