Building Microfrontends with Angular: Modular Architecture
In the world of web development, the pursuit of scalability, maintainability, and reusability is a constant challenge. As web applications grow in complexity, so does the need for a structured architecture that enables seamless development and maintenance. One approach that has gained prominence in recent years is microfrontends, a technique that involves breaking down a monolithic frontend application into smaller, more manageable parts. In this blog post, we will explore the concept of microfrontends and delve into how Angular, a popular JavaScript framework, can be used to build modular microfrontends.
Table of Contents
1. Understanding Microfrontends
1.1. What Are Microfrontends?
Microfrontends are a software architectural style that extends the microservices concept to the frontend layer of an application. In traditional monolithic frontend development, a single codebase handles the entire user interface. However, as applications grow, this monolithic approach becomes less maintainable and scalable. Microfrontends address these issues by breaking down the frontend into smaller, self-contained units.
Each microfrontend is responsible for a specific part of the user interface. For example, in an e-commerce application, you might have separate microfrontends for the product catalog, shopping cart, user profile, and checkout process. These microfrontends can be developed, tested, and deployed independently, allowing for faster development cycles and easier maintenance.
1.2. Benefits of Microfrontends
- Scalability: Microfrontends enable teams to work on smaller, focused components of an application, which can be easier to scale horizontally as traffic and demand increase.
- Independent Development: Different teams or developers can work on separate microfrontends simultaneously without interfering with each other’s work, fostering parallel development.
- Reusability: Microfrontends can be reused across multiple applications, promoting consistency in user interfaces and reducing development time.
- Fault Isolation: If one microfrontend encounters an issue or fails, it doesn’t necessarily bring down the entire application, enhancing fault tolerance.
- Technology Diversity: Teams can choose the most suitable technology stack for each microfrontend, allowing for flexibility and innovation.
Now that we understand the concept of microfrontends and their advantages, let’s explore how Angular fits into this modular architecture.
2. The Role of Angular in Microfrontends
2.1. Angular as a Powerful Frontend Framework
Angular is a robust and feature-rich JavaScript framework commonly used for building dynamic web applications. Its structured architecture, dependency injection system, and two-way data binding make it an excellent choice for developing large-scale frontend applications.
2.1.1. Key Features of Angular:
- Component-Based: Angular applications are built using components, which are self-contained units that encapsulate the user interface and behavior.
- Modular: Angular encourages the use of modules to organize code into smaller, reusable pieces, aligning well with the microfrontend approach.
- Dependency Injection: Angular’s dependency injection system promotes the separation of concerns and enables efficient testing and maintenance.
- Two-Way Data Binding: Angular’s data binding capabilities simplify the synchronization of data between the model and view.
2.2. Angular Modules: The Building Blocks
Angular modules are fundamental building blocks for creating modular applications. They group related components, directives, services, and other code into cohesive units. When it comes to microfrontends, Angular modules play a crucial role in maintaining separation and encapsulation.
To illustrate, let’s create a simple Angular module for a microfrontend responsible for displaying product listings:
typescript // product-list.module.ts import { NgModule } from '@angular/core'; import { CommonModule } from '@angular/common'; import { ProductListComponent } from './product-list.component'; @NgModule({ declarations: [ProductListComponent], imports: [CommonModule], exports: [ProductListComponent], }) export class ProductListModule {}
In this example, we define a module named ProductListModule that declares the ProductListComponent. We also import CommonModule for common Angular directives and export the component for use in other modules. This encapsulation ensures that the product listing microfrontend remains self-contained and isolated from other parts of the application.
3. Building Modular Microfrontends with Angular
3.1. Creating Independent Angular Applications
To build modular microfrontends with Angular, you should start by creating independent Angular applications for each microfrontend. Each application will have its own Angular module, components, services, and routing configuration.
Let’s create a basic structure for a product catalog microfrontend:
plaintext /product-catalog ??? src ? ??? app ? ? ??? product-catalog.module.ts ? ? ??? components ? ? ? ??? product-list.component.ts ? ? ? ??? product-detail.component.ts ? ? ??? services ? ? ? ??? product.service.ts ? ? ??? ... ??? …
In this structure, the product-catalog directory represents the standalone Angular application responsible for the product catalog microfrontend. It contains modules, components, services, and other assets specific to the microfrontend.
3.2. Sharing Components and Services
While microfrontends should be independent, there will be cases where you need to share components and services across multiple microfrontends to ensure consistency and reduce duplication.
3.3. Component Sharing
Angular provides a mechanism for sharing components through Angular libraries. You can create a library that includes common components and distribute it to your microfrontends.
bash ng generate library shared-components
After creating the library, you can develop and export components within it. For example, a shared product card component:
typescript // shared-components/src/lib/product-card/product-card.component.ts import { Component, Input } from '@angular/core'; @Component({ selector: 'app-product-card', templateUrl: './product-card.component.html', styleUrls: ['./product-card.component.css'], }) export class ProductCardComponent { @Input() product: Product; }
In this way, you can reuse the ProductCardComponent in multiple microfrontends by importing the shared-components library.
3.4. Service Sharing
Sharing services between microfrontends can be achieved through Angular’s dependency injection system. You can create a shared service in a library and provide it at the application level.
typescript // shared-services/src/lib/cart/cart.service.ts import { Injectable } from '@angular/core'; @Injectable({ providedIn: 'root', }) export class CartService { // ... }
By providing the service at the root level (providedIn: ‘root’), Angular ensures that a single instance of the service is available throughout the application, making it accessible to all microfrontends.
