Exploring Angular Reactive Forms: Advanced Form Handling
In the world of web development, forms play a crucial role in collecting data from users. Angular, being a robust and popular framework, provides two types of forms: Template-driven forms and Reactive forms. While both approaches have their merits, Reactive Forms are preferred for complex scenarios and advanced form handling due to their flexibility, testability, and ease of implementation. In this blog, we will explore Angular Reactive Forms and delve into their advanced features, including validations, dynamic forms, form arrays, and more.
Table of Contents
1. Introduction to Angular Reactive Forms
Angular Reactive Forms are a powerful way to manage form inputs in Angular applications. Unlike template-driven forms, Reactive Forms are based on a reactive programming paradigm, where form control values are bound to underlying data streams. This enables seamless integration with other reactive operators and allows for advanced form handling scenarios.
Reactive Forms provide a FormGroup class that represents a collection of form controls and tracks the status and validity of the group. Each form control is an instance of the FormControl class, which manages the value and validation of an individual form field.
2. Setting Up an Angular Project
Before we dive into Reactive Forms, let’s set up a new Angular project if you don’t have one already:
bash # Install Angular CLI globally (skip this step if you have it already) npm install -g @angular/cli # Create a new Angular project ng new angular-reactive-forms-demo cd angular-reactive-forms-demo
3. Creating a Basic Reactive Form
Let’s start by creating a simple form with a few input fields:
- First Name
- Last Name
In your Angular component file (e.g., app.component.ts), import the necessary modules and define the form:
typescript import { Component } from '@angular/core'; import { FormBuilder, FormGroup, Validators } from '@angular/forms'; @Component({ selector: 'app-root', templateUrl: './app.component.html', styleUrls: ['./app.component.css'] }) export class AppComponent { userForm: FormGroup; constructor(private fb: FormBuilder) {} ngOnInit() { this.userForm = this.fb.group({ firstName: ['', Validators.required], lastName: ['', Validators.required], email: ['', [Validators.required, Validators.email]], }); } onSubmit() { if (this.userForm.valid) { // Form processing logic goes here console.log(this.userForm.value); } } }
In the code above, we have created a FormGroup called userForm, representing the entire form. Inside the FormGroup, we define three FormControl instances for firstName, lastName, and email, each with its respective validation rules.
4. Form Validation
Form validation is a crucial aspect of any form handling mechanism. Angular Reactive Forms provide various built-in validators that we can use to validate user inputs. Additionally, we can create custom validators to suit specific validation requirements.
4.1 Built-in Validators
Let’s take a look at some of the commonly used built-in validators:
4.1.1 Required Validator:
The Validators.required validator ensures that a form field is not empty:
typescript // Inside the component class this.userForm = this.fb.group({ firstName: ['', Validators.required], // ... });
4.1.2 Email Validator:
The Validators.email validator checks whether the input value is a valid email address:
typescript // Inside the component class this.userForm = this.fb.group({ // ... email: ['', [Validators.required, Validators.email]], });
4.1.3 Min/Max Length Validators:
The Validators.minLength and Validators.maxLength validators enforce a minimum and maximum length for the input:
typescript // Inside the component class this.userForm = this.fb.group({ // ... firstName: ['', [Validators.required, Validators.minLength(2), Validators.maxLength(50)]], });
4.2 Custom Validators
In addition to the built-in validators, you can create custom validators to perform more specific validations. Custom validators are functions that receive a FormControl instance as an argument and return an object with validation errors if the condition is not met.
typescript // Inside a separate validator file (e.g., custom-validators.ts) import { AbstractControl, ValidationErrors } from '@angular/forms'; export function customValidator(control: AbstractControl): ValidationErrors | null { // Your validation logic goes here // Return an object with a validation error or null if valid return control.value === 'example' ? { forbiddenValue: true } : null; }
To use the custom validator, import it into your component and add it to the FormControl’s validators:
typescript // Inside the component class import { customValidator } from './custom-validators'; // ... this.userForm = this.fb.group({ // ... someField: ['', customValidator], });
5. Dynamic Forms
Sometimes, your form requirements may not be static, and you need to generate form fields dynamically based on user interactions or external data sources. Reactive Forms allow you to create dynamic forms with ease.
Let’s consider an example where we want to create a form that captures a list of addresses with fields for street address, city, and postal code. Users can add or remove address fields as needed.
typescript // Inside the component class import { FormArray } from '@angular/forms'; // ... this.userForm = this.fb.group({ // ... addresses: this.fb.array([this.createAddress()]), }); get addresses() { return this.userForm.get('addresses') as FormArray; } createAddress() { return this.fb.group({ street: ['', Validators.required], city: ['', Validators.required], postalCode: ['', Validators.required], }); } addAddress() { this.addresses.push(this.createAddress()); } removeAddress(index: number) { this.addresses.removeAt(index); }
In the code snippet above, we define a FormArray named addresses to hold multiple address form groups. The createAddress() method returns a FormGroup with fields for street, city, and postal code. We provide a method addAddress() to add a new address field dynamically and a method removeAddress() to remove an address field when no longer needed.
