What is a constructor in C#?
In C#, a constructor is a special method within a class that gets called automatically when an instance (object) of that class is created. The primary purpose of a constructor is to initialize the object’s state and prepare it for use. Constructors play a vital role in object-oriented programming (OOP) as they ensure that objects start with valid and meaningful initial values.
Constructors have a few key characteristics:
- Same Name as the Class: Constructors have the same name as the class they belong to. This naming convention helps the compiler identify and invoke the correct constructor when an object is created.
- Initialization Code: Inside a constructor, you can include code that sets the initial values of the object’s fields, properties, and other members. This initialization process ensures that the object is in a valid state when it’s created.
- Multiple Constructors: A class can have multiple constructors with different parameter lists. This concept is known as constructor overloading. It allows you to create objects with various initializations, depending on your requirements.
Here’s a simple example of a class with constructors in C#:
```csharp public class Person { // Fields private string name; private int age; // Default Constructor (Parameterless) public Person() { name = "John Doe"; age = 30; } // Parameterized Constructor public Person(string personName, int personAge) { name = personName; age = personAge; } } ```
In the example above, the `Person` class has two constructors: a default constructor with no parameters and a parameterized constructor that allows you to specify the name and age when creating an instance. Constructors are essential for ensuring that objects are properly initialized, and they provide flexibility in how objects can be created and configured in C# programs.
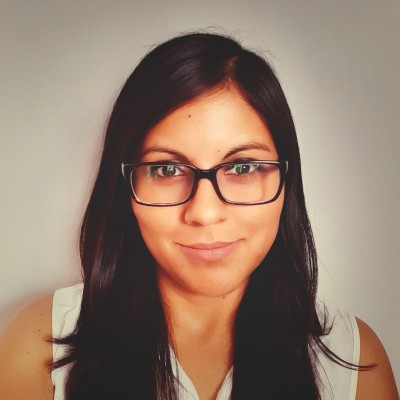
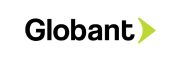