Exploring Angular Dependency Injection: Managing Application Dependencies
Modern web development frameworks like Angular provide developers with powerful tools to build complex, scalable, and maintainable applications. One such fundamental feature is Dependency Injection (DI), which plays a crucial role in managing application dependencies. In this blog, we will delve into the world of Angular Dependency Injection and understand its significance in creating robust and flexible applications.
Table of Contents
1. Understanding Dependency Injection
1.1. What is Dependency Injection?
Dependency Injection is a design pattern used in software development, wherein the dependencies of a class or component are provided externally rather than being created internally. In simpler terms, instead of a class creating its dependencies, they are “injected” into it from outside. This approach decouples the classes from their dependencies, making the code more modular, maintainable, and easier to test.
In Angular, the DI framework acts as a mediator, resolving and injecting the required dependencies into components, services, or other classes as requested.
1.2. Why Use Dependency Injection in Angular?
Dependency Injection offers several advantages in Angular development:
- Modularity: By having components rely on external services and modules, each part of the application becomes more self-contained and less reliant on specific implementation details.
- Maintainability: Decoupling dependencies from the components ensures that changes in one part of the application are less likely to impact other parts, reducing the risk of bugs and making maintenance more manageable.
- Reusability: Components can be easily reused across different parts of the application or even in other projects, as long as their dependencies are satisfied.
- Testability: Separating the creation of dependencies allows for easier testing, as mock or stub services can be provided during testing to isolate the component’s behavior.
2. Implementing Dependency Injection in Angular
2.1. The Injector
In Angular, the Injector is the heart of the Dependency Injection system. It is responsible for creating instances of classes with their required dependencies. The injector maintains a container that holds all the registered dependencies and knows how to resolve them when requested.
Angular provides the root injector for the entire application and also allows creating additional injectors for specific contexts, forming a hierarchical injection system.
2.1. Registering Dependencies
Before we can inject dependencies, we need to register them with Angular’s DI system. This is typically done by providing them at the root level or at a specific component level using the @Injectable decorator or providers array.
typescript // Example of a simple service import { Injectable } from '@angular/core'; @Injectable({ providedIn: 'root', }) export class DataService { // Service implementation here }
In this example, we have a DataService class that we want to be available throughout the application. By specifying { providedIn: ‘root’ }, we tell Angular to register this service with the root injector.
2.2. Constructor Injection vs. Property Injection
Angular supports two main approaches for dependency injection: constructor injection and property injection.
- Constructor Injection: In this approach, the required dependencies are passed to the class constructor as arguments.
typescript @Injectable({ providedIn: 'root', }) export class UserService { constructor(private dataService: DataService) { // Constructor logic here } }
- Property Injection: In this approach, the dependencies are declared as class properties with the @Inject decorator.
typescript @Injectable({ providedIn: 'root', }) export class UserService { @Inject(DataService) private dataService: DataService; // Class implementation here }
While both approaches achieve the same result, constructor injection is generally considered the best practice as it ensures that the dependencies are available as soon as the class is instantiated.
2.3. Hierarchical Injectors
Angular’s DI system operates using a hierarchical injection mechanism. When a component requests a dependency, Angular’s injector looks for it in the following order:
- The component’s own injector.
- If not found, it looks in the parent component’s injector, and so on, traversing up the component tree until it reaches the root injector.
- This hierarchical system allows for more fine-grained control over dependencies and enables sharing of services at different levels of the application.
3. Benefits of Angular Dependency Injection
3.1. Simplified Code
Dependency Injection promotes a cleaner and more organized codebase. Components and services become focused on their specific tasks, making the code more modular and easier to understand. The separation of concerns allows developers to focus on individual parts of the application without worrying about the intricacies of dependency management.
3.2. Reusability and Modularity
By having components depend on services and other components through interfaces rather than concrete implementations, it becomes easier to reuse them in different parts of the application. This modularity enables better code sharing, leading to faster development and easier maintenance.
3.3. Testability and Mocking
One of the significant advantages of Dependency Injection is its positive impact on unit testing. With external dependencies injected, it becomes straightforward to mock those dependencies during testing, allowing for isolated unit tests. This level of testability ensures that changes or updates to one part of the application are less likely to break other parts.
