Wrist-Ready: A Guide to Extending App Alerts to Android Wear Devices
The world is moving towards a more connected future, with smartphones, smartwatches, and other wearable devices becoming integral parts of our daily lives. Among the leading platforms for wearable tech is Android Wear. Android Wear, commonly known for powering smartwatches, provides a fantastic platform for developers to extend their apps and provide users with notifications right on their wrists.
Table of Contents
In this article, we’ll explore how to effectively extend your Android app’s notifications to smartwatches, making the most out of Android Wear’s features. Let’s get started!
1. Why Android Wear Notifications?
Before diving into the code, let’s discuss the importance of wearable notifications:
- Immediacy: Checking a wristwatch is faster and less intrusive than pulling out a phone.
- Context-aware: Smartwatches can provide context-based notifications (like workout stats during a run) more effectively than phones.
- Enhanced User Engagement: Users are more likely to engage with timely, relevant notifications delivered to their wrists.
2. Basic Notification
If you’ve ever created a notification for an Android app, you’d be pleased to know that those notifications will automatically appear on an Android Wear device paired with the phone. Here’s a simple example:
```java NotificationCompat.Builder builder = new NotificationCompat.Builder(this, CHANNEL_ID) .setSmallIcon(R.drawable.notification_icon) .setContentTitle("My notification") .setContentText("Hello from Android Wear!") .setPriority(NotificationCompat.PRIORITY_DEFAULT); NotificationManagerCompat notificationManager = NotificationManagerCompat.from(this); notificationManager.notify(NOTIFICATION_ID, builder.build()); ```
Once executed, this notification will appear both on the user’s phone and their paired Android Wear device.
3. Adding Actions
Android Wear supports action buttons, just like on phones. However, on a smartwatch, it’s often more relevant due to the ease of access. Here’s how you can add an action:
```java Intent actionIntent = new Intent(this, ActionResponseActivity.class); PendingIntent actionPendingIntent = PendingIntent.getActivity(this, 0, actionIntent, 0); NotificationCompat.Builder builder = new NotificationCompat.Builder(this, CHANNEL_ID) .setSmallIcon(R.drawable.notification_icon) .setContentTitle("Meeting Reminder") .setContentText("Meet with Sarah in 10 minutes.") .addAction(R.drawable.ic_reply, "Reply", actionPendingIntent); NotificationManagerCompat notificationManager = NotificationManagerCompat.from(this); notificationManager.notify(NOTIFICATION_ID, builder.build()); ```
4. Stacking Notifications
In case you have multiple notifications, Android Wear provides a stacking feature to group similar ones. It’s particularly useful to prevent overwhelming users:
```java String GROUP_KEY_WORK_EMAIL = "com.example.android.wearable.notification.KEY_WORK_EMAIL"; // First notification NotificationCompat.Builder builder1 = new NotificationCompat.Builder(this, CHANNEL_ID) .setSmallIcon(R.drawable.notification_icon) .setContentTitle("New Work Email from Alice") .setContentText("The project update...") .setGroup(GROUP_KEY_WORK_EMAIL); // Second notification NotificationCompat.Builder builder2 = new NotificationCompat.Builder(this, CHANNEL_ID) .setSmallIcon(R.drawable.notification_icon) .setContentTitle("New Work Email from Bob") .setContentText("Regarding the proposal...") .setGroup(GROUP_KEY_WORK_EMAIL); // Group notification Notification summaryNotification = new NotificationCompat.Builder(this, CHANNEL_ID) .setSmallIcon(R.drawable.notification_icon) .setStyle(new NotificationCompat.InboxStyle() .addLine("Alice: The project update...") .addLine("Bob: Regarding the proposal...") .setBigContentTitle("2 Work Emails") .setSummaryText("work@example.com")) .setGroup(GROUP_KEY_WORK_EMAIL) .setGroupSummary(true) .build(); NotificationManagerCompat notificationManager = NotificationManagerCompat.from(this); notificationManager.notify(emailNotificationId1, builder1.build()); notificationManager.notify(emailNotificationId2, builder2.build()); notificationManager.notify(SUMMARY_ID, summaryNotification); ```
5. Pages in Notifications
You can add extra pages to your Android Wear notification, which users can swipe to view. This is useful when there’s more information than the main notification should display:
```java // Main notification NotificationCompat.Builder mainNotification = new NotificationCompat.Builder(this, CHANNEL_ID) .setSmallIcon(R.drawable.notification_icon) .setContentTitle("Chat with Alice") .setContentText("How's the project going?"); // Page notification NotificationCompat.Builder secondPageNotification = new NotificationCompat.Builder(this) .setContentTitle("Earlier messages") .setContentText("Yesterday: Discussed the timeline. Last week: Defined objectives."); // Combine notifications Notification notification = mainNotification .extend(new NotificationCompat.WearableExtender().addPage(secondPageNotification.build())) .build(); NotificationManagerCompat notificationManager = NotificationManagerCompat.from(this); notificationManager.notify(NOTIFICATION_ID, notification); ```
6. Voice Input
Given the form factor, voice input is critical for wearables. Android Wear supports voice replies for notifications:
```java String replyLabel = getResources().getString(R.string.reply_label); String[] replyChoices = getResources().getStringArray(R.array.reply_choices); RemoteInput remoteInput = new RemoteInput.Builder(EXTRA_VOICE_REPLY) .setLabel(replyLabel) .setChoices(replyChoices) .build(); NotificationCompat.Action action = new NotificationCompat.Action.Builder(R.drawable.ic_reply, "Reply", actionPendingIntent) .addRemoteInput(remoteInput) .build(); NotificationCompat.Builder builder = new NotificationCompat.Builder(this, CHANNEL_ID) .setSmallIcon(R.drawable.notification_icon) .setContentTitle("Chat with Alice") .setContentText("How's the project going?") .addAction(action); NotificationManagerCompat notificationManager = NotificationManagerCompat.from(this); notificationManager.notify(NOTIFICATION_ID, builder.build()); ```
Conclusion
Android Wear offers a rich ecosystem for extending app notifications to smartwatches. With thoughtful design and context-driven interactions, developers can enhance user engagement and provide a seamless experience across devices. Dive in, and experiment to unlock the full potential of wearable notifications!
Table of Contents
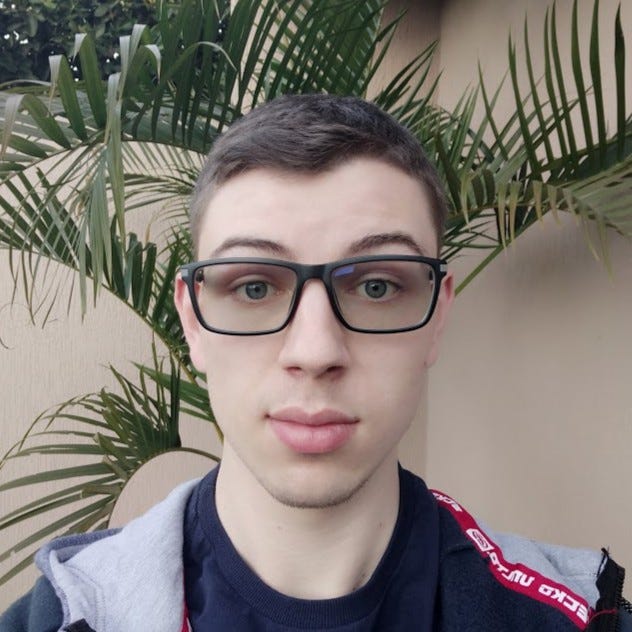
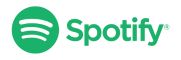