Building Offline-Capable Apps with Angular and IndexedDB
In today’s digital age, users expect applications to work seamlessly, whether they have an internet connection or not. Building offline-capable apps is crucial for providing a consistent and reliable user experience. Modern web applications face a challenge: providing a consistent user experience regardless of network connectivity. Users expect apps to be responsive and functional, even when they are offline or have a spotty internet connection. To meet this demand, developers must build offline-capable applications.
Table of Contents
Angular, a powerful and widely used front-end framework, can be combined with IndexedDB, a client-side database, to create applications that work seamlessly offline. In this blog, we will guide you through the process of building offline-capable apps with Angular and IndexedDB.
1. Understanding the Importance of Offline-Capable Apps
Before diving into the technical aspects, let’s understand why offline capability is essential for modern applications.
1.1. Enhanced User Experience
Offline-capable apps provide users with a smoother and more enjoyable experience. Instead of showing error messages when the internet connection is lost, these apps continue to function seamlessly.
1.2. Increased Accessibility
Offline capability makes applications more accessible to users in areas with limited or unreliable internet access. It caters to a broader audience, potentially increasing your app’s reach.
1.3. Data Integrity
Offline-capable apps can ensure data integrity by allowing users to work with their data even when offline. This reduces the risk of data loss due to network interruptions.
2. Setting Up the Angular Environment
To start building an offline-capable Angular app, you’ll need to set up your development environment. If you haven’t already, install Node.js and the Angular CLI. Here are the steps:
- Install Node.js: Visit the official Node.js website and download the installer for your operating system. Follow the installation instructions.
- Install Angular CLI: Open your terminal or command prompt and run the following command to install Angular CLI globally:
bash npm install -g @angular/cli
- Create a New Angular Project: Once Angular CLI is installed, you can create a new Angular project using the following command:
bash ng new offline-app
- Follow the prompts to configure your project. Once the project is created, navigate to the project directory:
bash cd offline-app
Now, you have a basic Angular project set up and ready to integrate offline capabilities using IndexedDB.
3. Working with IndexedDB
IndexedDB is a low-level API for storing significant amounts of structured data on the client side. It allows you to store, retrieve, and manipulate data even when the user is offline. Let’s explore the steps to work with IndexedDB in your Angular app.
3.1. Creating a Database
To get started with IndexedDB, you need to create a database. You can do this by creating a service in your Angular app that handles database initialization. Here’s a simplified example of how you can create a database:
typescript import { Injectable } from '@angular/core'; @Injectable({ providedIn: 'root' }) export class IndexedDbService { private dbName = 'myOfflineAppDB'; private dbVersion = 1; private db: IDBDatabase; constructor() { this.initDatabase(); } private initDatabase() { const request = window.indexedDB.open(this.dbName, this.dbVersion); request.onupgradeneeded = (event: any) => { this.db = event.target.result; // Create object stores and set up your database schema here }; request.onsuccess = (event: any) => { this.db = event.target.result; // Database is successfully opened and ready to use }; request.onerror = (event: any) => { console.error('Error opening database:', event.target.error); }; } }
In this code, we create an IndexedDbService that initializes the IndexedDB database. You can customize the database name, version, and schema according to your app’s requirements.
3.2. Defining Object Stores
IndexedDB uses object stores to store data. Object stores are similar to tables in a traditional relational database. You can define object stores to organize and store different types of data in your database. Here’s an example of defining an object store for storing user data:
typescript private initDatabase() { const request = window.indexedDB.open(this.dbName, this.dbVersion); request.onupgradeneeded = (event: any) => { this.db = event.target.result; // Create an object store for users if (!this.db.objectStoreNames.contains('users')) { this.db.createObjectStore('users', { keyPath: 'id', autoIncrement: true }); } // Add more object stores as needed }; // ... }
In this example, we create an object store named ‘users’ and specify that it should use the ‘id’ property as the key and auto-increment it.
3.3. Performing CRUD Operations
Now that you have defined your object stores, you can perform CRUD (Create, Read, Update, Delete) operations on your data. Here’s how you can add a user to the ‘users’ object store:
typescript async addUser(user: User): Promise<number> { const transaction = this.db.transaction(['users'], 'readwrite'); const objectStore = transaction.objectStore('users'); return new Promise((resolve, reject) => { const request = objectStore.add(user); request.onsuccess = (event: any) => { const userId = event.target.result; resolve(userId); }; request.onerror = (event: any) => { console.error('Error adding user:', event.target.error); reject(event.target.error); }; }); }
This code creates a transaction, accesses the ‘users’ object store, and adds a user to it. Similar methods can be created for reading, updating, and deleting data.