4. Providers and Services
4.1. Creating Services
In Angular, services are a common use case for dependency injection. A service is a class that typically encapsulates reusable functionality or data that multiple components might need. To create a service, use the @Injectable decorator, as shown earlier.
typescript @Injectable({ providedIn: 'root', }) export class DataService { // Service implementation here }
4.2. Providing Services
Once a service is created, it needs to be registered with Angular’s DI system to make it available for injection. This is done through the providers array in either the root module or the component’s decorator.
typescript import { NgModule } from '@angular/core'; import { DataService } from './data.service'; @NgModule({ providers: [DataService], }) export class AppModule { // Module configuration here }
In this example, we are providing the DataService at the root level, which means it will be available throughout the application.
4.3. Tree-shakable Providers
Angular allows for tree-shakable providers, which are providers that can be safely removed by the build optimizer if not used. This helps in reducing the application’s bundle size by eliminating unnecessary code.
To mark a provider as tree-shakable, use the providedIn property with the ‘any’ value when defining the @Injectable decorator.
typescript @Injectable({ providedIn: 'any', }) export class TreeShakableService { // Service implementation here }
5. Advanced Dependency Injection Techniques
5.1. Optional Dependencies
In some cases, a class may have optional dependencies that are not crucial for its functionality. Angular allows for optional dependencies using the @Optional decorator.
typescript @Injectable({ providedIn: 'root', }) export class LoggerService { constructor(@Optional() private analyticsService: AnalyticsService) { // Constructor logic here } }
In this example, the LoggerService has an optional dependency on AnalyticsService. If the AnalyticsService is not provided, the logger service will still function without any errors.
5.2. Factory Providers
Factory providers are used when we need to customize the creation of a dependency, rather than letting Angular create it using the constructor. This is useful when the creation logic is complex or requires additional configuration.
typescript import { Injectable } from '@angular/core'; @Injectable({ providedIn: 'root', useFactory: (dependencyService) => { return new ComplexService(dependencyService); }, deps: [DependencyService], }) export class ComplexService { // Service implementation here }
In this example, we define a factory function for the ComplexService that takes DependencyService as a parameter and returns a new instance of ComplexService with the provided dependency.
5.3. Injection Tokens
Injection tokens are used to customize how dependencies are resolved in specific scenarios. Unlike regular classes, injection tokens can be used as keys for the DI system.
typescript import { InjectionToken } from '@angular/core';
export const API_BASE_URL = new InjectionToken<string>(‘apiBaseUrl’);
In this example, we define an injection token API_BASE_URL, which can be used to provide a base URL for API calls.
typescript @NgModule({ providers: [{ provide: API_BASE_URL, useValue: 'https://api.example.com' }], }) export class AppModule { // Module configuration here }
We can provide a value for the injection token in the module or component decorator, as shown above.
Conclusion
Angular’s Dependency Injection is a powerful feature that greatly enhances the maintainability, testability, and reusability of your application’s code. By decoupling components and services from their dependencies, you can build modular and scalable applications with ease. Understanding the concepts and techniques of Angular DI is essential for any serious Angular developer, and with this knowledge, you can take your web development skills to the next level. Happy coding!
Whether you are a beginner or an experienced developer, learning and mastering Angular Dependency Injection will undoubtedly prove beneficial throughout your development journey. The ability to efficiently manage dependencies empowers you to build more robust, scalable, and testable applications, making you a more effective and proficient developer in the long run. So, start exploring Angular’s Dependency Injection today and unlock the full potential of your web applications.
Table of Contents
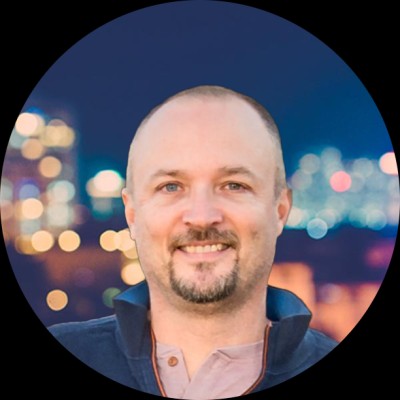
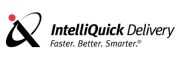