Angular and GraphQL: Modern API Integration
In the ever-evolving world of web development, providing efficient data management and integration capabilities is a top priority. Angular, a popular front-end framework, and GraphQL, a modern API query language, have gained significant traction for their ability to streamline data retrieval and manipulation processes. In this blog, we’ll explore how to integrate Angular with GraphQL to create powerful and performant applications. We’ll cover the fundamentals of GraphQL, how it differs from traditional REST APIs, and demonstrate code samples for seamless API integration within Angular.
Table of Contents
1. Understanding GraphQL:
GraphQL is a query language for APIs that enables clients to request only the data they need and nothing more. Unlike traditional REST APIs, where each endpoint corresponds to a specific resource, GraphQL provides a single endpoint through which clients can query and mutate data. This reduces the number of requests required and avoids over-fetching, leading to improved performance and reduced payload size.
1.1. Advantages of GraphQL over REST:
- Efficient Data Fetching: Clients can specify their data requirements precisely, avoiding the problem of over-fetching.
- Rapid Iteration and Versioning: GraphQL allows you to introduce changes to your API without impacting existing clients.
- Strongly Typed Schema: GraphQL schemas define the structure of the data, enabling better validation and documentation.
- Multiple Data Sources: GraphQL can aggregate data from various sources, providing a unified API.
- Declarative Data Fetching: Clients can request data with a single query, simplifying complex data retrieval.
1.2. GraphQL Schema and Types:
In GraphQL, the schema defines the data types and the structure of the data that can be queried or modified. It acts as a contract between the client and the server. The schema consists of Types, Queries, and Mutations.
A Type represents an object with specific fields, and it can be an Object Type, Scalar Type, Interface Type, Union Type, or Enum Type.
A Query defines the read operations that clients can perform to fetch data.
A Mutation defines the write operations that clients can perform to modify data.
2. Getting Started with Angular:
Before diving into GraphQL integration, let’s set up an Angular project and understand the basics of building components and services.
2.1. Setting up an Angular Project:
To create a new Angular project, ensure that you have Node.js and npm installed. Open your terminal and run the following command:
bash npx @angular/cli new my-angular-app cd my-angular-app
This will generate a new Angular project with the name my-angular-app. Next, serve the application locally to verify that it’s working:
bash ng serve
Visit http://localhost:4200 in your browser, and you should see the default Angular app.
2.2. Building Components and Services:
In Angular, components are the building blocks of the application user interface, while services handle business logic and data retrieval. Let’s create a simple component and service for demonstration purposes.
First, generate a new component using the Angular CLI:
bash ng generate component my-component
This will create a new folder named my-component with all the necessary files. Now, let’s create a service to fetch data. Run the following command:
bash ng generate service data
This will generate a new service file data.service.ts within the data folder.
Now, open the data.service.ts file and create a basic method to fetch some mock data:
typescript import { Injectable } from '@angular/core'; @Injectable({ providedIn: 'root', }) export class DataService { getMockData() { return [ { id: 1, name: 'John Doe', age: 28 }, { id: 2, name: 'Jane Smith', age: 32 }, { id: 3, name: 'Bob Johnson', age: 25 }, ]; } }
Next, open the my-component.component.ts file and import the DataService:
typescript import { Component, OnInit } from '@angular/core'; import { DataService } from '../data/data.service'; @Component({ selector: 'app-my-component', templateUrl: './my-component.component.html', styleUrls: ['./my-component.component.css'], }) export class MyComponentComponent implements OnInit { mockData: any[]; constructor(private dataService: DataService) {} ngOnInit(): void { this.mockData = this.dataService.getMockData(); } }
In the above code, we’ve imported the DataService and used it to fetch mock data. We store the data in the mockData property of the component.
Now, let’s display this data in the my-component.component.html template:
html <h2>Mock Data</h2> <ul> <li *ngFor="let item of mockData">{{ item.name }} (Age: {{ item.age }})</li> </ul>
Finally, add the app-my-component selector to the app.component.html file to display the MyComponentComponent:
html <app-my-component></app-my-component>
After making these changes, when you visit http://localhost:4200, you should see the list of mock data displayed on the page.
