Using Angular Material for Beautiful UI Designs
In the world of web development, user interface (UI) design plays a pivotal role in determining the success of a website or web application. A visually appealing and user-friendly UI can significantly enhance the overall user experience, leading to higher engagement and retention rates. One of the most effective ways to achieve beautiful UI designs in Angular applications is by leveraging Angular Material – a powerful UI component library that seamlessly integrates with Angular projects. In this blog, we’ll explore the benefits of using Angular Material and dive into its key components, styling options, and code samples to create stunning UI designs.
Table of Contents
1. Why Choose Angular Material:
Angular Material, developed by the Angular team, is a UI component library built specifically for Angular applications. It follows the Material Design principles introduced by Google, providing a clean and intuitive UI with a focus on simplicity and functionality. By using Angular Material, developers can save valuable time and effort in creating custom UI components from scratch, as the library comes with a rich set of pre-designed, responsive, and customizable components. Here are some of the key reasons why you should consider using Angular Material for your UI designs:
- Ready-to-Use Components: Angular Material offers a wide range of UI components, including buttons, cards, dialogs, tabs, and more. These components are well-tested and optimized for performance, ensuring a consistent and polished appearance across different platforms and devices.
- Responsive Design: With the increasing usage of mobile devices, responsive design has become crucial for modern web applications. Angular Material components are designed to be responsive, adapting seamlessly to various screen sizes, making your application look stunning on any device.
- Theming and Customization: Angular Material provides a powerful theming system that allows you to customize the appearance of your application with ease. You can define your color palettes, typography, and even create custom themes to match your brand identity.
- Accessibility: Accessibility is a critical aspect of UI design, ensuring that all users, including those with disabilities, can interact with your application. Angular Material takes accessibility seriously, making it easier for developers to create accessible web apps.
- Active Community Support: Being an official Angular project, Angular Material enjoys strong community support. You can find plenty of resources, tutorials, and code samples online, making it easier to get started and resolve issues.
2. Getting Started with Angular Material:
Now that we understand the benefits of using Angular Material, let’s dive into the process of getting started with it in your Angular project.
Step 1: Create a New Angular Project:
If you don’t have an existing Angular project, you can create one using the Angular CLI. Open your terminal or command prompt and run the following command:
bash ng new my-angular-app cd my-angular-app
This will create a new Angular project named “my-angular-app” and navigate you into the project directory.
Step 2: Install Angular Material and Angular CDK:
To use Angular Material in your project, you need to install the necessary packages. Angular Material depends on Angular CDK (Component Dev Kit), so you’ll need to install both packages using npm:
bash npm install @angular/material @angular/cdk
Step 3: Import Angular Material Modules:
Once the installation is complete, you need to import the required Angular Material modules in your app module (usually “app.module.ts”). This makes the Material components available for use in your application.
typescript // app.module.ts import { NgModule } from '@angular/core'; import { BrowserModule } from '@angular/platform-browser'; import { BrowserAnimationsModule } from '@angular/platform-browser/animations'; import { MatButtonModule } from '@angular/material/button'; import { MatCardModule } from '@angular/material/card'; // Import other modules as per your requirement @NgModule({ declarations: [AppComponent], imports: [ BrowserModule, BrowserAnimationsModule, MatButtonModule, MatCardModule, // Add other modules to imports array ], providers: [], bootstrap: [AppComponent] }) export class AppModule { }
Step 4: Include Material Icons:
Many Angular Material components come with built-in Material Icons support. To use them, add the following link to your index.html file:
html <!-- index.html --> <link href="https://fonts.googleapis.com/icon?family=Material+Icons" rel="stylesheet">
3. Using Angular Material Components:
With Angular Material successfully integrated into your Angular project, let’s explore some of the popular Material components and how to use them effectively to create a beautiful UI design.
3.1. Buttons:
Buttons are fundamental elements in any user interface. Angular Material provides various types of buttons that can be easily customized to match your application’s theme.
html <!-- app.component.html --> <button mat-raised-button>Click me!</button> <button mat-icon-button><mat-icon>favorite</mat-icon></button>
In this example, we used mat-raised-button to create a raised button and mat-icon-button to create an icon button. The mat-icon element inside the icon button displays the “favorite” icon from the Material Icons library.
3.2. Cards:
Cards are versatile components used to display information in a structured manner. They can contain text, images, buttons, and other components.
html <!-- app.component.html --> <mat-card> <mat-card-header> <mat-card-title>Angular Material Card</mat-card-title> <mat-card-subtitle>Subtitle</mat-card-subtitle> </mat-card-header> <img mat-card-image src="your-image-url.jpg" alt="Image"> <mat-card-content> Some text content goes here. </mat-card-content> <mat-card-actions> <button mat-button>Learn More</button> <button mat-button>Buy Now</button> </mat-card-actions> </mat-card>
In this example, we used the mat-card component to create a card with a header, image, content, and actions. Replace your-image-url.jpg with the actual URL of the image you want to display.
3.3. Dialogs:
Dialogs are used to present information or receive user input in a modal popup. They are perfect for displaying alerts, confirmations, or custom forms.
typescript // app.component.ts import { Component } from '@angular/core'; import { MatDialog } from '@angular/material/dialog'; import { DialogComponent } from './dialog.component'; @Component({ selector: 'app-root', template: ` <button mat-button (click)="openDialog()">Open Dialog</button> ` }) export class AppComponent { constructor(private dialog: MatDialog) { } openDialog() { this.dialog.open(DialogComponent, { width: '300px', data: { message: 'Hello, this is a dialog!' } }); } } typescript Copy code // dialog.component.ts import { Component, Inject } from '@angular/core'; import { MAT_DIALOG_DATA } from '@angular/material/dialog'; @Component({ selector: 'app-dialog', template: ` <h2 mat-dialog-title>Dialog Title</h2> <mat-dialog-content> {{ data.message }} </mat-dialog-content> <mat-dialog-actions> <button mat-button mat-dialog-close>Close</button> </mat-dialog-actions> ` }) export class DialogComponent { constructor(@Inject(MAT_DIALOG_DATA) public data: any) { } }
In this example, we created a simple dialog with the help of Angular Material’s MatDialog. The dialog displays a message passed as data and has a “Close” button to dismiss it.
Conclusion:
Angular Material is a powerful and feature-rich UI component library that can significantly enhance the design and user experience of your Angular applications. By using its pre-designed components, customization options, and theming capabilities, you can create stunning and visually appealing user interfaces. This blog only scratched the surface of what Angular Material can offer, so I encourage you to explore the official documentation and experiment with various components to take full advantage of this fantastic library. Happy coding!
Table of Contents
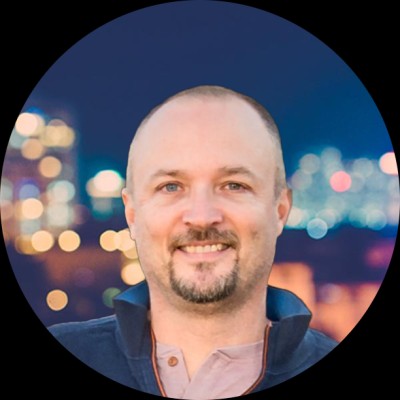
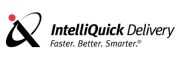