Angular Debugging: Tools and Techniques for Troubleshooting
Introduction to Angular Debugging
Debugging is a crucial part of software development, especially when working with complex frameworks like Angular. Effective debugging not only helps identify and fix issues but also enhances code quality and performance. This article explores essential tools and techniques for debugging Angular applications, providing practical examples to help you troubleshoot more efficiently.
Common Angular Debugging Tools
Angular offers a range of built-in and third-party tools designed to simplify the debugging process. Below are some of the most commonly used tools:
1. Angular DevTools
Angular DevTools is an official browser extension that helps developers inspect Angular applications. It provides insights into component structure, change detection, and performance.
Example: Using Angular DevTools
After installing Angular DevTools, you can inspect your application by navigating to the “Components” tab in the browser’s developer tools. Here, you can view the component hierarchy, explore component properties, and track the state of your application.
```typescript // Inspect component state in Angular DevTools @Component({ selector: 'app-example', template: `<div>{{ message }}</div>` }) export class ExampleComponent { message: string = 'Hello, Angular!'; } ```
2. Chrome DevTools
Chrome DevTools is a powerful set of developer tools built directly into the Chrome browser. It allows you to inspect and debug Angular applications, monitor network requests, and profile performance.
Example: Debugging with Breakpoints in Chrome DevTools
Set breakpoints in your TypeScript code by opening Chrome DevTools, navigating to the “Sources” tab, and selecting the TypeScript file you want to debug. You can set a breakpoint by clicking on the line number where you want the execution to pause.
```typescript // Set a breakpoint to inspect variable values const calculateSum = (a: number, b: number): number => { const sum = a + b; return sum; // Set breakpoint here }; ```
3. Augury
Augury is an open-source Chrome extension specifically designed for Angular applications. It provides a visual representation of your application’s component tree, router state, and allows you to explore component dependencies.
Example: Analyzing Component Tree with Augury
With Augury installed, you can view the entire component tree of your Angular application. This helps in understanding how components are nested and how data flows between them.
```typescript // Visualize the component tree with Augury @Component({ selector: 'app-parent', template: `<app-child></app-child>` }) export class ParentComponent {} @Component({ selector: 'app-child', template: `<p>Child Component</p>` }) export class ChildComponent {} ```
4. Debugging with Visual Studio Code (VS Code)
VS Code is a popular code editor with built-in debugging capabilities for Angular applications. You can set breakpoints, watch variables, and step through code to identify issues.
Example: Debugging in VS Code
To debug an Angular application in VS Code, you can use the “Debugger for Chrome” extension. Configure the launch.json file in your project to start a debugging session directly from the editor.
```json // launch.json configuration for debugging Angular in VS Code { "version": "0.2.0", "configurations": [ { "type": "chrome", "request": "launch", "name": "Launch Chrome against localhost", "url": "http://localhost:4200", "webRoot": "${workspaceFolder}" } ] } ```
Effective Debugging Techniques
1. Understanding Error Messages
Debugging is more than just using tools; it’s about employing the right techniques to find and fix issues efficiently. Here are some techniques to consider:
Angular’s error messages can be cryptic at times, but understanding them is key to effective debugging. Pay close attention to the stack trace and the error message details, as they often provide clues about the source of the problem.
Example: Interpreting an Error Message
If you encounter an error like `Error: Cannot find module ‘./app.module’`, it indicates that Angular is unable to locate the specified module. Double-check the module import paths and file names to resolve the issue.
2. Using Console Logs
Adding `console.log()` statements in your code is a simple yet effective way to debug issues. It helps you track variable values and understand the flow of your application.
Example: Logging Variable Values
```typescript const fetchData = () => { const data = apiService.getData(); console.log('Fetched Data:', data); // Log the data to inspect it return data; }; ```
3. Debugging Asynchronous Code
Asynchronous code, such as promises and observables, can be challenging to debug. Use tools like `async/await`, `subscribe`, and `tap` to gain more control over the execution flow.
Example: Debugging Observables
```typescript import { of } from 'rxjs'; import { tap } from 'rxjs/operators'; of('Hello, World!') .pipe( tap(message => console.log('Message:', message)) // Log the emitted value ) .subscribe(); ```
4. Analyzing Change Detection
Understanding Angular’s change detection mechanism is crucial for debugging performance issues. Tools like Angular DevTools can help you visualize and optimize change detection.
Example: Debugging Change Detection
```typescript @Component({ selector: 'app-change-detection', template: `<div>{{ value }}</div>`, changeDetection: ChangeDetectionStrategy.OnPush }) export class ChangeDetectionComponent { value: string = 'Initial Value'; } ```
Conclusion
Effective debugging in Angular requires a combination of the right tools and techniques. By mastering the use of Angular DevTools, Chrome DevTools, Augury, and VS Code, and employing effective debugging strategies, you can quickly identify and resolve issues in your Angular applications. Whether you’re dealing with complex asynchronous code or need to optimize change detection, these tools and techniques will help you maintain high-quality, performant applications.
Further Reading:
Table of Contents
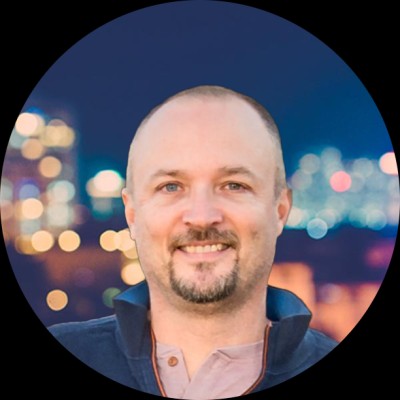
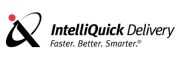