Angular and Material Design: Creating Modern User Interfaces
Creating a user interface that is both modern and functional is essential in today’s web development landscape. Angular, a robust front-end framework, paired with Material Design, Google’s design language, allows developers to craft beautiful and intuitive user interfaces. This article explores how to leverage Angular and Material Design to build sleek, responsive UIs with practical examples.
Understanding Angular and Material Design
Angular is a popular framework for building single-page applications (SPAs) with TypeScript, offering powerful tools for developing dynamic and robust applications. Material Design, on the other hand, is a design system that emphasizes consistency, visual feedback, and adaptive layouts. Integrating these two technologies enables developers to create interfaces that are not only aesthetically pleasing but also highly functional.
Setting Up Angular with Material Design
Before diving into building components, it’s essential to set up an Angular project with Material Design. Here’s a step-by-step guide to getting started.
1. Installing Angular Material
To begin, you’ll need to install Angular Material in your project:
```bash ng add @angular/material ```
This command will prompt you to choose a theme, set up global typography, and configure animations. Once the installation is complete, Angular Material will be integrated into your project.
2. Importing Material Modules
After installation, you need to import the necessary Material modules into your Angular application. Here’s an example of importing a few common modules:
```typescript import { NgModule } from '@angular/core'; import { MatButtonModule } from '@angular/material/button'; import { MatInputModule } from '@angular/material/input'; import { MatCardModule } from '@angular/material/card'; @NgModule({ imports: [ MatButtonModule, MatInputModule, MatCardModule ], exports: [ MatButtonModule, MatInputModule, MatCardModule ] }) export class MaterialModule {} ```
You can then include this `MaterialModule` in your main application module to make these Material components available throughout your app.
Creating a Modern UI with Angular Material
With Angular Material set up, you can start building modern, responsive UIs. Below are some examples of how to use Material Design components to create a polished interface.
1. Building a Responsive Navigation Bar
A well-designed navigation bar is crucial for user experience. Here’s how you can create one using Angular Material’s toolbar and button components:
```html <mat-toolbar color="primary"> <span>My App</span> <span class="spacer"></span> <button mat-button>Home</button> <button mat-button>About</button> <button mat-button>Contact</button> </mat-toolbar> ```
In this example, `mat-toolbar` is used to create a responsive navigation bar with buttons for different sections of the application.
2. Designing a Card-Based Layout
Cards are a fundamental component in Material Design, offering a flexible way to display content. Here’s an example of using Material cards to create a layout:
```html <div class="card-container"> <mat-card> <mat-card-header> <mat-card-title>Card Title</mat-card-title> <mat-card-subtitle>Card Subtitle</mat-card-subtitle> </mat-card-header> <mat-card-content> <p>This is some content inside a Material card.</p> </mat-card-content> </mat-card> </div> ```
You can style the `card-container` using CSS to ensure the cards are displayed in a grid or flex layout, depending on your design requirements.
3. Adding Forms with Angular Material
Forms are integral to most applications. Angular Material provides components like input fields, checkboxes, and radio buttons that follow Material Design guidelines. Here’s an example of a simple form:
```html <form class="example-form"> <mat-form-field> <mat-label>Name</mat-label> <input matInput placeholder="Enter your name"> </mat-form-field> <mat-form-field> <mat-label>Email</mat-label> <input matInput placeholder="Enter your email"> </mat-form-field> <button mat-raised-button color="primary">Submit</button> </form> ```
This form leverages `mat-form-field` for structured input fields and a `mat-raised-button` for a call-to-action button.
5. Enhancing UI/UX with Angular Animations
Animations can significantly enhance the user experience by providing visual feedback and guiding users through interactions. Angular’s animation module works seamlessly with Material Design components to create smooth and engaging transitions.
Example: Adding a Slide Animation to a Menu
```typescript import { trigger, state, style, transition, animate } from '@angular/animations'; @Component({ selector: 'app-menu', templateUrl: './menu.component.html', animations: [ trigger('slideInOut', [ state('in', style({ transform: 'translateX(0)' })), state('out', style({ transform: 'translateX(-100%)' })), transition('in => out', animate('300ms ease-in-out')), transition('out => in', animate('300ms ease-in-out')) ]) ] }) export class MenuComponent { menuState = 'out'; toggleMenu() { this.menuState = this.menuState === 'out' ? 'in' : 'out'; } } ```
This example demonstrates how to implement a sliding menu using Angular’s animation module. The `slideInOut` animation is triggered by the component’s `menuState`, resulting in a smooth sliding effect when the menu is toggled.
Conclusion
By combining Angular with Material Design, developers can create modern, responsive, and visually appealing user interfaces that adhere to best practices in UI/UX design. With the powerful components and customization options provided by Angular Material, building sleek, functional interfaces becomes more straightforward and efficient. Start integrating Material Design into your Angular projects today to elevate your application’s user experience.
Further Reading:
Table of Contents
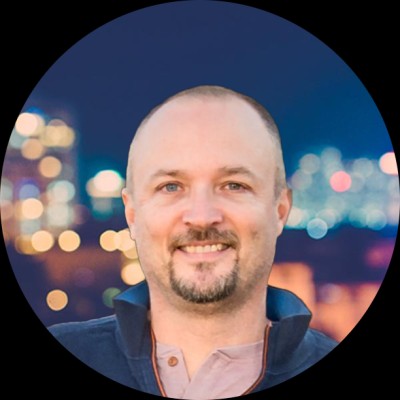
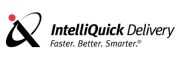