3. Integrating GraphQL with Angular:
Now that we have our Angular project set up, let’s integrate GraphQL and see how we can use it to fetch data from a server.
3.1. Installing Dependencies:
To use GraphQL in Angular, we’ll need the Apollo Client library. Apollo Client is a fully-featured GraphQL client that helps manage data fetching and state management in the client-side application. Install the necessary packages using npm:
bash npm install apollo-angular apollo-angular-http graphql
The apollo-angular package provides the Angular integration, while apollo-angular-http enables HTTP communication with the GraphQL server. graphql contains necessary types and functions for working with GraphQL queries and mutations.
3.2. Configuring GraphQL Endpoint:
Before we start querying data, we need a GraphQL server with an appropriate API endpoint. For this blog, we’ll use a mock GraphQL server provided by GraphQL Faker. GraphQL Faker allows us to quickly set up a fake GraphQL API with sample data.
First, install GraphQL Faker globally:
bash npm install -g graphql-faker
Next, create a new file called schema.graphql in the root of your Angular project. This file will define the schema for our mock API:
graphql type User { id: ID! name: String! age: Int! } type Query { users: [User!]! }
In this schema, we define a User type with fields id, name, and age, and a Query type with a users field that returns an array of users.
Now, in the terminal, run the following command to start the mock GraphQL server:
bash graphql-faker --extend schema.graphql
This will generate a mock GraphQL API with the specified schema, and you can access it at http://localhost:9002/graphql.
3.3. Writing GraphQL Queries and Mutations:
With the GraphQL server running, it’s time to write GraphQL queries and mutations to fetch and modify data. In Angular, we’ll use the Apollo Client to send GraphQL requests.
First, import the necessary modules in the app.module.ts:
typescript import { NgModule } from '@angular/core'; import { BrowserModule } from '@angular/platform-browser'; import { HttpClientModule } from '@angular/common/http'; import { ApolloModule, APOLLO_OPTIONS } from 'apollo-angular'; import { HttpLinkModule, HttpLink } from 'apollo-angular/http'; import { InMemoryCache } from 'apollo-cache-inmemory';
Next, create the Apollo Client and set up the GraphQL endpoint in the same file:
typescript @NgModule({ imports: [ BrowserModule, HttpClientModule, ApolloModule, HttpLinkModule, ], providers: [ { provide: APOLLO_OPTIONS, useFactory: (httpLink: HttpLink) => { return { cache: new InMemoryCache(), link: httpLink.create({ uri: 'http://localhost:9002/graphql', // Your GraphQL server URL }), }; }, deps: [HttpLink], }, ], bootstrap: [AppComponent], }) export class AppModule {}
Now, let’s update the data.service.ts file to use Apollo Client to fetch data:
typescript import { Injectable } from '@angular/core'; import { Apollo } from 'apollo-angular'; import { gql } from 'graphql-tag'; @Injectable({ providedIn: 'root', }) export class DataService { constructor(private apollo: Apollo) {} getUsers() { return this.apollo.query<any>({ query: gql` query { users { id name age } } `, }); } }
In the above code, we use the this.apollo.query method to send the GraphQL query to fetch users. The gql function is used to define the query using a tagged template literal.
Now, we need to update the MyComponentComponent to use the DataService to fetch data from the GraphQL server:
typescript import { Component, OnInit } from '@angular/core'; import { DataService } from '../data/data.service'; @Component({ selector: 'app-my-component', templateUrl: './my-component.component.html', styleUrls: ['./my-component.component.css'], }) export class MyComponentComponent implements OnInit { users: any[]; constructor(private dataService: DataService) {} ngOnInit(): void { this.dataService.getUsers().subscribe((result) => { this.users = result.data.users; }); } }
Finally, update the my-component.component.html template to display the users fetched from the GraphQL server:
html <h2>Users</h2> <ul> <li *ngFor="let user of users">{{ user.name }} (Age: {{ user.age }})</li> </ul>
With these changes, your Angular app will now fetch data from the mock GraphQL server and display it on the page.
4. Handling Data with Apollo Client:
Apollo Client provides powerful features for handling data, including caching, optimistic UI, and more.
