Building Real-Time Applications with Angular and WebSocket
In today’s fast-paced digital world, real-time communication and data exchange have become crucial components of modern web applications. Users expect instant updates and responses, making real-time functionality a must-have for many applications. One of the best ways to achieve real-time capabilities is by integrating WebSockets into your Angular applications. In this blog post, we will explore how to build real-time applications using Angular and WebSocket technology.
Table of Contents
1. Introduction to Real-Time Applications
Traditional web applications communicate with servers using HTTP, where the client sends a request to the server, and the server responds with the requested data. However, this request-response model is not suitable for real-time communication as it involves frequent polling or waiting for updates. WebSockets, on the other hand, provide a full-duplex communication channel that allows bi-directional, real-time communication between the client and the server.
2. Understanding WebSockets
2.1. What are WebSockets?
WebSockets are a protocol that enables real-time, two-way communication between a client and a server over a single, long-lived connection. Unlike HTTP, which is stateless and requires a new connection for every request, WebSockets maintain a continuous connection, enabling real-time data exchange.
2.2. WebSocket vs. HTTP
HTTP follows a request-response paradigm, where a client sends a request to the server, and the server responds with the data. This model is suitable for many applications, but it becomes inefficient for real-time scenarios where data needs to be pushed from the server to the client without waiting for a request.
WebSockets, on the other hand, offer a persistent connection that stays open, allowing data to be transmitted in real-time as soon as it’s available. This makes WebSockets much more efficient for real-time applications compared to traditional HTTP.
2.3. WebSocket Advantages
- Low Latency: WebSockets reduce latency by eliminating the need for repeated connections for each request.
- Bi-directional Communication: Both the client and server can send messages to each other at any time, making real-time collaboration possible.
- Efficient Data Transfer: WebSockets use a binary protocol, reducing overhead and making data transfer faster.
- Scalability: WebSocket connections are lightweight, allowing the server to handle a large number of concurrent connections.
3. Setting Up an Angular Project
Before we dive into implementing WebSockets, let’s set up an Angular project to work with. If you already have an Angular project, you can skip this step.
Code Sample – Creating a new Angular project
bash ng new real-time-app cd real-time-app
4. Implementing WebSocket in Angular
4.1. Installing WebSocket Package
To use WebSockets in our Angular application, we’ll need a WebSocket library. One popular choice is ngx-websocket, which provides a simple interface to work with WebSockets.
Code Sample – Installing ngx-websocket
bash npm install ngx-websocket --save
4.2. Creating a WebSocket Service
Next, let’s create a service to handle WebSocket connections and events.
Code Sample – Creating a WebSocket Service
typescript // websocket.service.ts import { Injectable } from '@angular/core'; import { WebSocketSubject } from 'rxjs/webSocket'; @Injectable({ providedIn: 'root', }) export class WebSocketService { private socket$: WebSocketSubject<any>; private readonly socketUrl = 'ws://your-websocket-url'; constructor() { this.socket$ = new WebSocketSubject(this.socketUrl); } public sendMessage(message: any): void { this.socket$.next(message); } public getMessages() { return this.socket$.asObservable(); } public closeConnection(): void { this.socket$.complete(); } }
4.3. Handling WebSocket Events
With the WebSocket service in place, we can now subscribe to WebSocket events to receive data from the server.
Code Sample – Subscribing to WebSocket events
typescript // app.component.ts import { Component, OnDestroy } from '@angular/core'; import { Subscription } from 'rxjs'; import { WebSocketService } from './websocket.service'; @Component({ selector: 'app-root', templateUrl: './app.component.html', styleUrls: ['./app.component.css'], }) export class AppComponent implements OnDestroy { private subscription: Subscription; constructor(private webSocketService: WebSocketService) { this.subscription = this.webSocketService.getMessages().subscribe( (message) => { // Handle incoming messages from the server }, (error) => { console.error('WebSocket error:', error); } ); } ngOnDestroy() { this.subscription.unsubscribe(); this.webSocketService.closeConnection(); } }
5. Building a Real-Time Chat Application
5.1. Designing the Chat Interface
Now that we have our WebSocket service ready, let’s build a real-time chat application using Angular. The chat interface will allow users to send and receive messages in real-time.
