Unraveling the Power of Angular: From Basic Components to Interactive Web Applications
The Angular framework, developed by Google, has gained significant popularity among web developers for creating dynamic, modern, and efficient single-page applications (SPAs). It’s no surprise then that businesses are increasingly looking to hire Angular developers to capitalize on this power. At the heart of Angular’s functionality are its components, which act as the building blocks of these applications.
In this blog post, we’ll explore Angular components, understand how they contribute to building dynamic user interfaces, and dive into examples that will guide you effectively. Whether you’re a seasoned developer or a business looking to hire Angular developers, this knowledge can prove incredibly valuable.
Through this exploration, you will be better equipped to leverage Angular’s strengths. It’s the very reason why companies hire Angular developers – to unlock the potential of dynamic user interfaces in their web applications. By the end of this post, you’ll not only grasp the fundamentals but also appreciate why it’s a smart business move to hire Angular developers.
1. Angular Components Overview
In Angular, a component is a self-contained unit that encapsulates a template (the view), class (the business logic), and metadata defined via a decorator, which allows you to configure the component’s behavior.
Components promote a modular structure, making your codebase more maintainable, scalable, and testable. The core idea behind using components is to divide a large application into several small, manageable, reusable, and interconnected components.
2. Creating a Basic Angular Component
Let’s begin our exploration by creating a basic Angular component. Here, we will create a simple “GreetingComponent” that displays a greeting message.
```typescript import { Component } from '@angular/core'; @Component({ selector: 'app-greeting', template: `<h1>{{message}}</h1>`, }) export class GreetingComponent { message: string = 'Hello, Angular!'; } ```
This component consists of:
- An import statement to bring in the `Component` decorator from the ‘@angular/core’ module.
- The `@Component` decorator, where we define metadata like the component’s selector (`app-greeting`) and template.
- The class `GreetingComponent` where we define the properties and methods that control the behavior of the view.
You can now use this component in any part of your application by adding the custom tag `<app-greeting></app-greeting>`.
3. Building Dynamic User Interfaces
The true power of Angular components lies in their ability to create dynamic user interfaces. Components can interact with each other, exchange data, and react to external changes, making your application interactive and responsive.
Let’s explore some concepts that can make your Angular components more dynamic.
3.1. Interpolation and Property Binding
Interpolation and property binding are two ways by which data gets transferred from the TypeScript code to the template.
Interpolation uses double curly braces (`{{ }}`) to display data. In our `GreetingComponent`, we used interpolation to display the `message`.
Property binding, on the other hand, is a one-way data binding technique that helps us bind values to DOM properties of HTML elements. Let’s consider a `UserComponent` where we want to bind an `id` property to the id of a `div`:
```typescript import { Component } from '@angular/core'; @Component({ selector: 'app-user', template: `<div [id]="userId">Hello User!</div>`, }) export class UserComponent { userId: string = 'user123'; } ```
The `[id]=”userId”` syntax is property binding, which assigns the `userId` value to the `div` id.
3.2. Event Binding
Event binding allows us to respond to user actions, such as clicks, key presses, and mouse movements. Let’s add a button to our `UserComponent` that updates the user ID when clicked:
```typescript import { Component } from '@angular/core'; @Component({ selector: 'app-user', template: ` <div [id]="userId">Hello User!</div> <button (click)="updateUserId()">Update ID</button> `, }) export class UserComponent { userId: string = 'user123'; updateUserId(): void { this.userId = 'user' + Math.floor(Math .random() * 1000); } } ```
Here, `(click)=”updateUserId()”` is event binding. It calls the `updateUserId` method when the button is clicked.
3.3. Two-Way Binding
Two-way binding is a feature that allows changes in the model to update the view and vice versa. This is useful when you want an input field in your template to update a property in your component, and any changes to that property should reflect back in the view.
Let’s extend our `UserComponent` to include two-way data binding:
```typescript import { Component } from '@angular/core'; @Component({ selector: 'app-user', template: ` <div [id]="userId">Hello, {{userId}}!</div> <input [(ngModel)]="userId"> <button (click)="updateUserId()">Update ID</button> `, }) export class UserComponent { userId: string = 'user123'; updateUserId(): void { this.userId = 'user' + Math.floor(Math.random() * 1000); } } ```
The `[(ngModel)]=”userId”` syntax represents two-way binding. It binds the `input` field and the `userId` property such that changes in one reflect in the other.
3.4. Component Interaction
Components can interact with each other to exchange data. This can be achieved through `@Input()` and `@Output()` decorators for parent-to-child and child-to-parent communication, respectively.
Let’s create two components: `ParentComponent` and `ChildComponent`. We will pass data from the parent to the child and send an event from the child to the parent:
```typescript // child.component.ts import { Component, Input, Output, EventEmitter } from '@angular/core'; @Component({ selector: 'app-child', template: ` <h2>{{ parentData }}</h2> <button (click)="notifyParent()">Notify Parent</button> `, }) export class ChildComponent { @Input() parentData: string; @Output() notify: EventEmitter<string> = new EventEmitter<string>(); notifyParent(): void { this.notify.emit('Message from Child Component'); } } // parent.component.ts import { Component } from '@angular/core'; @Component({ selector: 'app-parent', template: ` <app-child [parentData]="message" (notify)="receiveNotification($event)"></app-child> `, }) export class ParentComponent { message: string = 'Hello from Parent Component'; receiveNotification(notification: string): void { console.log(notification); } } ```
In the `ChildComponent`, we declare an `@Input()` property to receive data from the parent and an `@Output()` property to emit events to the parent. This type of interaction is a fundamental aspect of Angular development and one of the reasons businesses opt to hire Angular developers. In the `ParentComponent`, we bind to these properties to send data and receive notifications, showing the high level of interactivity possible when you have the expertise of Angular developers on your team. Understanding these concepts is crucial, whether you’re an aspiring developer or a business looking to hire Angular developers to strengthen your web applications.
Conclusion
Angular components are the core building blocks of Angular applications. They enable the construction of dynamic, interactive, and modular user interfaces. By comprehending the concepts of data binding, event handling, and component interaction, you can harness the power of Angular to create feature-rich and reactive web applications. This is why many companies decide to hire Angular developers who can efficiently use these features.
The examples covered in this post serve as a solid foundation for building dynamic user interfaces with Angular. However, the potential of Angular is vast, and there is still much more to explore. This is a key reason why businesses hire Angular developers – to tap into this vast potential. Keep practicing and exploring more complex concepts such as routing, dependency injection, services, directives, and more to fully leverage the capabilities of this robust framework. As you expand your skill set, you’ll find that you become an even more valuable asset for companies looking to hire Angular developers.
Table of Contents
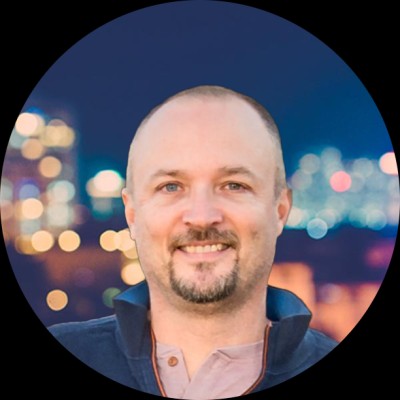
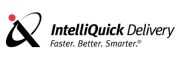