Android Q & A
How do I use LiveData in Android?
Using LiveData in Android empowers developers to build reactive and lifecycle-aware applications that seamlessly propagate data changes to UI components. Here’s a comprehensive guide on how to harness the power of LiveData in your Android app:
- Add LiveData Dependency: Begin by adding the LiveData dependency to your app’s build.gradle file.
groovy
implementation "androidx.lifecycle:lifecycle-livedata-ktx:2.4.0"
- Create LiveData Objects: Define LiveData objects to hold your data within your ViewModel or other data sources. LiveData can encapsulate various types of data, such as strings, integers, lists, or custom objects.
kotlin
val myLiveData: LiveData<String> = MutableLiveData()
- Set Data: Populate LiveData objects with initial data values. You can update LiveData objects by setting their values using setValue() or postValue() methods.
kotlin
(myLiveData as MutableLiveData).value = "Hello, LiveData!"
- Observe LiveData: In your UI components, observe LiveData objects to receive updates whenever the data changes. Use the observe() method to register observers and define actions to take when the data changes.
kotlin
myViewModel.myLiveData.observe(viewLifecycleOwner) { newData -> // Update UI with new data textView.text = newData }
- Handle Lifecycle Awareness: LiveData automatically handles lifecycle events of its associated lifecycle owner (e.g., activity or fragment). Observers are automatically removed when the lifecycle owner is destroyed, preventing memory leaks and crashes.
- Transform LiveData: Use LiveData transformation methods to transform and combine LiveData sources into new LiveData objects. This enables powerful data manipulation and composition operations.
kotlin
val transformedLiveData = Transformations.map(myLiveData) { originalData -> // Transform original data here transformedData }
- Threading Considerations: LiveData objects emit data on the main thread by default. To perform background operations, use coroutines or LiveData’s built-in support for background threading.
By following these steps, you can effectively use LiveData to create responsive, lifecycle-aware, and reactive Android applications. LiveData simplifies data management and propagation, enhances UI responsiveness, and promotes a more maintainable and scalable app architecture.
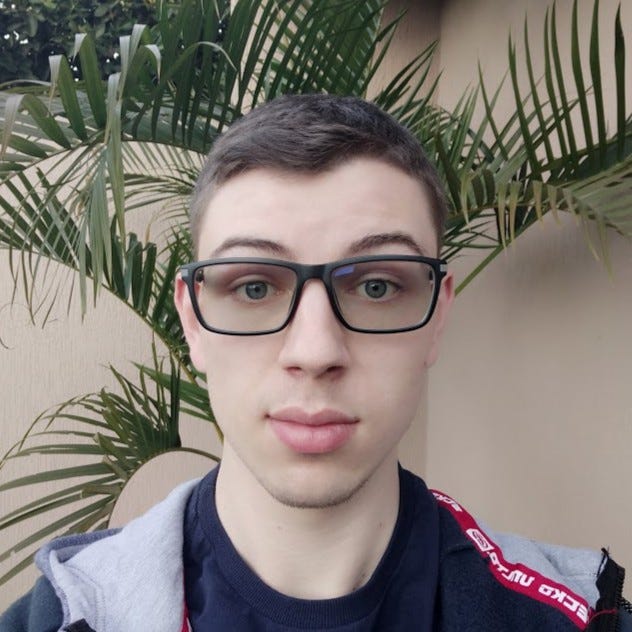
Previously at
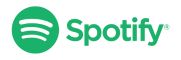
Skilled Android Engineer with 5 years of expertise in app development, ad formats, and enhancing user experiences across high-impact projects