C# Q & A
How to use async and await in C#?
Using `async` and `await` in C# is a powerful way to write asynchronous code that doesn’t block the main thread and allows for responsive and efficient applications, especially when dealing with time-consuming tasks like I/O operations or network requests. Here’s a step-by-step guide on how to use `async` and `await` effectively:
- Mark Methods as `async`: To use `async` and `await`, start by marking your methods as `async`. This is done by adding the `async` keyword before the method signature. For example:
```csharp async Task MyAsyncMethod() { // Async code here } ```
- Use `await` for Asynchronous Operations: Inside an `async` method, you can use the `await` keyword before an asynchronous operation to pause the method’s execution until the awaited operation is completed. This ensures that your code remains non-blocking. For example:
```csharp async Task MyAsyncMethod() { string result = await SomeAsyncOperation(); // Continue with the result } ```
- Return Types: If an `async` method needs to return a value, you can specify the return type as `Task<T>` where `T` is the type of the result. If the method doesn’t return anything, you can use `Task` as the return type. For example:
```csharp async Task<string> GetStringAsync() { string result = await SomeAsyncOperation(); return result; } ```
- Exception Handling: Properly handle exceptions in your `async` methods. You can use `try-catch` blocks as you would in synchronous code to catch and handle exceptions that might occur during asynchronous operations.
- Async All the Way: When using `async` and `await`, it’s a good practice to keep the asynchrony throughout your call stack. If you’re calling an asynchronous method, make sure the caller method is also marked as `async`, and so on, to maintain the benefits of asynchrony.
- Avoid Blocking: Avoid using blocking operations (e.g., `.Result`, `.Wait()`) inside an `async` method, as they can lead to deadlocks. Instead, use `await` for asynchronous operations to ensure non-blocking behavior.
By following these guidelines, you can harness the power of `async` and `await` in C# to write responsive and efficient code that performs well in scenarios involving asynchronous operations, such as web requests, database queries, and I/O-bound tasks.
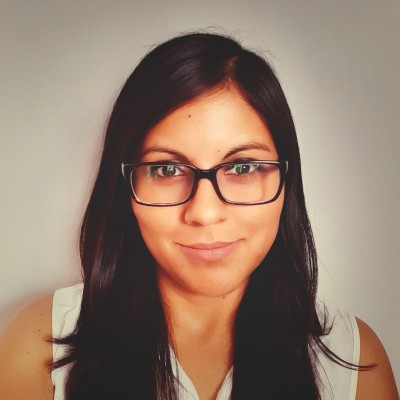
Previously at
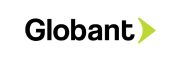
Experienced Backend Developer with 6 years of experience in C#. Proficient in C#, .NET, and Java.Proficient in REST web services and web app development.