In your template, you can use the *ngFor directive to display the dynamic form fields:
html <!-- Inside the component's template --> <form [formGroup]="userForm" (ngSubmit)="onSubmit()"> <!-- ... Other form fields ... --> <div formArrayName="addresses"> <div *ngFor="let address of addresses.controls; let i = index" [formGroupName]="i"> <input formControlName="street" placeholder="Street"> <input formControlName="city" placeholder="City"> <input formControlName="postalCode" placeholder="Postal Code"> <button type="button" (click)="removeAddress(i)">Remove Address</button> </div> <button type="button" (click)="addAddress()">Add Address</button> </div> <button type="submit" [disabled]="userForm.invalid">Submit</button> </form>
6. Form Arrays
Angular Reactive Forms also provide support for handling form arrays. Form arrays are an excellent choice when you want to manage a dynamic list of form controls, such as a list of email addresses, phone numbers, or any other repetitive form elements.
Let’s create a form that captures multiple email addresses:
typescript // Inside the component class import { FormArray } from '@angular/forms'; // ... this.userForm = this.fb.group({ // ... emailList: this.fb.array([]), }); get emailList() { return this.userForm.get('emailList') as FormArray; } addEmail() { this.emailList.push(this.fb.control('', [Validators.required, Validators.email])); } removeEmail(index: number) { this.emailList.removeAt(index); }
In the above code, we define a FormArray called emailList, which stores the email addresses provided by the user. We create the addEmail() method to add new email fields dynamically and the removeEmail() method to remove email fields as needed.
In your template, you can use the same *ngFor directive to display the form array:
html <!-- Inside the component's template --> <form [formGroup]="userForm" (ngSubmit)="onSubmit()"> <!-- ... Other form fields ... --> <div formArrayName="emailList"> <div *ngFor="let email of emailList.controls; let i = index"> <input [formControl]="email" placeholder="Email"> <button type="button" (click)="removeEmail(i)">Remove Email</button> </div> <button type="button" (click)="addEmail()">Add Email</button> </div> <button type="submit" [disabled]="userForm.invalid">Submit</button> </form>
7. Working with Nested Forms
In some scenarios, you may encounter forms that contain nested form groups or form arrays. For instance, consider a scenario where you need to collect information about multiple users, and each user has its set of properties, such as name, age, and hobbies.
Let’s create a form that handles information about multiple users with nested form groups:
typescript // Inside the component class this.userForm = this.fb.group({ // ... users: this.fb.array([this.createUserInfo()]), }); get users() { return this.userForm.get('users') as FormArray; } createUserInfo() { return this.fb.group({ name: ['', Validators.required], age: ['', Validators.required], hobbies: this.fb.array([]), }); } addUser() { this.users.push(this.createUserInfo()); } removeUser(index: number) { this.users.removeAt(index); }
In the code above, we create a FormArray named users, where each element of the array represents the information of an individual user. Each user is represented as a FormGroup containing name, age, and a FormArray hobbies. The addUser() and removeUser() methods allow users to be added or removed dynamically.
In your template, you can handle the nested form groups similarly to how we did with dynamic forms and form arrays:
html <!-- Inside the component's template --> <form [formGroup]="userForm" (ngSubmit)="onSubmit()"> <!-- ... Other form fields ... --> <div formArrayName="users"> <div *ngFor="let user of users.controls; let i = index" [formGroupName]="i"> <input formControlName="name" placeholder="Name"> <input formControlName="age" placeholder="Age"> <div formArrayName="hobbies"> <div *ngFor="let hobby of user.get('hobbies').controls; let j = index"> <input [formControl]="hobby" placeholder="Hobby"> <button type="button" (click)="removeHobby(i, j)">Remove Hobby</button> </div> <button type="button" (click)="addHobby(i)">Add Hobby</button> </div> <button type="button" (click)="removeUser(i)">Remove User</button> </div> <button type="button" (click)="addUser()">Add User</button> </div> <button type="submit" [disabled]="userForm.invalid">Submit</button> </form>
8. Handling Form Submission
Once the user fills out the form and clicks the submit button, you’ll want to handle the form submission. In our example, we’ve implemented an onSubmit() method inside the component class to process the form data.
typescript // Inside the component class onSubmit() { if (this.userForm.valid) { // Perform form submission logic here console.log(this.userForm.value); } }
The this.userForm.value contains the entire form data in JSON format, which you can then send to your server or perform any other necessary actions.
Conclusion
Angular Reactive Forms provide a robust and flexible way to handle forms in your Angular applications. We have explored various features of Reactive Forms, such as form validation, dynamic forms, form arrays, and working with nested forms. By leveraging these advanced form handling capabilities, you can build sophisticated and user-friendly forms that meet the requirements of even the most complex scenarios.
Whether you are building a simple contact form or a multi-step registration form, Angular Reactive Forms will empower you to create interactive and dynamic user interfaces with ease. As you continue to explore Angular and its features, you’ll discover even more possibilities to enhance your web applications and deliver outstanding user experiences.
With that, we encourage you to put your newfound knowledge into practice and start building your next Angular application with Reactive Forms. Happy coding!
Table of Contents
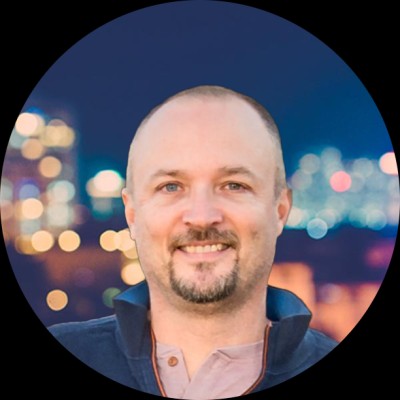
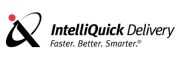