Android Wearables: Developing Apps for Smartwatches
The world of technology is rapidly evolving, and with it, we see an increasing demand for more portable and user-friendly devices. Smartwatches have emerged as a significant player in the wearable tech market, offering users a convenient way to access essential information on the go. As the popularity of smartwatches grows, so does the need for developers to create engaging and functional apps for these devices.
Table of Contents
In this blog post, we’ll explore the world of Android Wearables and guide you through the process of developing apps for smartwatches. Whether you’re a seasoned Android developer or just getting started with app development, this comprehensive guide will provide you with the necessary knowledge to build exciting and innovative wearable experiences.
1. Understanding Android Wearables:
Before we dive into the development process, let’s gain a solid understanding of Android Wearables. Android Wear is a version of the Android operating system specifically designed for smartwatches and other wearable devices. It provides a set of unique features and design guidelines that developers should adhere to while creating apps for smartwatches.
2. Features of Android Wearables:
Android Wearables come with a range of features that make them stand out from traditional Android devices. Some of the key features include:
- Notifications: Smartwatches excel at delivering timely notifications from various apps, providing users with quick access to crucial information without the need to take out their smartphones.
- Voice Commands: Android Wearables support voice commands, enabling users to interact with apps using voice input. This feature enhances the hands-free experience and allows for a more natural way of interacting with smartwatches.
- Custom Watch Faces: Developers can create custom watch faces to give users the ability to personalize the look and feel of their smartwatches. This allows for greater user engagement and uniqueness in the wearable experience.
- Sensors Integration: Smartwatches come equipped with various sensors like heart rate monitors, accelerometers, and GPS. Developers can leverage these sensors to build health and fitness apps, navigation tools, and more.
3. Getting Started with Android Wearable Development:
To begin developing apps for Android Wearables, you’ll need to set up your development environment. Here’s a step-by-step guide to getting started:
- Install Android Studio: Android Studio is the official Integrated Development Environment (IDE) for Android app development. Install Android Studio and set up the necessary SDKs, including the Android Wear SDK.
- Create a Wearable Project: In Android Studio, create a new Wearable project. This will generate the basic structure for your wearable app and ensure that it’s compatible with Android Wearables.
- Emulate an Android Wear Device: Android Studio provides an emulator that allows you to test your app on virtual Android Wear devices. Use this emulator to preview and debug your app.
4. Designing Apps for Android Wearables:
Designing for smartwatches requires a different approach compared to designing for smartphones or tablets. Here are some essential design guidelines to keep in mind:
- Simplified User Interface: Smartwatch screens are significantly smaller, so the user interface should be clean, uncluttered, and easy to navigate. Use concise text and simple icons to convey information.
- Gesture-based Navigation: Smartwatches often rely on gestures for navigation, such as swiping and tapping. Incorporate gesture-based interactions to make your app more intuitive.
- Card-based Layouts: Android Wear apps use a card-based layout, where each piece of information or functionality is presented as a separate card. Utilize this design pattern for a consistent and user-friendly experience.
- Contextual Information: Leverage the power of context by delivering relevant information based on the user’s location, preferences, and previous interactions.
5. Building an Android Wearable App: Step-by-Step Tutorial:
Now that we have a good understanding of Android Wearables and the design principles, let’s dive into the process of building a simple wearable app. In this example, we’ll create a basic weather app that displays the current temperature and weather conditions.
Step 1: Project Setup and Configuration
First, create a new Wearable project in Android Studio. Ensure that you’ve included the necessary permissions for accessing location and internet.
gradle // app/build.gradle android { ... defaultConfig { ... minSdkVersion 25 targetSdkVersion 30 ... } ... } dependencies { ... implementation 'androidx.wear:wear:1.1.0' implementation 'com.google.android.gms:play-services-wearable:17.0.0' ... }
Step 2: Designing the User Interface
Create the layout for your weather app, keeping in mind the design guidelines mentioned earlier. For this example, we’ll use a simple card-based layout.
