Web Meets Android: Dive Deep into WebView for a Superior User Experience
WebViews are one of the quintessential components of Android applications. They allow developers to display web pages directly within their Android apps, creating a seamless browsing experience without requiring users to switch to a browser app. For businesses looking to leverage this functionality, it might be an opportune moment to hire Android developers.
Table of Contents
This opens up a whole range of opportunities, from embedding specific web content to building hybrid apps that combine the power of web and native UI components. Hiring skilled Android developers can further enhance these integrations, offering a richer user experience.
In this article, we’ll dive deep into Android WebView, offering hands-on examples to give you a comprehensive understanding.
1. What is WebView?
At its core, a WebView provides an embedded view that displays web content within an Android app. Behind the scenes, it uses the WebKit rendering engine to process web content.
2. Setting Up WebView
Before we dive into code, you need to ensure that your app has permission to access the internet.
Add this line to your `AndroidManifest.xml`:
```xml <uses-permission android:name="android.permission.INTERNET" /> ```
Now, let’s move on to implementing the WebView.
2.1 Basic Implementation
- Layout File:
In your `activity_main.xml` (or other relevant layout file), add the WebView:
```xml <WebView android:id="@+id/webview" android:layout_width="match_parent" android:layout_height="match_parent" /> ```
- Java/Kotlin Code:
In your `MainActivity`, instantiate and load a URL:
```java WebView myWebView = (WebView) findViewById(R.id.webview); myWebView.loadUrl("https://www.example.com"); ```
2.2 Enabling JavaScript
By default, JavaScript is disabled in WebViews. If the content you plan to display relies on JavaScript, you’ll need to enable it:
```java WebSettings webSettings = myWebView.getSettings(); webSettings.setJavaScriptEnabled(true); ```
3. Handling Navigation
Allowing the user to navigate web content within your WebView is essential for an integrated experience.
3.1 WebViewClient
The key to this is setting a `WebViewClient`. This tells your WebView to handle URL loads internally rather than opening them in an external browser.
```java myWebView.setWebViewClient(new WebViewClient()); ```
Now, when a user clicks a link, it will load within the WebView itself.
4. Advanced WebView Features
4.1. Loading Local Content
Instead of a remote URL, you can also load HTML content stored within your app:
```java String customHtml = "<html><body><h1>Hello, WebView</h1></body></html>"; myWebView.loadData(customHtml, "text/html", "UTF-8"); ```
4.2 Handling JavaScript Alerts
To make sure JavaScript alerts show up, override the `onJsAlert` method:
```java myWebView.setWebChromeClient(new WebChromeClient() { @Override public boolean onJsAlert(WebView view, String url, String message, JsResult result) { // Handle the alert here return super.onJsAlert(view, url, message, result); } }); ```
4.3 JavaScript to Android Communication
Bridging JavaScript and Android allows for dynamic interactions. Here’s a simple example:
In Java:
```java class WebAppInterface { @JavascriptInterface public void showToast(String toast) { Toast.makeText(MainActivity.this, toast, Toast.LENGTH_SHORT).show(); } } myWebView.addJavascriptInterface(new WebAppInterface(), "Android"); ```
In your web content’s JavaScript:
```javascript Android.showToast('Hello from WebView!'); ```
4.4 Handling Page Loading Progress
Display a progress bar to inform users about page loading status:
```java myWebView.setWebChromeClient(new WebChromeClient() { @Override public void onProgressChanged(WebView view, int newProgress) { if (newProgress == 100) { // Page loading completed } else { // Update the progress bar with `newProgress` value } } }); ```
5. Caveats and Security
While WebViews are powerful, they come with potential pitfalls:
- Avoid XSS: Always validate the content being loaded to prevent Cross-Site Scripting attacks.
- Limit JavaScript Interfaces: Only expose necessary Android methods to your WebView to minimize risks.
- Handle SSL Errors: Override the `onReceivedSslError` method of `WebViewClient` to manage SSL errors appropriately.
- Keep WebView Updated: Ensure your users are on devices with the latest WebView updates to benefit from security patches.
Conclusion
Android WebView is a flexible component that bridges the world of web and native Android applications. When aiming to maximize its potential, many businesses opt to hire Android developers to ensure a polished implementation. When used correctly, it can enhance the user experience by integrating web content smoothly into your app.
Remember, though, with great power comes great responsibility. Alongside the decision to hire Android developers, always be mindful of the security implications when working with web content, ensuring you provide the best and safest user experience possible.
Table of Contents
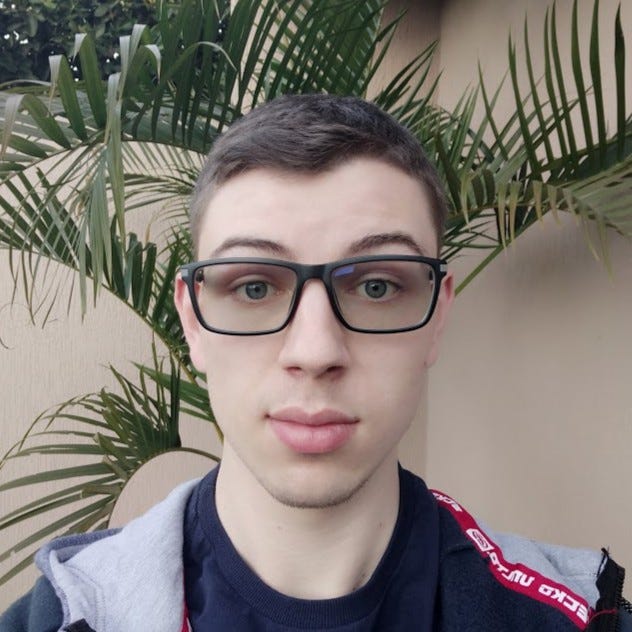
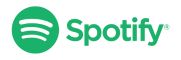