Angular and Reactive Programming: Handling Asynchronous Operations
In the fast-paced world of web development, it is essential to create responsive and interactive user interfaces that handle data efficiently. Asynchronous operations play a crucial role in achieving this goal by allowing applications to execute tasks concurrently without blocking the user interface. However, managing asynchronous code can be challenging, leading to callback hell and code that is difficult to maintain.
Table of Contents
This is where Angular and Reactive Programming come to the rescue. Angular is a popular JavaScript framework for building dynamic and scalable web applications, while Reactive Programming is a paradigm that provides elegant solutions for dealing with asynchronous operations. In this blog, we will introduce you to both Angular and Reactive Programming and demonstrate how they work together to handle asynchronous tasks effectively.
1. What is Angular?
Angular is a powerful, open-source web application framework developed and maintained by Google. It allows developers to build dynamic, single-page applications (SPAs) with ease. Angular follows the Model-View-Controller (MVC) architectural pattern, where the application’s logic, user interface, and data are separated into distinct components, making the code more organized and maintainable.
1.1. Key Features of Angular:
- Two-way data binding: Changes in the data automatically update the user interface and vice versa.
- Dependency Injection: Helps manage and inject dependencies into components, making testing and reusability easier.
- Directives: Extends HTML with custom attributes and tags to build reusable components.
- Services: Provides a way to organize and share code across multiple components.
2. Introduction to Reactive Programming
Reactive Programming is a paradigm that enables developers to handle asynchronous operations using a declarative approach. It focuses on data streams and propagates changes through the application whenever the data changes, allowing for a more responsive and scalable system. The core building block of Reactive Programming is the concept of “Observables.”
Observables represent data streams that can emit multiple values over time. These values can be of any type, including primitive data, objects, or even events. Observables can be created from various data sources, such as user input events, HTTP requests, or even custom streams.
Subscribers are the consumers of these observables. They “subscribe” to an observable to receive notifications whenever new data is emitted. Subscribers can then react to these changes and perform the necessary actions.
Operators are the methods used to manipulate the data emitted by observables. Operators allow developers to transform, filter, combine, or otherwise modify the data stream before it reaches the subscribers.
The combination of these three elements—Observables, Subscribers, and Operators—forms the basis of Reactive Programming.
3. The Role of Reactive Programming in Angular
Angular leverages the power of Reactive Programming to handle asynchronous tasks seamlessly. By adopting the Reactive Programming approach, Angular applications can easily manage various asynchronous operations, such as HTTP requests, user input, and real-time data streams.
Using Reactive Programming in Angular offers several benefits:
- Simplified Asynchronous Code: Reactive Programming simplifies the way asynchronous tasks are handled. It replaces the traditional callback-based approach with a more intuitive and concise syntax, making the code easier to read and maintain.
- Consistent Data Flow: With Reactive Programming, data flow becomes more predictable. It follows a unidirectional pattern, where data flows from the source (observable) to the subscribers, avoiding unexpected side effects.
- Error Handling: Reactive Programming provides robust error-handling mechanisms, allowing developers to handle errors gracefully and prevent application crashes.
- Memory Management: Angular’s built-in memory management system ensures that subscriptions are automatically cleaned up when they are no longer needed, preventing memory leaks.
By embracing Reactive Programming, Angular enhances the overall performance and stability of applications that involve complex asynchronous operations.
4. Key Concepts of Reactive Programming
Before diving deeper into Angular and Reactive Programming integration, let’s explore the fundamental concepts of Reactive Programming:
4.1. Observables
In Reactive Programming, Observables are the sources of data streams. They represent asynchronous data streams that can emit zero or more values over time. Observables can emit various types of data, including numbers, strings, arrays, objects, or even events.
To create an observable, you can use the Observable class from the popular ReactiveX library, which is widely used in the JavaScript ecosystem. Let’s see an example of creating a simple observable that emits three values:
typescript import { Observable } from 'rxjs'; const simpleObservable = new Observable((observer) => { observer.next('Hello'); observer.next('World'); observer.next('!'); }); // Subscribe to the observable simpleObservable.subscribe((value) => console.log(value)); // Output: // Hello // World // !
In this example, we create an observable named simpleObservable that emits three values: ‘Hello’, ‘World’, and ‘!’. We then subscribe to this observable and log each emitted value to the console.
4.2. Subscribers
Subscribers are the consumers of observables. They subscribe to an observable to receive notifications whenever new data is emitted. Subscribers are defined using the subscribe method on an observable.
