Angular Error Handling: Strategies for Debugging and Logging
Angular, being one of the most popular front-end frameworks for building web applications, offers a robust set of tools and features. However, like any complex system, it’s not immune to errors and bugs. Effective error handling is crucial for ensuring smooth user experiences and maintaining the integrity of your application. In this guide, we’ll delve into strategies for debugging and logging errors in Angular applications.
1. Understanding Error Handling in Angular
Error handling in Angular revolves around identifying, capturing, and responding to errors that occur during the application’s lifecycle. These errors can stem from various sources, including user input, server responses, or internal application logic. Angular provides mechanisms to intercept and manage these errors efficiently.
2. Built-in Error Handling Mechanisms
Angular offers several built-in features to facilitate error handling:
– ErrorInterceptor: Angular’s HttpClient module allows you to intercept HTTP requests and responses. Implementing an ErrorInterceptor enables centralized handling of HTTP errors, such as network failures or server-side errors.
– ErrorHandler: Angular provides an abstract class called ErrorHandler, which you can extend to create a custom error handling strategy for your application. By overriding its handleError method, you can define how your application should respond to unhandled errors.
3. Strategies for Debugging Errors
3.1. Utilizing Browser Developer Tools
Modern web browsers come equipped with powerful developer tools that offer a plethora of debugging features. These tools enable you to inspect the application’s DOM, monitor network requests, and debug JavaScript code effectively. Chrome Developer Tools, for instance, provides a rich set of features including breakpoints, console logging, and real-time code evaluation.
3.2. Leveraging Angular DevTools
Angular DevTools is a browser extension specifically designed for debugging Angular applications. It integrates seamlessly with Chrome and Firefox Developer Tools, providing additional insights into your application’s state, performance, and behavior. With Angular DevTools, you can inspect component hierarchies, track change detection cycles, and analyze routing transitions.
3.3. Implementing Unit Tests
Unit testing is an indispensable tool for identifying and preventing errors in your Angular application. By writing comprehensive unit tests using frameworks like Jasmine and Karma, you can validate individual components, services, and modules in isolation. This approach not only helps catch bugs early in the development process but also facilitates continuous integration and deployment pipelines.
4. Logging Strategies in Angular
4.1. Console Logging
Console logging is perhaps the simplest and most ubiquitous logging mechanism in JavaScript. Angular applications can leverage console.log, console.error, and other console methods to log relevant information, warnings, and errors to the browser console. While effective for debugging purposes, console logging should be used judiciously in production environments due to potential performance overhead.
4.2. Using Angular’s Logging Service
Angular provides a built-in logging service, accessible through the $log service or Angular’s dependency injection mechanism. By injecting the $log service into your components or services, you can log messages with varying levels of severity, such as debug, info, warn, and error. Additionally, Angular’s logging service offers flexibility in configuring log output destinations and formats.
4.3. Integrating Third-party Logging Libraries
Several third-party logging libraries, such as LogRocket, Sentry, and Splunk, offer advanced logging and monitoring capabilities for Angular applications. These libraries provide features like real-time error tracking, performance monitoring, and user session replay. Integrating such libraries into your Angular application can enhance its resilience and facilitate proactive error resolution.
Conclusion
Effective error handling is a fundamental aspect of Angular development, essential for ensuring the stability, reliability, and performance of your applications. By understanding the built-in error handling mechanisms, employing robust debugging strategies, and adopting appropriate logging practices, you can streamline the development process and deliver exceptional user experiences.
Implementing a comprehensive error handling strategy requires a combination of technical expertise, tools, and best practices. Continuously refining and optimizing your error handling approach will not only mitigate risks but also foster innovation and growth in your Angular projects.
Further Reading:
- Angular DevTools – Chrome Web Store – https://chromewebstore.google.com/
- Jasmine – JavaScript Testing Framework – https://jasmine.github.io/
- LogRocket – Angular Error Tracking and Logging – https://logrocket.com/angular-error-tracking
Table of Contents
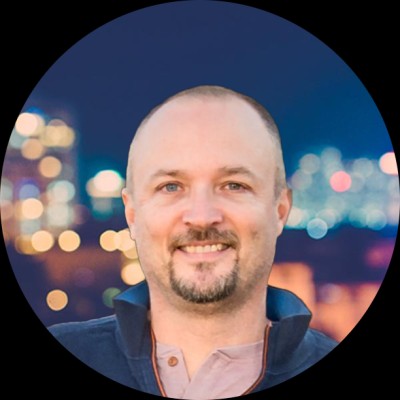
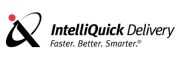