4.1. Introduction to Apollo Client:
Apollo Client comes with a built-in cache that stores GraphQL query results. When you re-run a query, Apollo Client first checks the cache for the data. If the data is already available, it returns it from the cache instead of making a new network request.
This cache improves application performance by reducing the number of network requests and helps maintain a consistent state across the application.
4.2. Querying Data with Apollo Client:
In the previous section, we used this.apollo.query to fetch data from the GraphQL server. By default, Apollo Client caches the results of queries automatically. If the same query is executed again, Apollo Client retrieves the data from the cache.
typescript import { Injectable } from '@angular/core'; import { Apollo } from 'apollo-angular'; import { gql } from 'graphql-tag'; @Injectable({ providedIn: 'root', }) export class DataService { constructor(private apollo: Apollo) {} getUsers() { return this.apollo.query<any>({ query: gql` query { users { id name age } } `, }); } }
In this example, if we call getUsers() multiple times within the application, Apollo Client will use the cached data when possible.
4.3. Mutating Data with Apollo Client:
Mutations are used to modify data on the server. Apollo Client provides a similar method, this.apollo.mutate, to perform mutations.
For example, let’s create a mutation to add a new user:
typescript import { Injectable } from '@angular/core'; import { Apollo } from 'apollo-angular'; import { gql } from 'graphql-tag'; @Injectable({ providedIn: 'root', }) export class DataService { constructor(private apollo: Apollo) {} getUsers() { return this.apollo.query<any>({ query: gql` query { users { id name age } } `, }); } addUser(name: string, age: number) { return this.apollo.mutate<any>({ mutation: gql` mutation AddUser($name: String!, $age: Int!) { addUser(name: $name, age: $age) { id name age } } `, variables: { name, age, }, update: (cache, { data: { addUser } }) => { const { users } = cache.readQuery<any>({ query: gql` query { users { id name age } } `, }); cache.writeQuery({ query: gql` query { users { id name age } } `, data: { users: [...users, addUser], }, }); }, }); } }
In the DataService, we have added a new method addUser that sends a mutation to add a new user. The addUser mutation takes two variables: name and age, which are used to create a new user on the server.
The update function inside the this.apollo.mutate call is used to update the cache after the mutation is executed. We read the existing users data from the cache using cache.readQuery, then we add the new user returned by the mutation to the existing users data and write the updated data back to the cache using cache.writeQuery.
Now, let’s use this new mutation in the MyComponentComponent to add a user when a button is clicked:
typescript import { Component, OnInit } from '@angular/core'; import { DataService } from '../data/data.service'; @Component({ selector: 'app-my-component', templateUrl: './my-component.component.html', styleUrls: ['./my-component.component.css'], }) export class MyComponentComponent implements OnInit { users: any[]; constructor(private dataService: DataService) {} ngOnInit(): void { this.dataService.getUsers().subscribe((result) => { this.users = result.data.users; }); } addUser() { const name = 'New User'; const age = 25; this.dataService.addUser(name, age).subscribe((result) => { const newUser = result.data.addUser; this.users.push(newUser); }); } }
In the addUser method, we call the addUser mutation from the DataService and provide the name and age of the new user. After the mutation is executed successfully, we add the newly created user to the users array in the component.
In the my-component.component.html, let’s add a button to trigger the addUser method:
html <h2>Users</h2> <ul> <li *ngFor="let user of users">{{ user.name }} (Age: {{ user.age }})</li> </ul> <button (click)="addUser()">Add New User</button>
Now, when you click the “Add New User” button, a new user with the name “New User” and age 25 will be added to the list of users on the page. The data will also be updated in the cache, ensuring consistency across the application.
5. Optimizing GraphQL Queries:
As your application grows, you may need to optimize GraphQL queries to reduce the number of requests and improve performance. Here are some techniques to optimize your queries:
5.1. Query Batching:
Instead of sending multiple small queries, you can batch them into a single request. Apollo Client automatically batches multiple queries into a single HTTP request, reducing network overhead.
5.2. Caching and Data Normalization:
Apollo Client caches query results by default. This helps avoid redundant requests and ensures a faster user experience. Additionally, you can normalize data in the cache to prevent duplication and efficiently manage updates across the application.