Let’s extend the previous example and add a subscriber to the simpleObservable:
typescript // ... (previous code) // Subscriber const simpleSubscriber = simpleObservable.subscribe((value) => console.log(value)); // Output: // Hello // World // ! // Unsubscribe after some time setTimeout(() => { simpleSubscriber.unsubscribe(); }, 2000);
In this modified example, we create a subscriber named simpleSubscriber, which listens to the simpleObservable. After subscribing, the subscriber receives and logs the emitted values as before. However, we also set a timeout of 2000 milliseconds to unsubscribe the subscriber after two seconds. This demonstrates how to clean up subscriptions and avoid unnecessary resource consumption.
4.3. Operators
Operators are the key to transforming and manipulating data emitted by observables. They allow you to modify the data stream before it reaches the subscribers. There are numerous built-in operators available in the ReactiveX library, and you can also create custom operators if needed.
Let’s consider an example where we use the map operator to transform the emitted values to uppercase:
typescript import { Observable } from 'rxjs'; import { map } from 'rxjs/operators'; const uppercaseObservable = new Observable((observer) => { observer.next('hello'); observer.next('world'); }); uppercaseObservable .pipe( map((value: string) => value.toUpperCase()) ) .subscribe((value) => console.log(value)); // Output: // HELLO // WORLD
In this example, we use the map operator to convert the emitted values to uppercase. As a result, the output will display the transformed values.
Reactive Programming’s Observables, Subscribers, and Operators work in harmony to handle asynchronous operations in a clean and efficient manner. Now that we understand the core concepts of Reactive Programming, let’s see how to integrate it into an Angular project.
5. Getting Started with Angular and Reactive Programming
To get started with Angular and Reactive Programming, you need to set up an Angular project and integrate the necessary libraries. Let’s go through the steps:
5.1. Setting Up an Angular Project
Before proceeding, ensure you have Node.js and npm (Node Package Manager) installed on your machine. With these prerequisites in place, you can use the Angular CLI (Command-Line Interface) to create a new Angular project:
bash $ npm install -g @angular/cli # Install Angular CLI globally (if not already installed) $ ng new my-angular-app # Create a new Angular project $ cd my-angular-app # Navigate to the project folder
The above commands will create a new Angular project named my-angular-app. Next, navigate to the project folder using the cd command.
5.2. Integrating Reactive Programming
To use Reactive Programming in Angular, we need to install the ReactiveX library (rxjs) and include it in our project. Thankfully, Angular already includes rxjs as a dependency, so there’s no need to install it separately.
The rxjs library provides all the necessary tools for working with observables, subscribers, and operators. Angular uses rxjs to handle various asynchronous operations, such as HTTP requests and form handling.
With the Angular project set up and rxjs integrated, we are ready to handle asynchronous operations efficiently using Angular and Reactive Programming.
6. Handling Asynchronous Operations with Angular and Reactive Programming
Let’s explore some common scenarios where Angular and Reactive Programming shine when dealing with asynchronous tasks.
6.1. Asynchronous HTTP Requests
Fetching data from a server is a common asynchronous operation in web applications. Angular makes it easy to handle HTTP requests using the built-in HttpClient module. Combining Angular’s HttpClient with Reactive Programming allows us to manage HTTP requests elegantly.
Consider the following example where we fetch data from a sample REST API and display it on the page:
typescript import { Component, OnInit } from '@angular/core'; import { HttpClient } from '@angular/common/http'; import { Observable } from 'rxjs'; interface Todo { id: number; title: string; completed: boolean; } @Component({ selector: 'app-todo-list', template: ` <ul> <li *ngFor="let todo of todos$ | async">{{ todo.title }}</li> </ul> `, }) export class TodoListComponent implements OnInit { todos$: Observable<Todo[]>; constructor(private http: HttpClient) {} ngOnInit() { this.todos$ = this.http.get<Todo[]>('https://jsonplaceholder.typicode.com/todos'); } }
In this example, we import the necessary modules from Angular and rxjs. The HttpClient is used to make an HTTP GET request to the JSONPlaceholder API, which provides sample data for testing. We define the Todo interface to represent the structure of the received data.
In the component class, we declare an Observable named todos$ to hold the data stream. In the ngOnInit method, we assign the result of the HTTP request to the todos$ observable. The data is fetched asynchronously, and Angular’s async pipe is used in the template to subscribe to the observable and automatically update the view whenever new data is available.
