Exploring Angular Routing: A Comprehensive Guide to Navigating Single-Page Applications
Modern web applications often encompass numerous pages, views, and components. Itβs vital to manage these different segments effectively to offer a smooth and seamless user experience. This is where Angular routing plays a crucial role, a skill that is a must-have when you want to hire Angular developers. Angular routing provides an elegant solution to handle different views within your Single-Page Application (SPA). When executed correctly, it significantly improves the fluidity and responsiveness of your application, characteristics often brought to the table by expert Angular developers. In this blog post, we will discuss Angular routing and provide examples of how to use it effectively. This way, when you decide to hire Angular developers, you’ll have a good understanding of the core competencies to look for.
What is Angular Routing?
In a traditional web application, clicking a link or changing the URL often results in a new request to the server, which in turn reloads the entire page. This process can be inefficient and detrimental to the user experience.
Angular routing is a feature provided by the Angular framework, designed to simplify navigation through different components or views within a Single-Page Application. With Angular routing, changing the view doesn’t require a page reload, resulting in a smoother user experience.
In essence, Angular routing allows you to “swap” the content of a part of your application based on the URL or other conditions. This is achieved by mapping specific URLs to components, enabling the switching between these components without a full page refresh. The route configuration defines these mappings.
Setting Up Angular Routing
Before diving into examples, let’s start by setting up Angular routing in a new project.
- Create a new Angular project
First, we’ll create a new Angular project using Angular CLI. Open your terminal and type:
```bash ng new angular-routing-example ```
- Generate new components
For the purpose of this example, let’s create three components named ‘Home’, ‘About’, and ‘Contact’. We can use Angular CLI to create these components:
```bash ng generate component Home ng generate component About ng generate component Contact ```
- Set up routing
Next, we’ll set up routing for our application. Angular CLI can generate a routing module for us with the following command:
```bash ng generate module app-routing --flat --module=app ```
This command generates a file named `app-routing.module.ts` in the `src/app` folder and imports it in the `app.module.ts`.
Configuring Routes
Routes are configured in the `app-routing.module.ts` file. The routes are an array of JavaScript objects, each containing two properties:
– path: a string that matches the URL in the browser address bar.
– component: the component class that should be used to display the view.
Here’s how you define routes for the Home, About, and Contact components:
```typescript import { NgModule } from '@angular/core'; import { RouterModule, Routes } from '@angular/router'; import { HomeComponent } from './home/home.component'; import { AboutComponent } from './about/about.component'; import { ContactComponent } from './contact/contact.component'; const routes: Routes = [ { path: 'home', component: HomeComponent }, { path: 'about', component: AboutComponent }, { path: 'contact', component: ContactComponent }, ]; @NgModule({ imports: [RouterModule.forRoot(routes)], exports: [RouterModule] }) export class AppRoutingModule { } ```
In this configuration, when a user navigates to `’/home’`, the `HomeComponent` is displayed. The same goes for `’/about’` and `’/contact’`.
Linking to Routes
Angular provides the `<router-outlet>` directive where it will display the components. In your `app.component.html` file, include this directive:
```html <router-outlet></router-outlet> ```
To create links to these routes, Angular provides the `routerLink` directive. This can be used in the `app.component.html` as shown:
```html <a routerLink="/home">Home</a> <a routerLink="/about">About</a> <a routerLink="/contact">Contact</a> <router-outlet></router-outlet> ```
When you click on these links, the corresponding components will be displayed in the place of the `<router-outlet>`.
Route Parameters
Sometimes, you need to pass dynamic data via the route. For instance, you might have a User component, and you want to load user data based on the user ID in the URL.
Here’s how you define a route with a parameter:
```typescript { path: 'user/:id', component: UserComponent }, ```
In the User component, you can access this parameter using the `ActivatedRoute` service:
```typescript import { Component, OnInit } from '@angular/core'; import { ActivatedRoute } from '@angular/router'; @Component({ selector: 'app-user', templateUrl: './user.component.html', styleUrls: ['./user.component.css'] }) export class UserComponent implements OnInit { id: string; constructor(private route: ActivatedRoute) { } ngOnInit() { this.id = this.route.snapshot.paramMap.get('id'); } } ```
The `id` in the URL can be accessed with `this.route.snapshot.paramMap.get(‘id’)`. Now you can use this `id` to load user data from your backend.
Route Guards
Route guards are useful when you want to prevent users from accessing certain routes based on certain conditions. For instance, you might want to prevent unauthenticated users from accessing a certain route.
You can create a route guard using Angular CLI:
```bash ng generate guard auth ```
This will create an `auth.guard.ts` file. An example of an AuthGuard that always allows access looks like this:
```typescript import { Injectable } from '@angular/core'; import { CanActivate, ActivatedRouteSnapshot, RouterStateSnapshot, UrlTree } from '@angular/router'; import { Observable } from 'rxjs'; @Injectable({ providedIn: 'root' }) export class AuthGuard implements CanActivate { canActivate( route: ActivatedRouteSnapshot, state: RouterStateSnapshot): Observable<boolean | UrlTree> | Promise<boolean | UrlTree> | boolean | UrlTree { return true; } } ```
You can use this guard in your routes like this:
```typescript { path: 'protected', component: ProtectedComponent, canActivate: [AuthGuard] }, ```
The `canActivate` method in the guard determines if the route can be activated. If it returns `true`, navigation will continue. If `false`, navigation will be canceled.
Conclusion
Angular routing is a powerful feature that makes managing navigation in your Single-Page App a breeze. This proficiency is particularly important when you’re looking to hire Angular developers, as routing is a fundamental aspect of Angular application development. It provides a clean and intuitive way to manage different views, handle route parameters, and secure routes using route guards. With Angular routing, you can create complex applications with many views, while ensuring a smooth and seamless user experience – a skill set that expert Angular developers should excel at.
Mastering Angular routing is crucial for developing sophisticated Angular applications, which is a key factor to consider when you decide to hire Angular developers. They should be comfortable with experimenting and delving deeper into this powerful feature. The more practice and experience an Angular developer has with routing, the more capable they will be in unlocking a world of possibilities for your Angular applications.
Table of Contents
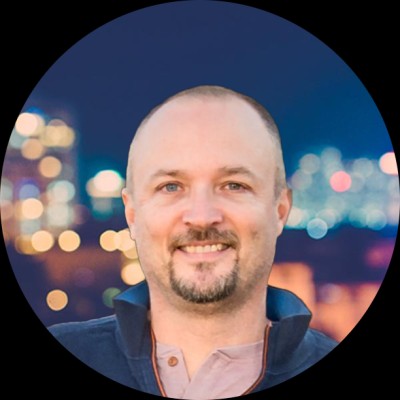
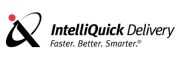