How do I use Room Persistence Library in Android?
The Room Persistence Library in Android provides an abstraction layer over SQLite databases, making it easier for developers to perform database operations and manage local data storage in their Android applications. Here’s a comprehensive guide on how to use the Room Persistence Library in your Android app:
Add Room Dependency: Start by adding the Room Persistence Library dependency to your app’s build.gradle file. Ensure that you’re using a compatible version of the Room library that matches your project’s AndroidX dependencies.
Define Entity Classes: Define the data model for your database by creating entity classes that represent the tables and columns in your SQLite database. Annotate each entity class with @Entity to specify its structure and properties. Use annotations such as @PrimaryKey, @ColumnInfo, and @ForeignKey to define primary keys, column names, and relationships between entities.
Create Data Access Objects (DAOs): Define Data Access Objects (DAOs) to define the methods for accessing and manipulating data in your database. Annotate each DAO interface with @Dao and declare methods for performing CRUD (Create, Read, Update, Delete) operations on your entities. Use SQL-like query annotations such as @Query, @Insert, @Update, and @Delete to define custom queries and operations.
Create Database Class: Create a database class that extends RoomDatabase and serves as the main entry point for interacting with your SQLite database. Annotate the database class with @Database and specify the list of entity classes and DAO interfaces that belong to the database. Implement the database singleton pattern to ensure that only one instance of the database is created and used throughout your app.
Instantiate Database Object: Obtain an instance of your database object using the Room.databaseBuilder() method or the Room.inMemoryDatabaseBuilder() method. Customize the database configuration as needed, such as specifying the database name, migration strategy, and other options.
Perform Database Operations: Use the DAO methods to perform database operations such as inserting, querying, updating, and deleting data. Invoke the DAO methods asynchronously using Kotlin coroutines, RxJava, or Android’s built-in AsyncTask to ensure that database operations don’t block the main UI thread and maintain smooth app performance.
Handle Database Transactions: Use database transactions to ensure data consistency and integrity when performing multiple database operations as a single unit of work. Encapsulate related database operations within a transaction block using the @Transaction annotation to execute them atomically and rollback changes if an error occurs.
Handle LiveData and Observables: Room integrates seamlessly with LiveData and RxJava to provide real-time updates and observe changes in your database data. Use LiveData or RxJava observables to observe database queries and receive automatic updates whenever the underlying data changes, enabling reactive UI updates and efficient data synchronization.
By following these steps, you can effectively use the Room Persistence Library to implement local data storage and database operations in your Android app, making it easier to manage and interact with data while maintaining data integrity and performance. Always refer to the latest Room documentation and best practices for guidance on using Room in your Android app development.
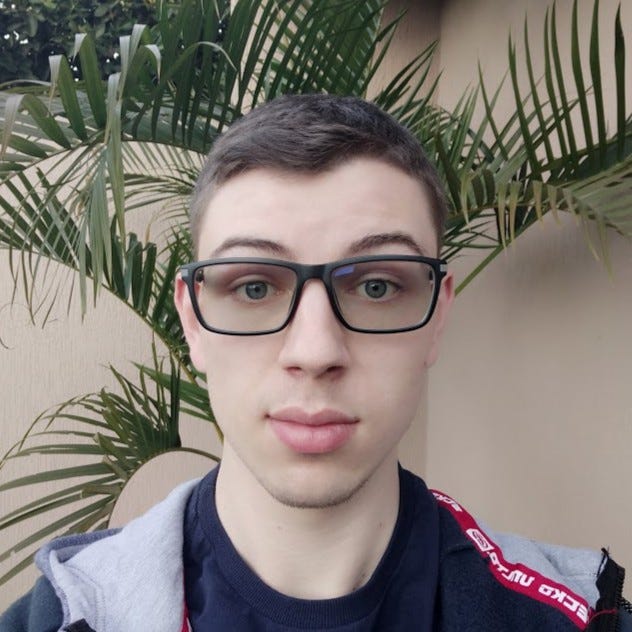
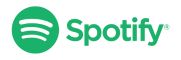