Angular Best Practices: Writing Clean and Maintainable Code
Angular, a robust framework for building dynamic web applications, empowers developers to create scalable and maintainable codebases. However, without following best practices, Angular projects can quickly become cluttered and difficult to manage. In this guide, we’ll explore key strategies for writing clean and maintainable Angular code, backed by real-world examples.
1. Consistent Folder Structure
Maintaining a consistent folder structure is crucial for keeping your Angular project organized and easily navigable. By adhering to a standard structure, team members can quickly locate files and understand the project’s architecture. Here’s an example folder structure:
``` - src - app - components - services - models - modules - utils - shared - assets - environments ```
2. Modularize Components
Angular’s component-based architecture promotes reusability and separation of concerns. Break down your application into smaller, reusable components that focus on specific functionalities. For instance, a user authentication module should contain components related to login, registration, and password recovery. Here’s an example of a modularized component structure:
``` - components - auth - login - register - forgot-password - dashboard - sidebar - header - widgets ```
3. Utilize Angular Services
Services in Angular are singletons that provide shared functionality across components. Utilize services to encapsulate business logic, data fetching, and state management. By centralizing these responsibilities, you can prevent code duplication and promote maintainability. Here’s an example of a user service:
```typescript @Injectable({ providedIn: 'root' }) export class UserService { private users: User[] = []; constructor(private http: HttpClient) {} getUsers(): Observable<User[]> { return this.http.get<User[]>('/api/users'); } addUser(user: User): Observable<User> { return this.http.post<User>('/api/users', user); } deleteUser(id: number): Observable<void> { return this.http.delete<void>(`/api/users/${id}`); } } ```
4. Implement Lazy Loading
In large Angular applications, lazy loading helps improve performance by loading modules only when they are needed. Split your application into feature modules and lazy load them using Angular’s routing capabilities. This reduces initial bundle size and speeds up the application’s startup time. Here’s an example of lazy loading in Angular routing:
```typescript const routes: Routes = [ { path: 'dashboard', loadChildren: () => import('./dashboard/dashboard.module').then(m => m.DashboardModule) }, { path: 'admin', loadChildren: () => import('./admin/admin.module').then(m => m.AdminModule) }, // Other routes... ]; ```
5. Follow Angular Style Guide
Adhering to the official Angular Style Guide ensures consistency and readability across your codebase. Follow naming conventions, use descriptive variable names, and employ consistent formatting. By adopting a unified coding style, developers can collaborate more effectively and maintain code with ease.
Conclusion
Writing clean and maintainable Angular code is essential for the long-term success of your project. By following best practices such as maintaining a consistent folder structure, modularizing components, utilizing Angular services, implementing lazy loading, and adhering to the Angular Style Guide, you can build scalable applications that are easy to maintain and extend.
External Resources:
- Angular Style Guide – https://angular.io/guide/styleguide
- Angular Lazy Loading – https://angular.io/guide/router#lazy-loading
- Angular Services – https://angular.io/guide/architecture-services
Table of Contents
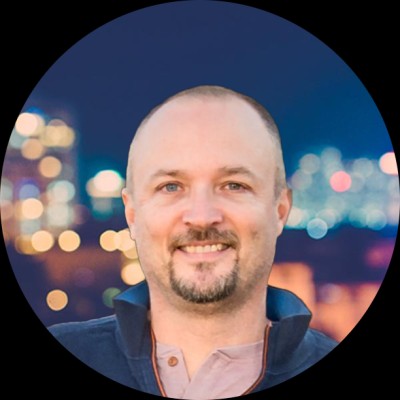
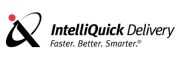