How to configure services and dependencies in ASP.NET Core?
Configuring services and dependencies in ASP.NET Core is a fundamental step in setting up your application. This process involves registering and configuring services that your application relies on, making them available for use throughout the application. Here’s how to do it:
- Open the `Startup.cs` File:
– In your ASP.NET Core project, open the `Startup.cs` file. This file is where you’ll configure your application’s services and dependencies.
- Configure Services in the `ConfigureServices` Method:
– Inside the `Startup` class, you’ll find a method named `ConfigureServices`. This is where you register your services and dependencies with the built-in dependency injection container. You can use various methods like `AddTransient`, `AddScoped`, and `AddSingleton` to register services with different lifetimes.
```csharp public void ConfigureServices(IServiceCollection services) { services.AddTransient<ITransientService, TransientService>(); services.AddScoped<IScopedService, ScopedService>(); services.AddSingleton<ISingletonService, SingletonService>(); } ```
In the example above, we’ve registered three services with different lifetimes: transient, scoped, and singleton. The choice of lifetime depends on how you want the service to be created and shared within your application.
- Accessing Configuration Settings:
– You can also configure your application’s settings and options in the `ConfigureServices` method. For example, if you need to access settings from `appsettings.json`, you can bind them to strongly-typed classes:
```csharp public void ConfigureServices(IServiceCollection services) { services.Configure<MyOptions>(Configuration.GetSection("MyOptions")); } ```
- Additional Configuration: Depending on your application’s needs, you may need to configure other services like authentication, authorization, middleware, and database connections in the `ConfigureServices` method.
- Using Dependency Injection:
– Once services are registered, you can use constructor injection to access them in your controllers, services, and other components of your application. The ASP.NET Core framework will automatically resolve and inject the required dependencies when creating instances of your classes.
```csharp public class MyController : Controller { private readonly ITransientService _transientService; public MyController(ITransientService transientService) { _transientService = transientService; } // Use _transientService in controller actions... } ```
By configuring services and dependencies in ASP.NET Core, you establish the foundation for your application to work with various components and third-party libraries. This helps promote modularity, testability, and maintainability while providing a clean and organized way to manage your application’s core functionality.
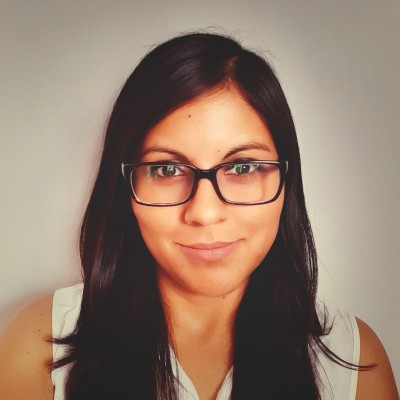
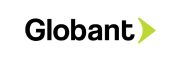