Understanding Angular Lifecycle Hooks: Managing Component Behavior
In the world of web development, creating dynamic and interactive user interfaces is crucial for providing a seamless user experience. Angular, as one of the leading frontend frameworks, offers a powerful set of tools to achieve this. One such set of tools is Angular Lifecycle Hooks, which allows developers to control and manage the behavior of components during their lifecycle. Understanding these lifecycle hooks is fundamental for building efficient and robust Angular applications. In this blog post, we will dive into the concept of Angular Lifecycle Hooks, explore their functionalities, and provide practical code examples to illustrate their usage.
Table of Contents
1. What are Angular Lifecycle Hooks?
Angular Lifecycle Hooks are predefined methods provided by the Angular framework that allow developers to tap into specific points of a component’s lifecycle. These points include the creation, rendering, and destruction of components. By leveraging these hooks, developers can execute custom logic at different stages of a component’s life, enabling them to control the component’s behavior effectively.
2. The Lifecycle of an Angular Component
Before delving into the details of individual lifecycle hooks, let’s briefly understand the typical lifecycle of an Angular component:
- Creation: This phase starts when Angular creates a component by calling its constructor. At this point, the component’s properties are not initialized yet.
- Initialization: In this phase, Angular sets the component’s input properties and calls the ngOnInit hook.
- Change Detection: After the initialization, Angular automatically triggers the change detection mechanism to update the component’s view if any bound data has changed.
- Content Projection: This phase occurs if the component contains projected content within its template.
- View and Content Initialization: Angular initializes the component’s view and content in this phase, which involves rendering the template and its child components.
- Change Detection (Again): After initializing the view, Angular performs change detection once more to ensure any updates are reflected.
- Component Destruction: When a component is no longer needed, Angular calls the ngOnDestroy hook before removing it from the DOM.
3. Commonly Used Lifecycle Hooks
Angular provides several lifecycle hooks that developers can use to interact with a component at various stages of its lifecycle. Let’s explore some of the most commonly used lifecycle hooks:
3.1. ngOnInit – Initialization Hook
The ngOnInit hook is one of the most frequently used lifecycle hooks. It is called after Angular initializes the component’s input properties and sets up its view. Developers often use this hook to perform initialization tasks, such as fetching data from a server, initializing variables, or subscribing to observables.
Here’s an example of how to use the ngOnInit hook:
typescript import { Component, OnInit } from '@angular/core'; @Component({ selector: 'app-example', template: '<p>{{ message }}</p>', }) export class ExampleComponent implements OnInit { message: string = ''; ngOnInit() { this.message = 'Hello, Angular!'; } }
In this example, the ngOnInit hook is used to set the message property of the component to ‘Hello, Angular!’. This message will be displayed in the template when the component is rendered.
3.2. ngOnChanges – Input Change Hook
The ngOnChanges hook is called whenever any input property of the component changes. It receives an object containing the changes, allowing developers to react to these changes and update the component’s behavior accordingly.
Here’s an example of how to use the ngOnChanges hook:
typescript import { Component, OnChanges, SimpleChanges } from '@angular/core'; @Component({ selector: 'app-example', template: '<p>{{ message }}</p>', }) export class ExampleComponent implements OnChanges { message: string = ''; ngOnChanges(changes: SimpleChanges) { if (changes.message) { this.message = changes.message.currentValue; } } }
In this example, the ngOnChanges hook is used to update the message property whenever its value changes. This ensures that the component’s behavior reflects the latest input.
3.3. ngAfterViewInit – View Initialization Hook
The ngAfterViewInit hook is called after Angular initializes the component’s view and its child views. This is particularly useful when you need to interact with the DOM or perform any action that relies on the component’s template being fully rendered.
Here’s an example of how to use the ngAfterViewInit hook:
typescript import { Component, AfterViewInit, ViewChild, ElementRef } from '@angular/core'; @Component({ selector: 'app-example', template: '<p #messageElement>{{ message }}</p>', }) export class ExampleComponent implements AfterViewInit { message: string = ''; @ViewChild('messageElement', { static: false }) messageElement: ElementRef; ngAfterViewInit() { this.messageElement.nativeElement.style.color = 'blue'; } }
In this example, the ngAfterViewInit hook is used to set the text color of the messageElement to blue. Since ngAfterViewInit is called after the view is fully initialized, we can safely access and manipulate the DOM elements.
3.4. ngOnDestroy – Destruction Hook
The ngOnDestroy hook is called just before Angular destroys a component. This is the ideal place to perform cleanup tasks, such as unsubscribing from subscriptions and releasing resources, to prevent memory leaks.
Here’s an example of how to use the ngOnDestroy hook:
typescript import { Component, OnDestroy } from '@angular/core'; import { Subscription } from 'rxjs'; @Component({ selector: 'app-example', template: '<p>Component Content</p>', }) export class ExampleComponent implements OnDestroy { private dataSubscription: Subscription; constructor(private dataService: DataService) { this.dataSubscription = this.dataService.getData().subscribe((data) => { // Do something with the data }); } ngOnDestroy() { this.dataSubscription.unsubscribe(); } }
In this example, the ngOnDestroy hook is used to unsubscribe from the data service’s subscription, ensuring that there are no memory leaks when the component is destroyed.
Conclusion
Angular Lifecycle Hooks play a crucial role in managing component behavior in Angular applications. They allow developers to execute custom logic at specific points during a component’s lifecycle, enabling efficient control over the component’s behavior. In this blog post, we have explored some of the most commonly used lifecycle hooks, including ngOnInit, ngOnChanges, ngAfterViewInit, and ngOnDestroy. By mastering these lifecycle hooks, you can build more robust and responsive Angular applications. Happy coding!
Table of Contents
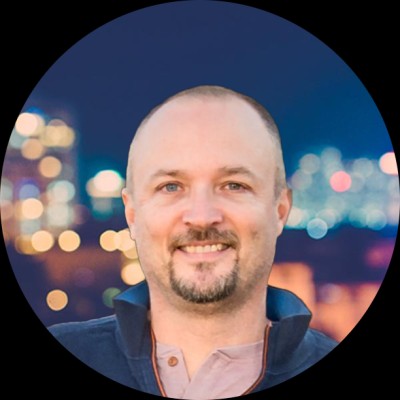
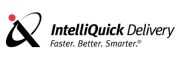