Angular and Firebase: Building Real-Time Apps with Serverless Backend
In today’s fast-paced digital world, real-time web applications have become increasingly popular. Users expect applications to respond instantaneously and reflect changes in real-time. To meet these expectations, developers often turn to Angular and Firebase, a powerful combination for building responsive and scalable applications with a serverless backend.
Table of Contents
This blog post will serve as a comprehensive guide to help you understand the fundamentals of building real-time web apps using Angular and Firebase. We’ll cover the key concepts, setup, and implementation with code samples along the way.
1. Why Choose Angular and Firebase?
1.1. Angular: A Robust Frontend Framework
Angular is a widely-used, open-source frontend framework maintained by Google. It provides developers with a structured and scalable approach to building single-page applications (SPAs). With its extensive set of features and components, Angular simplifies the development process and enhances code maintainability.
1.2. Firebase: A Versatile Backend-as-a-Service (BaaS)
Firebase is a Backend-as-a-Service (BaaS) platform by Google that allows developers to build powerful serverless applications effortlessly. It offers a variety of services such as Realtime Database, Authentication, Cloud Functions, Hosting, and more, eliminating the need for setting up and managing traditional server infrastructures. Firebase’s real-time capabilities make it an ideal choice for developing responsive applications.
2. Getting Started: Setting Up the Environment
Before diving into development, we need to set up our development environment. Ensure you have Node.js and npm (Node Package Manager) installed. Then, create a new Angular project using the Angular CLI:
bash npm install -g @angular/cli ng new real-time-app cd real-time-app
Next, install the Firebase CLI to interact with Firebase services:
bash npm install -g firebase-tools
3. Creating a Firebase Project
To start using Firebase, you need to create a Firebase project:
- Go to the Firebase Console.
- Click on “Add Project” and follow the prompts to set up your project.
- Once the project is created, you’ll be redirected to the project dashboard.
4. Setting Up Firebase in Angular
To integrate Firebase into your Angular project, you’ll need to install the Firebase package and configure it with your Firebase project credentials.
Step 1: Install Firebase
In your Angular project folder, run the following command to install the Firebase package:
bash npm install firebase
Step 2: Firebase Configuration
In your Angular project, navigate to the src/environments folder and create two new files: environment.ts and environment.prod.ts. These files will hold environment-specific configuration settings.
In the environment.ts file, add the Firebase configuration object from your Firebase project dashboard:
typescript // src/environments/environment.ts export const environment = { production: false, firebaseConfig: { apiKey: "YOUR_API_KEY", authDomain: "YOUR_AUTH_DOMAIN", projectId: "YOUR_PROJECT_ID", storageBucket: "YOUR_STORAGE_BUCKET", messagingSenderId: "YOUR_MESSAGING_SENDER_ID", appId: "YOUR_APP_ID", }, };
For the production environment, repeat the same process in the environment.prod.ts file with your production-specific Firebase configuration.
Step 3: Import Firebase Configuration
In your Angular app.module.ts file, import the Firebase configuration and initialize the Firebase app:
typescript // src/app/app.module.ts import { NgModule } from '@angular/core'; import { AngularFireModule } from '@angular/fire'; import { environment } from '../environments/environment'; @NgModule({ imports: [ // ... AngularFireModule.initializeApp(environment.firebaseConfig), // ... ], declarations: [/* ... */], bootstrap: [/* ... */] }) export class AppModule { }
5. Real-Time Data with Firebase Realtime Database
Firebase Realtime Database is a NoSQL cloud-hosted database that allows you to store and sync data in real-time. Let’s create a simple real-time chat application to demonstrate its capabilities.
Step 1: Set Up the Chat Interface
Create a new component for the chat interface:
bash ng generate component chat
In the chat.component.html file, add the basic chat interface elements:
html <!-- src/app/chat/chat.component.html --> <div class="chat-container"> <div class="chat-messages"> <div class="message" *ngFor="let message of messages"> <span class="username">{{ message.username }}:</span> <span class="content">{{ message.content }}</span> </div> </div> <div class="chat-input"> <input type="text" placeholder="Enter your message" [(ngModel)]="newMessage" (keyup.enter)="sendMessage()" /> </div> </div>
In the chat.component.ts file, define the properties and methods for the chat component:
typescript // src/app/chat/chat.component.ts import { Component, OnInit } from '@angular/core'; import { AngularFireDatabase, AngularFireList } from '@angular/fire/database'; import { Observable } from 'rxjs'; @Component({ selector: 'app-chat', templateUrl: './chat.component.html', styleUrls: ['./chat.component.css'] }) export class ChatComponent implements OnInit { messagesRef: AngularFireList<any>; messages: Observable<any[]>; newMessage: string = ''; constructor(private db: AngularFireDatabase) { this.messagesRef = this.db.list('messages'); this.messages = this.messagesRef.valueChanges(); } ngOnInit(): void { this.messages.subscribe(); } sendMessage(): void { if (this.newMessage.trim() !== '') { this.messagesRef.push({ username: 'User', // Replace this with the user's actual name or authentication system content: this.newMessage.trim(), timestamp: Date.now() }); this.newMessage = ''; } } }
Here, we’re using AngularFireDatabase to interact with the Firebase Realtime Database. The messagesRef is the reference to the “messages” node in the database, and the messages array is an observable of the data. We use valueChanges() to subscribe to changes in the database and update the messages in real-time.
