Angular and Authentication: Implementing User Login and Registration
In the ever-evolving landscape of web development, authentication remains a crucial aspect of building secure and user-friendly applications. Angular, being one of the most popular front-end frameworks, offers robust tools and techniques for implementing user login and registration functionalities seamlessly. In this guide, we’ll delve into the process of integrating authentication features into your Angular applications, covering everything from setting up authentication services to implementing user interfaces for login and registration.
1. Setting Up Authentication Services
Before diving into the implementation details, it’s essential to set up authentication services within your Angular application. Authentication services handle tasks such as user authentication, token management, and API communication. For this purpose, Angular provides the HttpClient module for making HTTP requests to backend servers securely.
```typescript // auth.service.ts import { Injectable } from '@angular/core'; import { HttpClient } from '@angular/common/http'; import { Observable } from 'rxjs'; @Injectable({ providedIn: 'root' }) export class AuthService { private baseUrl = 'https://your-backend.com/api/auth'; constructor(private http: HttpClient) { } login(credentials: any): Observable<any> { return this.http.post(`${this.baseUrl}/login`, credentials); } register(user: any): Observable<any> { return this.http.post(`${this.baseUrl}/register`, user); } // Additional methods for token management and user authentication } ```
2. Implementing User Interfaces
With the authentication services in place, let’s proceed to implement the user interfaces for login and registration forms. Angular’s built-in forms module provides powerful tools for creating reactive forms with validation capabilities.
```html <!-- login.component.html --> <form [formGroup]="loginForm" (ngSubmit)="onSubmit()"> <input type="email" formControlName="email" placeholder="Email"> <input type="password" formControlName="password" placeholder="Password"> <button type="submit">Login</button> </form> ``` ```typescript // login.component.ts import { Component, OnInit } from '@angular/core'; import { FormBuilder, FormGroup, Validators } from '@angular/forms'; import { AuthService } from '../auth.service'; @Component({ selector: 'app-login', templateUrl: './login.component.html', styleUrls: ['./login.component.css'] }) export class LoginComponent implements OnInit { loginForm: FormGroup; constructor(private formBuilder: FormBuilder, private authService: AuthService) { } ngOnInit(): void { this.loginForm = this.formBuilder.group({ email: ['', [Validators.required, Validators.email]], password: ['', [Validators.required]] }); } onSubmit(): void { if (this.loginForm.valid) { this.authService.login(this.loginForm.value).subscribe(response => { // Handle successful login }, error => { // Handle login error }); } } } ```
Conclusion
Implementing user login and registration in Angular applications is a fundamental yet crucial task for ensuring security and user engagement. By following the steps outlined in this guide, you can seamlessly integrate authentication features into your Angular projects, providing users with a seamless and secure experience.
External Resources:
- Angular Documentation – https://angular.io/guide/forms
- JWT Authentication in Angular – https://www.digitalocean.com/community/tutorials/angular-authentication-using-jwt
- Angular Firebase Authentication – https://angularfirebase.com/lessons/google-user-auth-with-firestore-custom-data/
Table of Contents
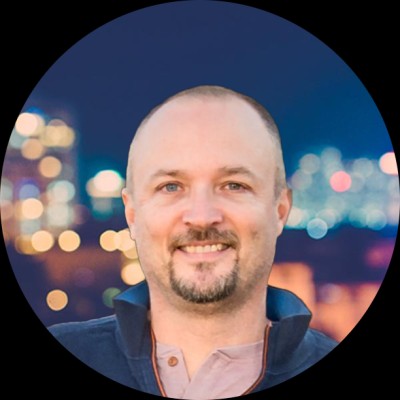
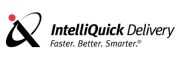