Angular and Authentication with OAuth 2.0: Secure User Access
In modern web applications, securing user access is a top priority. OAuth 2.0, a widely adopted authorization framework, provides a robust method for authenticating users. Angular, with its powerful framework, is well-suited to integrate OAuth 2.0, allowing developers to implement secure user authentication with ease. This article explores how to use OAuth 2.0 in Angular, providing practical examples and best practices for secure authentication.
Understanding OAuth 2.0
OAuth 2.0 is an industry-standard protocol for authorization, allowing applications to securely access resources on behalf of a user. It decouples authentication from authorization, enabling third-party applications to access user data without exposing their credentials.
Key Concepts of OAuth 2.0:
- Resource Owner: The user who owns the data.
- Client: The application requesting access to the resource.
- Authorization Server: The server that issues access tokens.
- Resource Server: The server that hosts the protected resources.
Using Angular for OAuth 2.0 Authentication
Angular, with its modular architecture and comprehensive support for HTTP communications, is ideal for implementing OAuth 2.0. Below are key steps and code examples demonstrating how to integrate OAuth 2.0 with an Angular application.
1. Setting Up OAuth 2.0 with Angular
The first step in integrating OAuth 2.0 is to configure the Angular application to interact with the OAuth 2.0 provider. For this example, we’ll use the popular `angular-oauth2-oidc` library, which simplifies OAuth 2.0 implementation in Angular.
Installation:
```bash npm install angular-oauth2-oidc --save ```
Example: Configuring OAuth 2.0 in Angular
```typescript import { OAuthService, AuthConfig } from 'angular-oauth2-oidc'; export const authConfig: AuthConfig = { issuer: 'https://example-auth.com', redirectUri: window.location.origin, clientId: 'your-client-id', responseType: 'code', scope: 'openid profile email', showDebugInformation: true, }; export class AuthConfigModule { constructor(private oauthService: OAuthService) { this.oauthService.configure(authConfig); this.oauthService.loadDiscoveryDocumentAndTryLogin(); } } ```
In this example, the `AuthConfig` object defines the necessary configurations, including the issuer URL, client ID, and redirect URI. The `loadDiscoveryDocumentAndTryLogin()` method loads the authorization server’s configuration and attempts to log in the user.
2. Implementing Login and Logout
After setting up OAuth 2.0, you need to implement login and logout functionality. This involves redirecting users to the OAuth 2.0 provider for authentication and handling the returned access token.
Example: Login and Logout Methods
```typescript import { Component } from '@angular/core'; import { OAuthService } from 'angular-oauth2-oidc'; @Component({ selector: 'app-auth', template: ` <button (click)="login()">Login</button> <button (click)="logout()">Logout</button> `, }) export class AuthComponent { constructor(private oauthService: OAuthService) {} login() { this.oauthService.initCodeFlow(); } logout() { this.oauthService.logOut(); } } ```
Here, the `login()` method initiates the OAuth 2.0 authorization code flow, redirecting the user to the login page. The `logout()` method clears the access token and ends the user session.
3. Securing Routes with OAuth 2.0
To secure specific routes in your Angular application, you can use route guards that check whether the user is authenticated before allowing access.
Example: Implementing an Auth Guard
```typescript import { Injectable } from '@angular/core'; import { CanActivate, Router } from '@angular/router'; import { OAuthService } from 'angular-oauth2-oidc'; @Injectable({ providedIn: 'root', }) export class AuthGuard implements CanActivate { constructor(private oauthService: OAuthService, private router: Router) {} canActivate(): boolean { if (this.oauthService.hasValidAccessToken()) { return true; } else { this.router.navigate(['/login']); return false; } } } ```
The `AuthGuard` class checks whether a valid access token exists before allowing access to protected routes. If the token is invalid or absent, the user is redirected to the login page.
4. Handling Access Tokens
Once authenticated, the access token received from the OAuth 2.0 provider can be used to access protected resources. Angular’s HTTP client can include this token in the headers of API requests.
Example: Attaching the Access Token to HTTP Requests
```typescript import { HttpClient, HttpHeaders } from '@angular/common/http'; import { OAuthService } from 'angular-oauth2-oidc'; @Injectable({ providedIn: 'root', }) export class ApiService { constructor(private http: HttpClient, private oauthService: OAuthService) {} getProtectedResource() { const headers = new HttpHeaders().set( 'Authorization', 'Bearer ' + this.oauthService.getAccessToken() ); return this.http.get('https://api.example.com/protected', { headers }); } } ```
In this example, the `Authorization` header is set with the access token, allowing the API request to authenticate the user.
Conclusion
Integrating OAuth 2.0 with Angular provides a secure and scalable method for managing user authentication. By leveraging Angular’s robust framework and the `angular-oauth2-oidc` library, developers can implement secure authentication flows, protect routes, and manage access tokens effectively. This approach enhances the security of your Angular applications and provides users with a seamless and secure login experience.
Further Reading:
Table of Contents
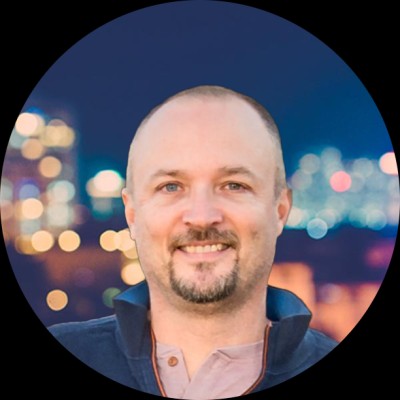
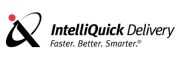