Step 2: Styling the Chat Interface
For simplicity, we’ll use some basic CSS to style the chat component:
css /* src/app/chat/chat.component.css */ .chat-container { display: flex; flex-direction: column; justify-content: space-between; height: 100%; } .chat-messages { flex-grow: 1; overflow-y: auto; } .message { margin-bottom: 5px; } .username { font-weight: bold; } .chat-input { margin-top: 10px; }
Now that our chat interface is set up, let’s move on to displaying and syncing real-time messages with the Firebase Realtime Database.
6. Real-Time Data Sync with Firebase Realtime Database
With Firebase Realtime Database, data synchronization is automatic. When data changes in the database, connected clients receive real-time updates without needing to refresh the page. In our chat application, new messages will be automatically added to the chat interface as they are sent.
When a user sends a message, the sendMessage() method is called, pushing the new message object to the “messages” node in the database. AngularFireDatabase handles the synchronization, and any connected clients will receive the new message in real-time.
7. Implementing User Authentication with Firebase Authentication
Adding user authentication is crucial for many real-time applications, as it allows you to identify users and control access to certain features or data.
Firebase Authentication provides various authentication methods, including email/password, Google, Facebook, Twitter, and more. Let’s implement email/password authentication in our chat application.
Step 1: Enable Email/Password Authentication
In the Firebase Console, navigate to “Authentication” in the left sidebar. Under the “Sign-in method” tab, enable the “Email/Password” provider.
Step 2: Implement User Registration and Login
Create two new components for user registration and login:
bash ng generate component register ng generate component login
In the register.component.html file, add the registration form:
html <!-- src/app/register/register.component.html --> <div class="register-container"> <h2>Register</h2> <form (submit)="registerUser()"> <input type="email" placeholder="Email" [(ngModel)]="email" required /> <input type="password" placeholder="Password" [(ngModel)]="password" required /> <button type="submit">Register</button> </form> </div>
In the register.component.ts file, implement the registration functionality:
typescript // src/app/register/register.component.ts import { Component } from '@angular/core'; import { AngularFireAuth } from '@angular/fire/auth'; @Component({ selector: 'app-register', templateUrl: './register.component.html', styleUrls: ['./register.component.css'] }) export class RegisterComponent { email: string = ''; password: string = ''; constructor(private auth: AngularFireAuth) { } registerUser(): void { this.auth.createUserWithEmailAndPassword(this.email, this.password) .then(() => { console.log('User registered successfully!'); }) .catch((error) => { console.error('Registration error:', error); }); } }
Similarly, in the login.component.html and login.component.ts files, create the login form and implement the login functionality using signInWithEmailAndPassword().
Now, add routing for these components in the app-routing.module.ts file:
typescript // src/app/app-routing.module.ts import { NgModule } from '@angular/core'; import { RouterModule, Routes } from '@angular/router'; import { ChatComponent } from './chat/chat.component'; import { LoginComponent } from './login/login.component'; import { RegisterComponent } from './register/register.component'; const routes: Routes = [ { path: '', redirectTo: 'chat', pathMatch: 'full' }, { path: 'chat', component: ChatComponent }, { path: 'login', component: LoginComponent }, { path: 'register', component: RegisterComponent }, // Add more routes for other components if necessary ]; @NgModule({ imports: [RouterModule.forRoot(routes)], exports: [RouterModule] }) export class AppRoutingModule { }
Conclusion
In this comprehensive guide, we have explored the powerful combination of Angular and Firebase to build real-time web applications with a serverless backend. We covered setting up the development environment, configuring Firebase in Angular, and implementing real-time data synchronization using Firebase Realtime Database. Additionally, we learned how to add user authentication to our application.
This is just the beginning of what you can achieve with Angular and Firebase. From here, you can explore other Firebase services like Cloud Functions, Firestore, and Storage, to enhance your real-time app even further.
The combination of Angular’s frontend capabilities and Firebase’s serverless backend offers a potent solution for building modern, responsive, and real-time web applications. So, go ahead and start building your own real-time apps today!
Table of Contents
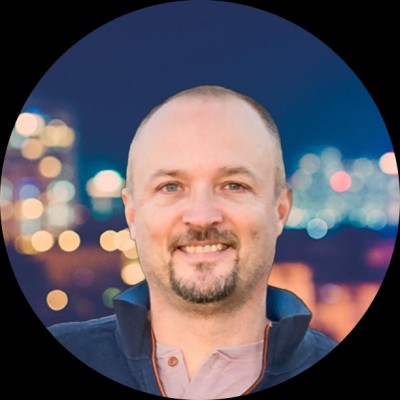
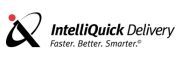