Angular and Web Accessibility: Building Inclusive Apps
Web accessibility ensures that digital content is usable by all individuals, including those with disabilities. By creating accessible applications, you can reach a broader audience and comply with legal requirements. Angular, a popular web development framework, offers robust tools and practices for building accessible apps.
Understanding Web Accessibility
Web accessibility involves designing and developing websites and applications so that people with disabilities can perceive, understand, navigate, and interact with them. Accessibility is crucial for providing equal access to information and functionality, regardless of users’ abilities.
Using Angular for Accessibility
Angular provides several features and techniques to help developers build accessible applications. Below are some key aspects and code examples demonstrating how Angular can be employed for enhancing accessibility.
-
Semantic HTML and ARIA Attributes
Semantic HTML is the foundation of web accessibility. It involves using HTML elements according to their intended purpose. Angular facilitates the use of semantic HTML by integrating seamlessly with standard HTML5 elements and attributes.
Example: Adding ARIA Attributes
ARIA (Accessible Rich Internet Applications) attributes enhance the accessibility of dynamic content. Here’s an example of using ARIA attributes in an Angular component.
```html <!-- Accessible button with ARIA role and label --> <button aria-label="Submit Form" (click)="submitForm()">Submit</button> <!-- Accessible modal dialog --> <div role="dialog" aria-labelledby="dialogTitle" aria-describedby="dialogDescription"> <h2 id="dialogTitle">Dialog Title</h2> <p id="dialogDescription">Dialog content goes here.</p> <button aria-label="Close" (click)="closeDialog()">Close</button> </div> ```
2. Keyboard Navigation
Ensuring that users can navigate your application using only a keyboard is a critical aspect of accessibility. Angular allows you to manage focus and handle keyboard events effectively.
Example: Managing Focus with Angular
You can use Angular’s `@ViewChild` decorator to control focus within components, enhancing keyboard navigation.
```typescript import { Component, ElementRef, ViewChild } from '@angular/core'; @Component({ selector: 'app-keyboard-nav', template: ` <button (click)="focusInput()">Focus Input</button> <input #textInput type="text" aria-label="Input Field" /> ` }) export class KeyboardNavComponent { @ViewChild('textInput') textInput!: ElementRef; focusInput() { this.textInput.nativeElement.focus(); } } ```
3. Color Contrast and Visual Accessibility
Maintaining sufficient color contrast between text and background is essential for users with visual impairments. Angular allows you to apply styles dynamically, ensuring your application meets contrast requirements.
Example: Dynamic Theme Adjustment
Using Angular, you can adjust the application’s theme to ensure adequate contrast, catering to users with visual disabilities.
```typescript import { Component } from '@angular/core'; @Component({ selector: 'app-theme-switcher', template: ` <button (click)="toggleTheme()">Toggle Theme</button> <div [ngClass]="currentTheme"> <p>This is some sample text.</p> </div> ` }) export class ThemeSwitcherComponent { currentTheme = 'light-theme'; toggleTheme() { this.currentTheme = this.currentTheme === 'light-theme' ? 'dark-theme' : 'light-theme'; } } ```
4. Accessible Forms
Forms are a crucial part of web applications, and ensuring their accessibility is vital. Angular provides tools like reactive forms that make it easier to create accessible form controls.
Example: Creating Accessible Form Controls
Here’s how you can build an accessible form using Angular’s reactive forms.
```typescript import { Component } from '@angular/core'; import { FormBuilder, FormGroup, Validators } from '@angular/forms'; @Component({ selector: 'app-accessible-form', template: ` <form [formGroup]="contactForm" (ngSubmit)="onSubmit()"> <label for="name">Name:</label> <input id="name" formControlName="name" aria-required="true" /> <label for="email">Email:</label> <input id="email" formControlName="email" aria-required="true" type="email" /> <button type="submit">Submit</button> </form> ` }) export class AccessibleFormComponent { contactForm: FormGroup; constructor(private fb: FormBuilder) { this.contactForm = this.fb.group({ name: ['', Validators.required], email: ['', [Validators.required, Validators.email]] }); } onSubmit() { if (this.contactForm.valid) { console.log('Form Submitted', this.contactForm.value); } } } ```
5. Screen Reader Compatibility
Screen readers are essential tools for users with visual impairments. Angular supports ARIA roles and attributes, which improve screen reader compatibility.
Example: Enhancing Screen Reader Experience
You can use ARIA roles and live regions to provide dynamic updates to screen readers.
```typescript import { Component } from '@angular/core'; @Component({ selector: 'app-live-region', template: ` <div aria-live="polite" aria-atomic="true"> {{ notification }} </div> <button (click)="sendNotification()">Send Notification</button> ` }) export class LiveRegionComponent { notification = 'Welcome to the application.'; sendNotification() { this.notification = 'You have a new message.'; } } ```
Conclusion
Building accessible applications is not only about compliance but also about inclusivity. Angular provides the tools and flexibility needed to create applications that are usable by everyone, regardless of their abilities. By implementing these best practices, you can ensure that your Angular applications are accessible, user-friendly, and inclusive.
Further Reading:
Table of Contents
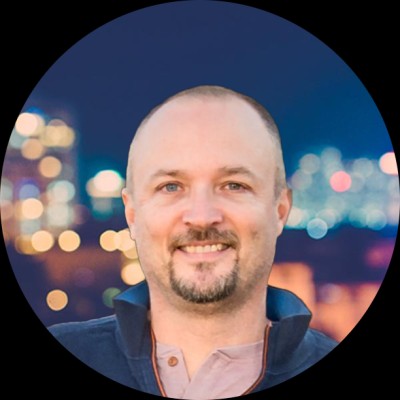
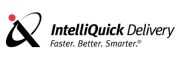