Angular and Microservices: Building Scalable Applications
In today’s fast-paced digital landscape, scalability and flexibility are crucial for building modern web applications. Combining Angular with a microservices architecture allows developers to create scalable, resilient applications that can adapt to changing requirements. This blog explores how Angular and microservices can be effectively integrated to build scalable applications, complete with practical examples and best practices.
Understanding Microservices Architecture
Microservices architecture involves breaking down a large application into smaller, independent services that communicate over a network. Each microservice is responsible for a specific piece of functionality, making the application easier to scale, maintain, and develop.
Benefits of Microservices:
– Scalability: Each service can be scaled independently.
– Flexibility: Services can be developed, deployed, and updated separately.
– Resilience: Failures in one service don’t necessarily affect others.
Integrating Angular with Microservices
Angular is a powerful framework for building dynamic, single-page applications (SPAs). When combined with a microservices architecture, Angular can act as the frontend layer that interacts with various backend microservices. This allows developers to create feature-rich, modular, and scalable web applications.
Key Considerations:
– API Gateway: Acts as an entry point for frontend requests to backend microservices.
– Inter-Service Communication: Manages communication between Angular and different microservices.
– Security: Implementing proper authentication and authorization between Angular and backend services.
1. Setting Up an Angular Application
The first step in building a scalable application with Angular and microservices is setting up the Angular project. Angular CLI simplifies this process by providing tools to create, build, and manage Angular applications.
```bash ng new angular-microservices-app cd angular-microservices-app ng serve ```
2. Communicating with Microservices
Angular communicates with backend microservices via HTTP requests. The `HttpClient` module in Angular simplifies this interaction, allowing developers to make API calls and handle responses.
Example: Consuming a User Microservice
Assume you have a microservice that manages user data. Here’s how Angular can interact with it:
```typescript import { HttpClient } from '@angular/common/http'; import { Injectable } from '@angular/core'; import { Observable } from 'rxjs'; @Injectable({ providedIn: 'root' }) export class UserService { private apiUrl = 'https://api.yourservice.com/users'; constructor(private http: HttpClient) {} getUsers(): Observable<User[]> { return this.http.get<User[]>(this.apiUrl); } } ```
3. Implementing Authentication and Authorization
In a microservices architecture, each service may have its own authentication and authorization mechanisms. Angular can handle these using JWT (JSON Web Tokens) and secure HTTP requests.
Example: Adding JWT Authentication
```typescript import { HttpClient, HttpHeaders } from '@angular/common/http'; import { Injectable } from '@angular/core'; import { Observable } from 'rxjs'; @Injectable({ providedIn: 'root' }) export class AuthService { private loginUrl = 'https://api.yourservice.com/auth/login'; constructor(private http: HttpClient) {} login(credentials: {username: string, password: string}): Observable<any> { return this.http.post(this.loginUrl, credentials, { headers: new HttpHeaders({ 'Content-Type': 'application/json' }) }); } getToken(): string { return localStorage.getItem('token') || ''; } } ```
4. Load Balancing and API Gateway
An API Gateway can manage traffic between Angular and the microservices. It can also handle load balancing, request routing, and security, ensuring that the Angular frontend communicates efficiently with the microservices.
Example: Configuring NGINX as an API Gateway
An example NGINX configuration for routing requests to the appropriate microservices:
```nginx http { upstream user_service { server user-service-host:8080; } upstream order_service { server order-service-host:8081; } server { listen 80; location /api/users/ { proxy_pass http://user_service; } location /api/orders/ { proxy_pass http://order_service; } } } ```
5. Deploying the Angular Application
After developing the Angular application, it must be deployed alongside the microservices. Tools like Docker and Kubernetes can help in containerizing and orchestrating the Angular app and microservices, ensuring scalability and resilience.
Example: Dockerizing the Angular Application
Here’s a simple Dockerfile to containerize your Angular application:
```dockerfile # Stage 1: Build the Angular application FROM node:14 AS build WORKDIR /app COPY . . RUN npm install RUN npm run build --prod # Stage 2: Serve the application FROM nginx:alpine COPY --from=build /app/dist/angular-microservices-app /usr/share/nginx/html EXPOSE 80 CMD ["nginx", "-g", "daemon off;"] ```
Conclusion
Combining Angular with a microservices architecture empowers developers to build scalable, maintainable, and resilient applications. By leveraging Angular’s robust framework alongside the flexibility of microservices, you can develop applications that are both performant and adaptable to future needs.
Further Reading:
Table of Contents
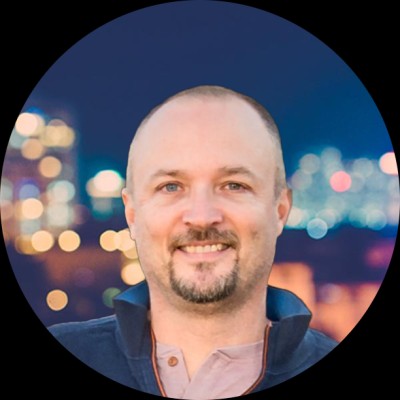
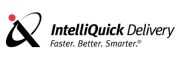