Exploring Angular Unit Testing: Frameworks and Best Practices
Angular, a popular JavaScript framework for building web applications, provides developers with a powerful toolset for creating dynamic and responsive apps. However, as your application grows in complexity, maintaining its stability becomes increasingly challenging. This is where unit testing comes into play. In this comprehensive guide, we will explore Angular unit testing, covering various testing frameworks and best practices to ensure your Angular applications are robust and bug-free.
Table of Contents
1. Why Unit Testing in Angular Matters
Before diving into the specifics of Angular unit testing, let’s understand why it’s crucial for modern web development.
1.1. Early Bug Detection
Unit testing allows you to catch bugs and issues early in the development process. By testing individual parts (units) of your code, you can identify problems before they propagate throughout your application.
1.2. Confidence in Refactoring
When you decide to refactor or make changes to your codebase, unit tests act as a safety net. They help ensure that your changes don’t introduce new issues, making the development process smoother and less error-prone.
1.3. Documentation
Unit tests serve as documentation for your code. They provide clear examples of how each component should behave, making it easier for other developers (including future you) to understand and work with your codebase.
1.4. Collaboration
Testing your code allows for easier collaboration among team members. When everyone understands how different parts of the application are supposed to function, it becomes simpler to work together on a project.
2. Unit Testing Frameworks for Angular
Angular offers several unit testing frameworks to choose from. Each framework has its own strengths and weaknesses, and your choice should align with your project’s requirements and your team’s preferences.
2.1. Jasmine
Jasmine is a popular behavior-driven development (BDD) testing framework for JavaScript. It’s often used with Angular for unit testing. Jasmine provides a clean syntax for writing tests and includes a wide range of matchers for assertions.
Here’s a simple example of a Jasmine test in an Angular component:
javascript describe('MyComponent', () => { it('should create', () => { const fixture = TestBed.createComponent(MyComponent); const component = fixture.componentInstance; expect(component).toBeTruthy(); }); });
In this example, we’re testing whether an instance of MyComponent can be created. Jasmine’s describe and it functions help organize tests, and the expect function is used for assertions.
2.2. Karma
Karma is a test runner for JavaScript that’s often used with Angular. It allows you to execute your tests in various browsers and provides a clear report of the results. Karma works seamlessly with Jasmine, making it a popular choice for Angular projects.
To set up Karma for your Angular project, you’ll typically use the Angular CLI, which simplifies the process. Here’s how you can run your tests using Karma:
bash ng test
Karma will launch the specified browsers and execute your tests, providing feedback on test pass/fail status.
2.3. Protractor
Protractor is an end-to-end (E2E) testing framework for Angular applications. While it’s primarily designed for E2E testing, it can also be used for unit testing. Protractor allows you to interact with your application as a user would, simulating user actions and testing how your components behave in real scenarios.
Here’s a simple Protractor test that clicks a button and checks if a specific element is displayed:
javascript it('should display a message after clicking a button', () => { const button = element(by.id('myButton')); button.click(); const message = element(by.id('message')); expect(message.isDisplayed()).toBeTruthy(); });
Protractor is particularly useful when you need to test complex user interactions and workflows within your Angular application.
2.4. Test Bed
The Angular Test Bed is a testing utility that comes built-in with the Angular framework. It provides a set of utilities for configuring and interacting with Angular components within your tests.
Here’s an example of using the Test Bed to create a test for an Angular component:
javascript import { ComponentFixture, TestBed } from '@angular/core/testing'; import { MyComponent } from './my.component'; describe('MyComponent', () => { let component: MyComponent; let fixture: ComponentFixture<MyComponent>; beforeEach(() => { TestBed.configureTestingModule({ declarations: [MyComponent], }); fixture = TestBed.createComponent(MyComponent); component = fixture.componentInstance; }); it('should create', () => { expect(component).toBeTruthy(); }); });
The Test Bed simplifies the process of setting up an Angular testing environment and is a valuable tool for unit testing Angular components.
3. Best Practices for Angular Unit Testing
To ensure effective unit testing in your Angular project, consider these best practices:
3.1. Isolate Dependencies
When writing unit tests, aim to isolate the component or service being tested from its dependencies. Use mock objects or stubs to simulate the behavior of dependencies, ensuring that tests focus solely on the unit under examination.
javascript // Example using a Jasmine spy to mock a service dependency const userServiceSpy = jasmine.createSpyObj('UserService', ['getUser']); beforeEach(() => { TestBed.configureTestingModule({ declarations: [MyComponent], providers: [{ provide: UserService, useValue: userServiceSpy }], }); });
3.2. Keep Tests Simple
Each unit test should focus on a single aspect of the component or service. Avoid testing multiple behaviors within a single test, as this can lead to unclear and brittle tests.
3.3. Test Edge Cases
Don’t just test the happy path—ensure that your tests cover edge cases and error scenarios. This helps uncover potential issues that might not be evident in regular usage.
3.4. Use Descriptive Test Names
Clear and descriptive test names make it easier to understand the purpose of each test. This aids in identifying failures and maintaining the test suite over time.
javascript it('should display an error message when the user is not found', () => { // Test logic here });
3.5. Continuous Integration (CI)
Integrate your unit tests into your CI/CD pipeline to automatically run tests on every code commit. This ensures that new changes don’t introduce regressions.
3.6. Code Coverage
Monitor code coverage to track which parts of your codebase are covered by tests. Aim for high code coverage to minimize the risk of untested code causing issues.
Conclusion
Unit testing is an essential practice for ensuring the reliability and maintainability of Angular applications. By choosing the right testing framework, following best practices, and integrating testing into your development workflow, you can build robust and bug-free Angular apps with confidence.
In this guide, we’ve explored some of the key testing frameworks available for Angular, including Jasmine, Karma, Protractor, and the Angular Test Bed. We’ve also discussed best practices to help you write effective unit tests.
Remember that unit testing is an ongoing process. As your application evolves, your tests should evolve with it. Regularly review and update your tests to keep pace with changes in your codebase.
By investing in unit testing, you’re not only enhancing the quality of your Angular applications but also making your development process more efficient and collaborative. Happy testing!
Table of Contents
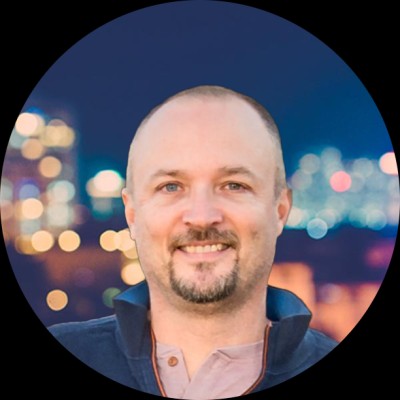
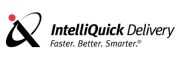