6. Real-time Data with GraphQL Subscriptions:
GraphQL supports real-time data through subscriptions. Subscriptions allow the server to push data updates to clients, enabling real-time features like live notifications and chat.
To use subscriptions, you’ll need a server that supports GraphQL subscriptions. Popular options include Apollo Server, GraphQL Yoga, and AWS AppSync.
Here’s an example of setting up a subscription to receive real-time updates when a new user is added:
typescript import { Injectable } from '@angular/core'; import { Apollo } from 'apollo-angular'; import { gql } from 'graphql-tag'; import { Observable } from 'rxjs'; @Injectable({ providedIn: 'root', }) export class DataService { constructor(private apollo: Apollo) {} getUsers(): Observable<any> { return this.apollo.query<any>({ query: gql` query { users { id name age } } `, }); } subscribeToNewUsers(): Observable<any> { return this.apollo.subscribe<any>({ query: gql` subscription { newUser { id name age } } `, }); } }
In this example, we’ve added a new method subscribeToNewUsers that sets up a subscription to receive new user updates from the server.
In the MyComponentComponent, we can subscribe to new user updates and update the user list when a new user is added:
typescript import { Component, OnInit } from '@angular/core'; import { DataService } from '../data/data.service'; @Component({ selector: 'app-my-component', templateUrl: './my-component.component.html', styleUrls: ['./my-component.component.css'], }) export class MyComponentComponent implements OnInit { users: any[]; constructor(private dataService: DataService) {} ngOnInit(): void { this.dataService.getUsers().subscribe((result) => { this.users = result.data.users; }); this.dataService.subscribeToNewUsers().subscribe((result) => { const newUser = result.data.newUser; this.users.push(newUser); }); } addUser() { // ... } }
Now, when a new user is added to the server, the newUser subscription will be triggered, and the user list will be updated in real-time without the need to refresh the page.
7. Securing GraphQL Endpoints:
Securing your GraphQL endpoints is crucial to protect your application and data from unauthorized access. Here are some best practices to ensure security:
7.1. Authentication and Authorization:
Implement authentication mechanisms like JWT (JSON Web Tokens) or OAuth to verify the identity of clients. Use authorization rules to control what data clients can access.
7.2. Rate Limiting and Query Cost Analysis:
Implement rate limiting to prevent abuse and protect your server from excessive requests. Also, analyze the cost of complex queries to prevent performance bottlenecks.
8. Best Practices for Angular and GraphQL Integration:
To ensure a robust and scalable integration of Angular with GraphQL, consider the following best practices:
8.1. Separation of Concerns:
Adhere to the principles of clean architecture and separate the UI, business logic, and data access layers. Keep GraphQL queries and mutations in separate files for better organization.
8.2. Error Handling and Debugging:
Handle errors gracefully and provide meaningful feedback to users. Use tools like Apollo DevTools and GraphQL Playground for easy debugging during development.
8.3. Performance Optimization:
Optimize your queries to fetch only the necessary data. Leverage caching and data normalization to minimize network requests and improve application performance.
Conclusion
In this blog, we explored how to integrate Angular with GraphQL, a modern API integration technique. We covered the fundamentals of GraphQL, setting up an Angular project, and using Apollo Client for seamless data retrieval and manipulation. We also discussed optimizing queries, real-time data with subscriptions, securing GraphQL endpoints, and best practices for a successful integration.
With Angular and GraphQL together, you can create powerful and performant applications that provide efficient data management and real-time updates. As you continue your journey with Angular and GraphQL, keep experimenting and leveraging the full potential of these technologies to build remarkable web experiences. Happy coding!
Table of Contents
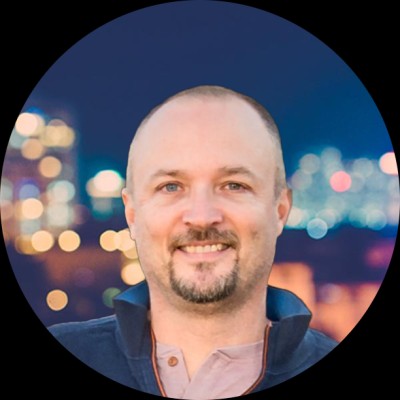
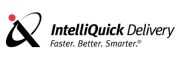