Code Sample – Chat Component Template
html <!-- chat.component.html --> <div class="chat-container"> <div class="chat-messages"> <div *ngFor="let message of messages"> <span [ngClass]="{'my-message': message.isMe}"> {{ message.sender }}: {{ message.text }} </span> </div> </div> <div class="chat-input"> <input type="text" placeholder="Type your message..." [(ngModel)]="newMessage" (keyup.enter)="sendMessage()" /> <button (click)="sendMessage()">Send</button> </div> </div>
Code Sample – Chat Component Styles
css /* chat.component.css */ .chat-container { display: flex; flex-direction: column; height: 100%; padding: 20px; } .chat-messages { flex: 1; overflow-y: auto; } .my-message { font-weight: bold; } .chat-input { display: flex; margin-top: 10px; } input { flex: 1; padding: 5px; } button { margin-left: 10px; }
5.2. Implementing Chat Functionality
In the Chat component, we’ll use the WebSocket service to send and receive messages.
Code Sample – Chat Component TypeScript
typescript // chat.component.ts import { Component } from '@angular/core'; import { WebSocketService } from '../websocket.service'; @Component({ selector: 'app-chat', templateUrl: './chat.component.html', styleUrls: ['./chat.component.css'], }) export class ChatComponent { messages: { sender: string; text: string; isMe: boolean }[] = []; newMessage: string = ''; constructor(private webSocketService: WebSocketService) { this.webSocketService.getMessages().subscribe((message: any) => { this.messages.push(message); }); } sendMessage(): void { if (this.newMessage.trim() !== '') { this.webSocketService.sendMessage({ sender: 'Me', text: this.newMessage, isMe: true, }); this.newMessage = ''; } } }
6. Real-Time Data Visualization
6.1. Using WebSocket for Live Data
WebSockets are not limited to just chat applications. They can also be used for real-time data visualization, such as live charts and graphs. Let’s explore how we can achieve this in our Angular app.
6.2. Creating Real-Time Charts
For the purpose of this demonstration, we’ll use the popular ngx-charts library to create real-time line charts.
Code Sample – Installing ngx-charts
bash npm install @swimlane/ngx-charts --save
Code Sample – Real-Time Line Chart Component
typescript // real-time-chart.component.ts import { Component, OnDestroy } from '@angular/core'; import { Subscription, interval } from 'rxjs'; import { WebSocketService } from '../websocket.service'; @Component({ selector: 'app-real-time-chart', template: ` <ngx-charts-line-chart [view]="view" [scheme]="colorScheme" [results]="chartData" [legend]="showLegend" [showXAxisLabel]="showXAxisLabel" [showYAxisLabel]="showYAxisLabel" [xAxis]="xAxisLabel" [yAxis]="yAxisLabel" > </ngx-charts-line-chart> `, }) export class RealTimeChartComponent implements OnDestroy { view: any[] = [700, 400]; colorScheme = { domain: ['#5AA454'], }; chartData: { name: string; value: number }[] = []; private subscription: Subscription; constructor(private webSocketService: WebSocketService) { this.subscription = interval(1000).subscribe(() => { const randomValue = Math.floor(Math.random() * 100); this.chartData.push({ name: new Date().toISOString(), value: randomValue }); }); this.webSocketService.getMessages().subscribe((message: any) => { // Assuming the server sends real-time data as a numeric value this.chartData.push({ name: new Date().toISOString(), value: message.data }); }); } ngOnDestroy() { this.subscription.unsubscribe(); } }
6.3. Using the Real-Time Line Chart Component
You can now use the RealTimeChartComponent in your application to display real-time data updates on a line chart.
html <!-- app.component.html --> <app-real-time-chart></app-real-time-chart>
7. Collaborative Whiteboard Application
7.1.Setting Up the Whiteboard
Another exciting real-time application we can build is a collaborative whiteboard. Multiple users can draw on the whiteboard simultaneously, and changes made by one user are instantly reflected on the screens of others. Let’s get started!
Code Sample – Whiteboard Component Template
html <!-- whiteboard.component.html --> <canvas #whiteboardCanvas (mousedown)="onMouseDown($event)" (mousemove)="onMouseMove($event)" (mouseup)="onMouseUp()" (mouseleave)="onMouseUp()" ></canvas> Code Sample - Whiteboard Component Styles css Copy code /* whiteboard.component.css */ canvas { border: 1px solid #ccc; cursor: crosshair; }
7.2. Sharing Whiteboard Data with WebSocket
To synchronize the drawing actions on the whiteboard, we’ll use WebSockets to broadcast the drawing data to all connected clients.
