Animating Your Angular Web Applications: A Practical Guide to Angular Animations
When it comes to building dynamic, interactive web applications, one of the key elements often overlooked by developers is animation. However, expert Angular developers recognize the potential of using animations to provide visual feedback, guide tasks, and make your application more interactive and fun. Angular, a widely-used platform for building web applications, comes equipped with powerful tools for implementing complex animations. Thus, when you hire Angular developers, you tap into their expertise of using such tools to their full potential. Today, we will dive deep into the world of Angular animations, demonstrating how Angular developers animate your applications to make them more engaging and user-friendly.
What is Angular Animation?
Angular’s animation system lets developers build animations that live within their web applications in a declarative manner. It uses the Web Animations API to power its animations and provides a DSL (Domain Specific Language) that’s easy to use, yet powerful enough to build complex animations.
Getting Started with Angular Animations
Before diving into animations, ensure that you have an Angular project set up. If you don’t, you can create a new one using the Angular CLI:
```bash ng new my-animation-project ``` Then, navigate into the newly created project: ```bash cd my-animation-project ```
To use Angular animations, you must import the `BrowserAnimationsModule` from the `@angular/platform-browser/animations` package and add it to your app’s `NgModule` imports array:
```typescript import { BrowserAnimationsModule } from '@angular/platform-browser/animations'; @NgModule({ ... imports: [ ... BrowserAnimationsModule, ], ... }) export class AppModule { } ```
This module provides the necessary infrastructure for defining and managing animations in Angular.
Basic Animation: Fade In and Fade Out
To start, we’ll begin with one of the most common animations you’ll see on the web – fading in and fading out. First, let’s import the necessary functions from `@angular/animations`:
```typescript import { trigger, transition, style, animate } from '@angular/animations'; ```
These functions will allow us to define and control our animation. Next, we add the animation metadata to our component. This metadata will define the animations:
```typescript @Component({ ... animations: [ trigger('fadeInOut', [ transition(':enter', [ style({ opacity: 0 }), animate('500ms', style({ opacity: 1 })) ]), transition(':leave', [ animate('500ms', style({ opacity: 0 })) ]) ]) ], ... }) export class MyComponent { } ```
In the code above, `:enter` refers to when the element is added to the view, and `:leave` refers to when it is removed from the view. The `style` function is used to define the styles at various stages of the animation, and the `animate` function describes how the transition will take place.
Now, we can attach this animation to any HTML element within the component’s template:
```html <div [@fadeInOut]> I will fade in when created and fade out when destroyed. </div> ```
The `@` symbol denotes an animation trigger, followed by the name of the trigger.
Complex Animation: Slide In and Slide Out
Now, let’s make things a little more complex with a slide in and slide out animation. This will involve changing both the `opacity` and `transform` properties:
```typescript @Component({ ... animations: [ trigger('slideInOut', [ transition(':enter', [ style({ transform: 'translateX(-100%)', opacity: 0 }), animate('500ms ease-in', style({ transform: 'translateX(0)', opacity: 1 })) ]), transition(':leave', [ animate('500ms ease-in', style({ transform: 'translateX(-100%)', opacity: 0 })) ]) ]) ], ... }) export class MyComponent { } ```
In the above code, `translateX(-100%)` moves the element completely off the screen to the left. The `animate` function then gradually changes the `transform` property to `translateX(0)`, which brings the element back to its original position, while also increasing the `opacity` from 0 to 1.
This animation can also be applied in the HTML template just like the previous example:
```html <div [@slideInOut]> I will slide in from the left when created and slide out to the left when destroyed. </div> ```
Grouping and Sequencing Animations
In some scenarios, you might need to perform multiple animations simultaneously or in a specific sequence. Angular provides the `group` and `sequence` functions to accomplish this:
```typescript import { group, sequence, query, animateChild } from '@angular/animations'; @Component({ ... animations: [ trigger('parent', [ transition(':enter', [ group([ query('@child', animateChild()), sequence([ style({ opacity: 0 }), animate('1s', style({ opacity: 1 })), ]) ]) ]), transition(':leave', [ sequence([ animate('1s', style({ opacity: 0 })), query('@child', animateChild()) ]) ]) ]), trigger('child', [ transition(':enter', [ style({ opacity: 0 }), animate('500ms', style({ opacity: 1 })) ]), transition(':leave', [ animate('500ms', style({ opacity: 0 })) ]) ]) ], ... }) export class MyComponent { } ```
In the above code, the `parent` animation contains a `group` which means the animations inside will run in parallel. The `query` function with `animateChild()` will start the `child` animation. The `sequence` function ensures that the animations inside it will run in the specified order. So, the `parent` fades in at the same time as the `child`, but it continues to fade in for an additional half a second after the `child` has fully appeared. On leave, the `parent` begins fading out immediately, and halfway through its fade-out, the `child` begins to fade out.
Conclusion
Animation can enhance the user experience of a web application by providing visual feedback and making interactions more engaging. The Angular animation system is a powerful tool that, once mastered, can greatly enhance your Angular applications. This is where hiring Angular developers can be a game-changer for your projects, as they are well-versed in using these powerful tools.
Angular provides simple, declarative APIs for defining complex animations and allows for fine-grained control over animation sequencing and timing. Whether you’re fading elements in and out, sliding menus into view, or orchestrating complex multi-element animations, Angular animations have you covered. When you hire Angular developers, they bring their expertise in harnessing these features to your project, creating web applications that are not only functional but also interactive and visually appealing.
As with anything, practice is the key to mastering Angular animations. Use these examples as a starting point and experiment with the different functions and options available. Before you know it, you’ll be bringing your Angular applications to life with complex, eye-catching animations.
Table of Contents
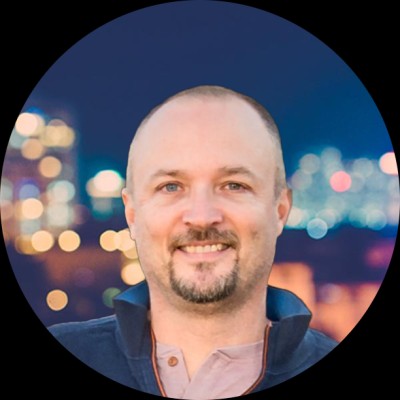
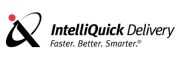