4. Routing in Microfrontends
4.1. Angular Router and Microfrontends
Routing is a fundamental aspect of any web application, and microfrontends are no exception. Angular provides a powerful routing mechanism that allows you to define routes and navigate between views seamlessly.
Each microfrontend can have its own routing configuration, enabling isolated navigation within the microfrontend itself. However, for a cohesive user experience, you may also need to implement cross-microfrontend navigation.
4.2. Cross-Microfrontend Navigation
Cross-microfrontend navigation involves navigating from one microfrontend to another within the same application. To achieve this, you can use Angular’s Router and RouterModule to define routes that link to different microfrontends.
typescript // app-routing.module.ts import { NgModule } from '@angular/core'; import { RouterModule, Routes } from '@angular/router'; const routes: Routes = [ { path: 'catalog', loadChildren: () => import('./product-catalog/product-catalog.module').then(m => m.ProductCatalogModule) }, { path: 'cart', loadChildren: () => import('./shopping-cart/shopping-cart.module').then(m => m.ShoppingCartModule) }, // ... ]; @NgModule({ imports: [RouterModule.forRoot(routes)], exports: [RouterModule], }) export class AppRoutingModule {}
In this example, we define routes for the product catalog and shopping cart microfrontends. When a user navigates to the /catalog or /cart paths, Angular loads the respective microfrontends lazily using the loadChildren method.
5. State Management
5.1. State Management in Microfrontends
Efficient state management is crucial for maintaining a consistent user experience in microfrontends. Different microfrontends might need to share data or synchronize their states to provide a seamless user experience.
5.2. Using NgRx for State Management
NgRx is a state management library for Angular that implements the Redux pattern. It provides a predictable and centralized way to manage application state, making it a suitable choice for microfrontends.
To use NgRx in a microfrontend, you can create a store for each microfrontend and define actions, reducers, and selectors specific to that microfrontend’s state.
typescript // product-catalog/store/product.actions.ts import { createAction, props } from '@ngrx/store'; import { Product } from '../models/product.model'; export const addToCart = createAction( '[Product Catalog] Add to Cart', props<{ product: Product }>() );
In this example, we define an action for adding a product to the cart within the product catalog microfrontend.
By employing NgRx in each microfrontend, you can manage and share state in a structured and maintainable way.
6. Deployment Strategies
6.1. Deploying Microfrontends
Deploying microfrontends can be challenging due to the need to manage multiple independent applications. However, various deployment strategies can help streamline the process.
6.2. Single-Server Deployment
In a single-server deployment, all microfrontends are hosted on the same server or domain. This approach simplifies deployment but may lead to increased complexity in managing dependencies and versioning.
6.3. Containerization and Orchestration
Containerization technologies like Docker and container orchestration platforms like Kubernetes can be used to deploy microfrontends as separate containers. This approach offers scalability and isolation while ensuring that each microfrontend is independently deployable.
6.4. CDN-Based Deployment
Content Delivery Networks (CDNs) can be leveraged to host microfrontends, distributing them globally for improved performance. CDNs can cache assets, reducing load times for users.
6.5. Serverless Deployment
Serverless computing platforms like AWS Lambda or Azure Functions can be used to deploy microfrontends as serverless functions. This approach offers cost-efficiency and scalability, as resources are allocated on-demand.
6.6. Hybrid Deployment
In a hybrid deployment, you can combine multiple deployment strategies to suit the specific needs of each microfrontend. For example, critical microfrontends may be containerized and deployed on Kubernetes, while less critical ones are hosted on a CDN.
6.7. Managing Versioning and Dependencies
Managing versions and dependencies is critical in a microfrontend architecture. Each microfrontend may have its own set of dependencies and version requirements. Tools like package managers and dependency resolution strategies are essential for ensuring compatibility and consistency.
7. Challenges and Considerations
7.1. Integration Challenges
Integrating microfrontends into a cohesive user experience can be challenging. You need to consider issues such as cross-microfrontend communication, sharing user authentication, and maintaining a consistent look and feel.
7.2. Maintaining Consistency
Maintaining a consistent user interface and user experience across all microfrontends is essential for a seamless user journey. Style guides, design systems, and UI component libraries can help achieve this consistency.
Conclusion
Building microfrontends with Angular and a modular architecture can significantly improve the scalability, maintainability, and reusability of your web applications. By breaking down your frontend into smaller, independent units, you empower development teams to work efficiently, embrace diverse technology stacks, and provide a better user experience. While there are challenges to overcome, the benefits of microfrontends make them a compelling architectural choice for modern web development.
In this blog post, we’ve explored the fundamentals of microfrontends, the role of Angular in building them, and key considerations for successful implementation. With the right approach and tools, you can embark on a journey toward creating modular, maintainable, and scalable web applications using microfrontends with Angular.
Table of Contents
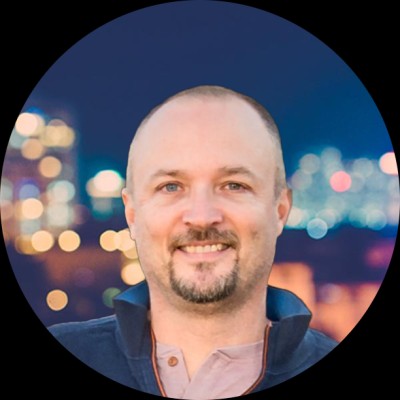
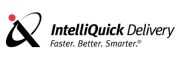