Angular and Firebase Realtime Database: Syncing Data in Real Time
Introduction to Real-Time Data Synchronization
Real-time data synchronization is essential for modern web applications, especially when dealing with dynamic data that needs to be updated instantly across multiple clients. Firebase Realtime Database offers a robust solution for real-time data sync, allowing data to be stored and synchronized in real-time across all connected clients.
Angular, with its powerful data-binding and component-based architecture, is an excellent frontend framework for building dynamic, real-time applications. By integrating Angular with Firebase Realtime Database, developers can create applications that respond instantly to data changes, providing a seamless user experience.
1. Setting Up Angular and Firebase
Before diving into real-time data synchronization, ensure you have an Angular project set up and Firebase configured. You can set up Firebase by creating a project in the Firebase Console and integrating it with your Angular application using the AngularFire library.
Example: Setting Up Firebase in Angular
```typescript // Install AngularFire and Firebase // npm install firebase @angular/fire import { NgModule } from '@angular/core'; import { BrowserModule } from '@angular/platform-browser'; import { AngularFireModule } from '@angular/fire'; import { AngularFireDatabaseModule } from '@angular/fire/database'; import { environment } from '../environments/environment'; @NgModule({ declarations: [ // Components ], imports: [ BrowserModule, AngularFireModule.initializeApp(environment.firebaseConfig), AngularFireDatabaseModule // Import the Firebase Realtime Database module ], providers: [], bootstrap: [/ Root Component /] }) export class AppModule { } ```
Ensure your environment file (`environment.ts`) contains the Firebase configuration details:
```typescript export const environment = { production: false, firebaseConfig: { apiKey: "YOUR_API_KEY", authDomain: "YOUR_AUTH_DOMAIN", databaseURL: "YOUR_DATABASE_URL", projectId: "YOUR_PROJECT_ID", storageBucket: "YOUR_STORAGE_BUCKET", messagingSenderId: "YOUR_MESSAGING_SENDER_ID", appId: "YOUR_APP_ID" } }; ```
2. Syncing Data in Real-Time
With Firebase configured, you can now implement real-time data synchronization in your Angular application. Firebase Realtime Database allows you to create, read, update, and delete (CRUD) data while instantly syncing changes across all clients.
Example: Reading Data in Real-Time
```typescript import { Component, OnInit } from '@angular/core'; import { AngularFireDatabase } from '@angular/fire/database'; import { Observable } from 'rxjs'; @Component({ selector: 'app-realtime-data', template: ` <ul> <li ngFor="let item of items | async">{{ item.name }}</li> </ul> ` }) export class RealtimeDataComponent implements OnInit { items: Observable<any[]>; constructor(private db: AngularFireDatabase) {} ngOnInit() { this.items = this.db.list('/items').valueChanges(); } } ```
3. Writing Data to Firebase Realtime Database
Writing data to Firebase is straightforward and follows the same real-time synchronization model. When data is written, all clients connected to the database are instantly updated.
Example: Adding Data to Firebase
```typescript import { Component } from '@angular/core'; import { AngularFireDatabase } from '@angular/fire/database'; @Component({ selector: 'app-add-item', template: ` <input [(ngModel)]="newItemName" placeholder="New Item" /> <button (click)="addItem()">Add Item</button> ` }) export class AddItemComponent { newItemName: string = ''; constructor(private db: AngularFireDatabase) {} addItem() { this.db.list('/items').push({ name: this.newItemName }); this.newItemName = ''; } } ```
4. Updating and Deleting Data in Real-Time
Updating and deleting data is also handled in real-time, ensuring all connected clients reflect the changes immediately.
Example: Updating Data in Firebase
```typescript updateItem(key: string, newName: string) { this.db.list('/items').update(key, { name: newName }); } ```
Example: Deleting Data from Firebase
```typescript deleteItem(key: string) { this.db.list('/items').remove(key); } ```
5. Advanced Real-Time Sync Techniques
Firebase also provides advanced features such as querying data, handling offline scenarios, and integrating with other Firebase services like Cloud Functions for more complex data synchronization and processing needs.
Example: Querying Data
```typescript this.items = this.db.list('/items', ref => ref.orderByChild('name').equalTo('specificName')).valueChanges(); ```
Conclusion
Integrating Angular with Firebase Realtime Database allows developers to build powerful, real-time applications with ease. By leveraging Firebase’s real-time synchronization capabilities and Angular’s dynamic frontend framework, you can create applications that provide a seamless and responsive user experience.
Further Reading:
Table of Contents
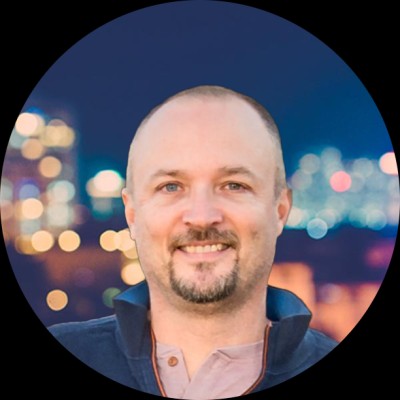
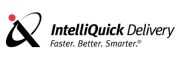