4. Building an Angular App with Offline Capabilities
Now that you have set up IndexedDB and learned how to work with it, it’s time to integrate offline capabilities into your Angular app.
4.1. Designing the UI
Before implementing offline capabilities, design your app’s user interface. Ensure that the UI clearly communicates whether the app is in online or offline mode. You can use Angular components and templates for this purpose.
Here’s an example of a component that displays a message based on the app’s online status:
typescript import { Component, OnInit } from '@angular/core'; import { NetworkService } from './network.service'; @Component({ selector: 'app-root', template: ` <div> <h1>Offline-Capable App</h1> <p>{{ onlineMessage }}</p> </div> ` }) export class AppComponent implements OnInit { onlineMessage: string; constructor(private networkService: NetworkService) {} ngOnInit() { this.onlineMessage = this.networkService.isOnline() ? 'Online' : 'Offline'; } }
In this example, the NetworkService can be used to check the online status of the app.
4.2. Handling Data Synchronization
Synchronizing data between the client and server is a critical aspect of offline-capable apps. You’ll need to implement logic to sync data when the app goes online. This typically involves making HTTP requests to the server to update or retrieve data.
typescript import { Injectable } from '@angular/core'; import { HttpClient } from '@angular/common/http'; @Injectable({ providedIn: 'root' }) export class DataService { private apiUrl = 'https://api.example.com'; constructor(private http: HttpClient) {} // Implement methods for syncing data with the server }
The DataService class can have methods for syncing data with the server when the app is online.
4.3. Implementing Offline Mode
To make your app fully offline-capable, you need to implement offline mode handling. This means allowing users to interact with the app and perform operations even when there’s no internet connection. You can achieve this by utilizing the data stored in IndexedDB.
Here’s an example of a service that retrieves user data from IndexedDB and displays it in the UI when offline:
typescript import { Injectable } from '@angular/core'; import { IndexedDbService } from './indexed-db.service'; @Injectable({ providedIn: 'root' }) export class OfflineDataService { constructor(private indexedDbService: IndexedDbService) {} async getUsersOffline(): Promise<User[]> { const transaction = this.indexedDbService.db.transaction(['users'], 'readonly'); const objectStore = transaction.objectStore('users'); return new Promise((resolve, reject) => { const request = objectStore.getAll(); request.onsuccess = (event: any) => { const users = event.target.result; resolve(users); }; request.onerror = (event: any) => { console.error('Error retrieving users:', event.target.error); reject(event.target.error); }; }); } }
In this code, the OfflineDataService retrieves user data from the ‘users’ object store in IndexedDB and returns it for display in the UI when the app is offline.
5. Testing and Debugging
Testing your offline-capable Angular app is crucial to ensure that it functions as expected in various network conditions. Use tools like Angular’s testing utilities and browser developer tools to debug and fine-tune your app’s offline capabilities.
6. Deploying Your Offline-Capable Angular App
Once you have built and thoroughly tested your offline-capable Angular app, it’s time to deploy it to a web server. You can use hosting platforms like Netlify, Vercel, or GitHub Pages to make your app accessible to users.
Conclusion
Building offline-capable apps with Angular and IndexedDB is a powerful way to provide a seamless user experience, regardless of network connectivity. By following the steps outlined in this blog, you can create applications that work reliably even when offline, enhancing user satisfaction and accessibility. Offline capability is not just a feature but a necessity in today’s digital landscape, and Angular combined with IndexedDB makes it more achievable than ever. Start building your offline-capable app today and watch it thrive in a world of varying network conditions.
Table of Contents
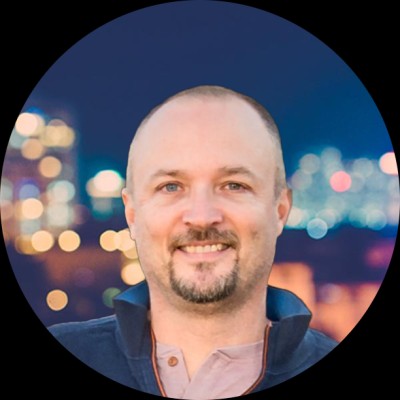
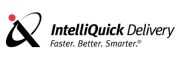