Angular and Firebase Cloud Firestore: Real-Time Data Sync
In modern web development, real-time data synchronization is critical for creating dynamic and responsive applications. Firebase Cloud Firestore, a NoSQL database, provides robust support for real-time data syncing, making it an excellent choice for Angular developers. This article explores how to integrate Firebase Cloud Firestore with Angular to achieve seamless real-time data synchronization, complete with practical code examples.
Understanding Firebase Cloud Firestore
Firebase Cloud Firestore is a flexible, scalable database for mobile, web, and server development. It provides real-time synchronization, allowing data to be updated across multiple clients simultaneously. Its integration with Angular can help build interactive, data-driven applications with ease.
Setting Up Angular with Firebase
To start using Firebase Cloud Firestore with Angular, you need to set up your Angular project and configure Firebase. Below are the key steps:
1. Creating an Angular Project
Begin by creating a new Angular project using Angular CLI.
```bash ng new angular-firebase-app cd angular-firebase-app ```
2. Installing Firebase and AngularFire
Install the necessary Firebase and AngularFire packages to enable Firebase integration.
```bash npm install firebase @angular/fire ```
3. Configuring Firebase
Set up Firebase in your Angular application by adding the Firebase configuration in your `environment.ts` file.
```typescript export const environment = { production: false, firebase: { apiKey: "YOUR_API_KEY", authDomain: "YOUR_PROJECT_ID.firebaseapp.com", projectId: "YOUR_PROJECT_ID", storageBucket: "YOUR_PROJECT_ID.appspot.com", messagingSenderId: "YOUR_MESSAGING_SENDER_ID", appId: "YOUR_APP_ID" } }; ```
4. Initializing Firebase
Import and initialize Firebase in your `AppModule`.
```typescript import { AngularFireModule } from '@angular/fire'; import { AngularFirestoreModule } from '@angular/fire/firestore'; import { environment } from '../environments/environment'; @NgModule({ declarations: [AppComponent], imports: [ BrowserModule, AngularFireModule.initializeApp(environment.firebase), AngularFirestoreModule ], providers: [], bootstrap: [AppComponent] }) export class AppModule { } ```
Real-Time Data Sync with Firestore
One of the key features of Firebase Cloud Firestore is its real-time capabilities. AngularFire makes it easy to use Firestore in Angular for real-time data synchronization.
1. Creating a Firestore Collection
To start working with data, create a Firestore collection and add some documents.
```typescript import { AngularFirestore } from '@angular/fire/firestore'; import { Injectable } from '@angular/core'; @Injectable({ providedIn: 'root' }) export class DataService { constructor(private firestore: AngularFirestore) { } getItems() { return this.firestore.collection('items').snapshotChanges(); } addItem(item: any) { return this.firestore.collection('items').add(item); } } ```
2. Syncing Data in Real-Time
You can now subscribe to real-time updates from Firestore in your Angular components.
```typescript import { Component, OnInit } from '@angular/core'; import { DataService } from './data.service'; @Component({ selector: 'app-item-list', template: ` <ul> <li *ngFor="let item of items"> {{ item.payload.doc.data().name }} </li> </ul> ` }) export class ItemListComponent implements OnInit { items: any[] = []; constructor(private dataService: DataService) { } ngOnInit(): void { this.dataService.getItems().subscribe(data => { this.items = data; }); } } ```
3. Adding Data to Firestore
You can easily add data to your Firestore collection, and it will be reflected in real-time across all clients.
```typescript import { Component } from '@angular/core'; import { DataService } from './data.service'; @Component({ selector: 'app-add-item', template: ` <input [(ngModel)]="itemName" placeholder="Item Name" /> <button (click)="addItem()">Add Item</button> ` }) export class AddItemComponent { itemName: string = ''; constructor(private dataService: DataService) { } addItem() { this.dataService.addItem({ name: this.itemName }); this.itemName = ''; } } ```
4. Deleting Data from Firestore
You can also delete data from your Firestore collection, and the changes will be synchronized in real-time.
```typescript deleteItem(itemId: string) { this.firestore.collection('items').doc(itemId).delete(); } ```
Conclusion
Integrating Angular with Firebase Cloud Firestore provides a powerful solution for real-time data synchronization. By leveraging Firebase’s real-time capabilities, you can build dynamic and responsive applications that provide an excellent user experience. Whether you’re creating a chat application, a collaborative tool, or any other data-driven application, Angular and Firebase Cloud Firestore can help you achieve seamless real-time functionality.
Further Reading:
Table of Contents
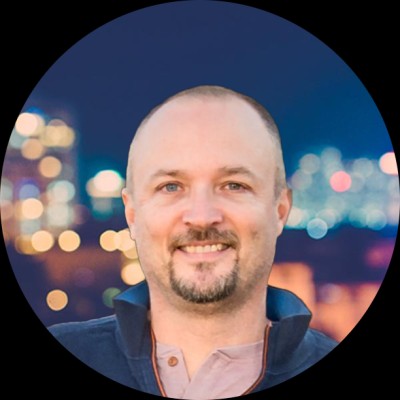
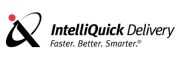