Angular and GraphQL with Apollo Client: Efficient Data Fetching
In the world of modern web development, efficiently managing data fetching is key to building responsive and user-friendly applications. Angular, coupled with GraphQL and Apollo Client, provides a powerful combination for managing data queries and mutations. This article explores how to integrate GraphQL with Angular using Apollo Client, providing practical examples of efficient data fetching strategies.
Understanding GraphQL and Apollo Client
GraphQL is a query language for APIs that enables clients to request exactly the data they need, minimizing over-fetching or under-fetching issues commonly associated with RESTful APIs. Apollo Client is a popular JavaScript library that helps manage data fetching in GraphQL-based applications, offering features like caching, state management, and real-time updates.
Setting Up Angular with Apollo Client
Before diving into examples, let’s set up an Angular project with Apollo Client for GraphQL integration.
1. Installing Necessary Packages
To start, you need to install the required packages in your Angular project. These include Apollo Client, Angular Apollo, and GraphQL.
```bash npm install @apollo/client @apollo/angular graphql ```
2. Configuring Apollo Client in Angular
After installing the necessary packages, configure Apollo Client in your Angular application by creating an Apollo module.
```typescript import { NgModule } from '@angular/core'; import { ApolloClientOptions, InMemoryCache, ApolloLink } from '@apollo/client/core'; import { ApolloModule, APOLLO_OPTIONS } from 'apollo-angular'; import { HttpLink } from 'apollo-angular/http'; @NgModule({ imports: [ApolloModule], providers: [ { provide: APOLLO_OPTIONS, useFactory: (httpLink: HttpLink): ApolloClientOptions<any> => { return { link: httpLink.create({ uri: 'https://your-graphql-endpoint.com/graphql' }), cache: new InMemoryCache(), }; }, deps: [HttpLink], }, ], }) export class GraphQLModule {} ```
This configuration sets up Apollo Client with a basic HTTP link and in-memory cache. Replace `’https://your-graphql-endpoint.com/graphql’` with your actual GraphQL endpoint.
Fetching Data with GraphQL Queries
Once the setup is complete, you can start fetching data using GraphQL queries.
Example 1: Querying Data with Apollo Client
Here’s how to use Apollo Client to perform a simple query in an Angular component.
```typescript import { Component, OnInit } from '@angular/core'; import { Apollo, gql } from 'apollo-angular'; const GET_USERS = gql` query GetUsers { users { id name email } } `; @Component({ selector: 'app-user-list', template: ` <div *ngIf="loading">Loading...</div> <ul *ngIf="!loading"> <li *ngFor="let user of users"> {{ user.name }} ({{ user.email }}) </li> </ul> `, }) export class UserListComponent implements OnInit { users: any[] = []; loading = true; constructor(private apollo: Apollo) {} ngOnInit() { this.apollo .watchQuery({ query: GET_USERS, }) .valueChanges.subscribe((result: any) => { this.users = result?.data?.users; this.loading = result.loading; }); } } ```
In this example, the `GET_USERS` query fetches user data, and the `Apollo` service is used to execute the query and handle the result.
Mutating Data with GraphQL
GraphQL is not just for querying data; it also allows you to perform mutations to change data on the server.
Example 2: Creating New Data with a Mutation
Here’s an example of how to use Apollo Client to perform a mutation.
```typescript import { Component } from '@angular/core'; import { Apollo, gql } from 'apollo-angular'; const ADD_USER = gql` mutation AddUser($name: String!, $email: String!) { addUser(name: $name, email: $email) { id name email } } `; @Component({ selector: 'app-add-user', template: ` <form (submit)="addUser()"> <input [(ngModel)]="name" placeholder="Name" required /> <input [(ngModel)]="email" placeholder="Email" required /> <button type="submit">Add User</button> </form> `, }) export class AddUserComponent { name: string = ''; email: string = ''; constructor(private apollo: Apollo) {} addUser() { this.apollo.mutate({ mutation: ADD_USER, variables: { name: this.name, email: this.email, }, }).subscribe(response => { console.log('User added:', response.data); }); } } ```
In this example, the `ADD_USER` mutation adds a new user by sending the `name` and `email` as variables.
Handling Errors and Loading States
Efficient data fetching involves more than just querying and mutating; handling errors and loading states are equally important.
Example 3: Managing Loading and Error States
You can enhance the user experience by managing loading and error states effectively.
```typescript ngOnInit() { this.apollo .watchQuery({ query: GET_USERS, }) .valueChanges.subscribe({ next: (result) => { this.users = result?.data?.users; this.loading = result.loading; }, error: (error) => { console.error('Error fetching data:', error); this.loading = false; }, }); } ```
This code snippet includes error handling to ensure the application gracefully handles any issues during data fetching.
Optimizing Data Fetching with Apollo Client
Apollo Client offers advanced features like caching, pagination, and real-time updates that can significantly optimize data fetching in your Angular application.
Example 4: Using Apollo Client’s Cache
By leveraging Apollo Client’s in-memory cache, you can optimize data fetching by reducing redundant network requests.
```typescript this.apollo.watchQuery({ query: GET_USERS, fetchPolicy: 'cache-first', }) .valueChanges.subscribe((result) => { this.users = result?.data?.users; }); ```
Setting the `fetchPolicy` to `’cache-first’` ensures that data is fetched from the cache if available, reducing the need for unnecessary network requests.
Conclusion
Integrating GraphQL with Angular using Apollo Client provides a powerful approach to efficient data fetching in modern web applications. By leveraging the capabilities of GraphQL and Apollo Client, developers can create highly responsive and data-driven applications. Whether you’re querying, mutating, or optimizing data fetches, this combination offers the tools needed for robust and scalable application development.
Further Reading:
Table of Contents
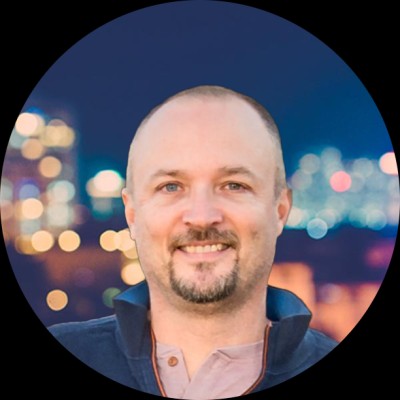
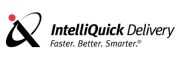