Code Sample – Whiteboard Component TypeScript
typescript // whiteboard.component.ts import { Component, ViewChild, ElementRef, AfterViewInit } from '@angular/core'; import { WebSocketService } from '../websocket.service'; @Component({ selector: 'app-whiteboard', templateUrl: './whiteboard.component.html', styleUrls: ['./whiteboard.component.css'], }) export class WhiteboardComponent implements AfterViewInit { @ViewChild('whiteboardCanvas', { static: false }) canvas: ElementRef<HTMLCanvasElement>; private ctx: CanvasRenderingContext2D; private isDrawing: boolean = false; private lastX: number; private lastY: number; constructor(private webSocketService: WebSocketService) {} ngAfterViewInit() { this.ctx = this.canvas.nativeElement.getContext('2d'); } onMouseDown(event: MouseEvent) { this.isDrawing = true; this.lastX = event.offsetX; this.lastY = event.offsetY; } onMouseMove(event: MouseEvent) { if (this.isDrawing) { const currentX = event.offsetX; const currentY = event.offsetY; this.draw(this.lastX, this.lastY, currentX, currentY); this.webSocketService.sendMessage({ type: 'draw', fromX: this.lastX, fromY: this.lastY, toX: currentX, toY: currentY, }); this.lastX = currentX; this.lastY = currentY; } } onMouseUp() { this.isDrawing = false; } private draw(fromX: number, fromY: number, toX: number, toY: number) { this.ctx.beginPath(); this.ctx.moveTo(fromX, fromY); this.ctx.lineTo(toX, toY); this.ctx.stroke(); this.ctx.closePath(); } private clearCanvas() { this.ctx.clearRect(0, 0, this.canvas.nativeElement.width, this.canvas.nativeElement.height); } }
7.3. Collaborative Whiteboard with WebSocket
Now that we have implemented the WhiteboardComponent, we can use it in our app to create a collaborative whiteboard.
html <!-- app.component.html --> <app-whiteboard></app-whiteboard>
8. Security Considerations
8.1. Securing WebSocket Connections
When implementing real-time applications, security is of utmost importance. By default, WebSockets use the ws:// protocol, which is not secure. To ensure secure communication, we should use the wss:// protocol, which encrypts the data transmitted between the client and the server.
To enable secure WebSocket connections, ensure that your server supports SSL/TLS and use the wss:// protocol instead of ws:// in your WebSocket URLs.
8.2. Authentication and Authorization
Depending on the nature of your real-time application, you may need to implement authentication and authorization mechanisms. Ensure that users are properly authenticated before allowing them to establish WebSocket connections. Additionally, apply appropriate authorization rules to control access to specific resources or functionalities within the application.
9. Performance Optimization
9.1. Scaling WebSocket Applications
As your real-time application gains popularity and the number of concurrent WebSocket connections increases, you may need to scale your application to handle the load effectively. Consider using load balancing and distributed architectures to distribute WebSocket connections across multiple servers.
9.2. Handling Concurrent Connections
To ensure optimal performance, carefully manage WebSocket connections and release resources when they are no longer needed. Also, handle potential errors gracefully to prevent connection drops and improve the overall user experience.
Conclusion
WebSockets offer a powerful mechanism for building real-time applications with Angular. By leveraging the WebSocket protocol, developers can achieve instant communication and data exchange between clients and servers, enabling the creation of interactive and collaborative applications.
In this blog post, we explored the fundamentals of WebSockets, setting up an Angular project, and implementing real-time features such as chat, live data visualization, and a collaborative whiteboard. We also discussed security considerations and performance optimization techniques for WebSocket-based applications.
As you continue your journey into real-time web development, consider exploring more advanced features and use cases for WebSockets to deliver even more engaging and dynamic experiences for your users.
Now, armed with the knowledge of Angular and WebSocket integration, it’s time to bring your real-time application ideas to life and revolutionize the way your users interact with your web applications!
Happy coding!
Table of Contents
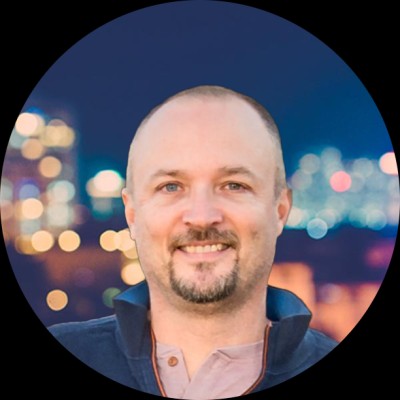
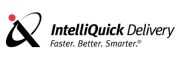