xml <!-- res/layout/activity_weather.xml --> <androidx.wear.widget.BoxInsetLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" android:layout_width="match_parent" android:layout_height="match_parent"> <androidx.wear.widget.CircularProgressLayout android:id="@+id/progressLayout" android:layout_width="match_parent" android:layout_height="match_parent" android:layout_gravity="center"> <androidx.wear.widget.CircularProgress android:id="@+id/progress" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_gravity="center" app:circle_color="@color/colorAccent" app:circle_radius="48dp" app:circle_stroke_width="6dp" /> <TextView android:id="@+id/tvTemperature" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_gravity="center" android:text="Loading..." android:textSize="18sp" /> </androidx.wear.widget.CircularProgressLayout> </androidx.wear.widget.BoxInsetLayout>
Step 3: Fetching Weather Data
Now, let’s fetch the weather data from a weather API. For simplicity, we’ll use OpenWeatherMap API.
java // WeatherService.java import retrofit2.Call; import retrofit2.http.GET; import retrofit2.http.Query; public interface WeatherService { @GET("weather") Call<WeatherResponse> getWeather(@Query("q") String city, @Query("appid") String apiKey); } java // WeatherResponse.java import com.google.gson.annotations.SerializedName; public class WeatherResponse { @SerializedName("main") private Main main; @SerializedName("weather") private Weather[] weather; public Main getMain() { return main; } public Weather[] getWeather() { return weather; } }
Step 4: Displaying Weather Data
Update the main activity to fetch weather data and display it on the smartwatch screen.
java // MainActivity.java import android.os.Bundle; import android.support.wearable.activity.WearableActivity; import android.widget.TextView; public class MainActivity extends WearableActivity { private TextView tvTemperature; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_weather); tvTemperature = findViewById(R.id.tvTemperature); // TODO: Fetch weather data using Retrofit and display it on the screen } }
6. Testing and Deployment:
Testing your Android Wearable app is crucial to ensure it performs as expected on different smartwatch models. Use the Android Emulator or test the app on actual Android Wear devices to identify and fix any issues.
Once you’re satisfied with your app’s performance, it’s time to deploy it to the Google Play Store. Ensure that your app meets all the necessary requirements and guidelines set by Google before submitting it for review.
Conclusion
Developing apps for Android Wearables presents an exciting opportunity for developers to explore the world of wearable technology. By understanding the unique features and design principles of smartwatches, you can create engaging and user-friendly apps that enhance the overall wearable experience.
In this blog post, we covered the basics of Android Wearable development, from setting up the development environment to building a simple weather app. Now it’s your turn to dive deeper into the world of Android Wearables and create innovative apps that cater to the growing audience of smartwatch users.
Remember to stay updated with the latest trends in wearable technology, as the field is constantly evolving. Happy coding and best of luck with your Android Wearable app development journey!
Table of Contents
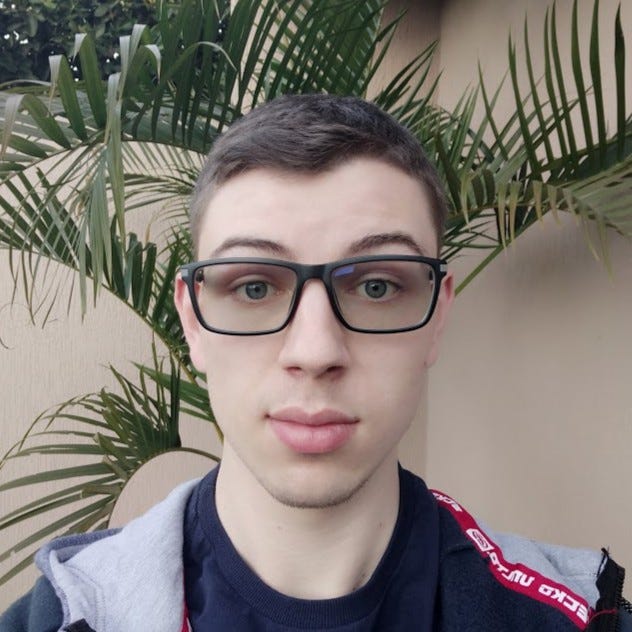
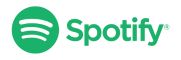