6.2. Reactive Forms
Forms are an essential part of web applications, and handling form input asynchronously is a common requirement. Angular’s Reactive Forms module, combined with Reactive Programming, simplifies form handling and validation.
Let’s create a simple login form with Angular’s Reactive Forms:
typescript import { Component, OnInit } from '@angular/core'; import { FormBuilder, FormGroup, Validators } from '@angular/forms'; @Component({ selector: 'app-login', template: ` <form [formGroup]="loginForm" (ngSubmit)="onSubmit()"> <input type="text" formControlName="username" placeholder="Username"> <input type="password" formControlName="password" placeholder="Password"> <button type="submit" [disabled]="loginForm.invalid">Login</button> </form> `, }) export class LoginComponent implements OnInit { loginForm: FormGroup; constructor(private fb: FormBuilder) {} ngOnInit() { this.loginForm = this.fb.group({ username: ['', Validators.required], password: ['', Validators.required], }); } onSubmit() { if (this.loginForm.valid) { // Perform login operation } } }
In this example, we import the necessary modules from Angular, including the FormBuilder and FormGroup classes. The loginForm is created using the FormBuilder, and we define two form controls for the username and password fields, both with required validators.
The template binds the form controls to the corresponding input fields using the formControlName directive. The login button is disabled if the form is invalid.
Angular’s Reactive Forms module automatically handles form validation and tracks changes in the form controls using Reactive Programming. As a result, we can easily check the form’s validity and perform the login operation only when the form data is valid.
6.3. Real-time Data Streams
Real-time data streams, such as WebSocket connections, are becoming more common in modern web applications. With Reactive Programming, handling real-time data becomes more manageable.
Let’s create a simple real-time data display using Angular and WebSocket:
typescript import { Component, OnInit, OnDestroy } from '@angular/core'; import { webSocket, WebSocketSubject } from 'rxjs/webSocket'; @Component({ selector: 'app-real-time-data', template: ` <div *ngIf="data">{{ data }}</div> `, }) export class RealTimeDataComponent implements OnInit, OnDestroy { data: any; private webSocketSubject: WebSocketSubject<any>; ngOnInit() { this.webSocketSubject = webSocket('wss://my-websocket-server.com'); this.webSocketSubject.subscribe( (message) => (this.data = message), (error) => console.error('WebSocket error:', error), () => console.log('WebSocket connection closed.') ); } ngOnDestroy() { this.webSocketSubject.complete(); } }
In this example, we import the webSocket function from rxjs, which allows us to create a WebSocket subject and connect to a WebSocket server.
In the component class, we initialize the WebSocket connection inside the ngOnInit method, where we use webSocket(‘wss://my-websocket-server.com’) to connect to the WebSocket server at the specified URL.
We then subscribe to the WebSocketSubject and handle incoming messages using the subscription. The data property is updated with the received message, which triggers the view update automatically.
When the component is destroyed (in ngOnDestroy), we close the WebSocket connection using this.webSocketSubject.complete() to prevent memory leaks.
Conclusion
Angular and Reactive Programming are a powerful combination for handling asynchronous operations in modern web applications. The declarative approach of Reactive Programming simplifies the management of complex asynchronous code, making it easier to maintain and understand.
In this blog post, we explored the fundamentals of Angular, Reactive Programming, and how they work together. We covered key concepts like Observables, Subscribers, and Operators, and demonstrated how to handle asynchronous HTTP requests, form handling, and real-time data streams using Angular and Reactive Programming.
By mastering these concepts, you’ll be able to build more efficient, responsive, and scalable web applications, providing a seamless user experience. Embrace the power of Angular and Reactive Programming and take your web development skills to new heights!
Table of Contents
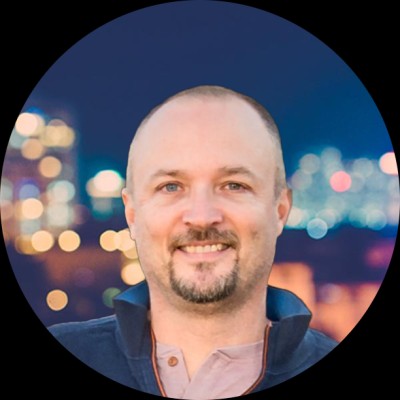
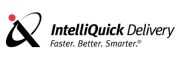