C# Q & A
What is a C# namespace?
Creating a class in C# is a fundamental step in object-oriented programming, as classes serve as the blueprint for creating objects and organizing your code. To create a C# class, follow these steps:
- Open Your Development Environment: First, open your C# development environment, such as Visual Studio or Visual Studio Code, where you plan to write and compile your code.
- Create a New C# Project or File: Depending on your project structure, you can either create a new C# project or add a new C# file to an existing project. In most cases, you’ll right-click on your project in the Solution Explorer and select “Add” > “Class” to create a new class file.
- Name Your Class: Give your class a meaningful and descriptive name. Follow common naming conventions, such as using PascalCase, where the first letter of each word is capitalized (e.g., `MyClass`).
- Define the Class: Inside the class file, use the `class` keyword followed by the name of your class to declare it. For example:
```csharp public class MyClass { // Class members (fields, properties, methods) go here } ```
- Add Members to Your Class: Within the class declaration, you can define class members, such as fields, properties, methods, and constructors. These members define the behavior and data associated with your class.
- Compile and Use Your Class: Save the class file, and compile your project to ensure there are no syntax errors. Once your class is successfully compiled, you can create instances of it in other parts of your code and use its members as needed.
Here’s a simple example of a C# class with a constructor and a method:
```csharp public class Person { // Fields (data members) private string name; private int age; // Constructor public Person(string personName, int personAge) { name = personName; age = personAge; } // Method public void DisplayInfo() { Console.WriteLine($"Name: {name}, Age: {age}"); } } ```
Creating classes is a fundamental aspect of C# development, enabling you to encapsulate data and behavior in a structured manner. By following these steps, you can define and use classes to build complex and organized applications effectively.
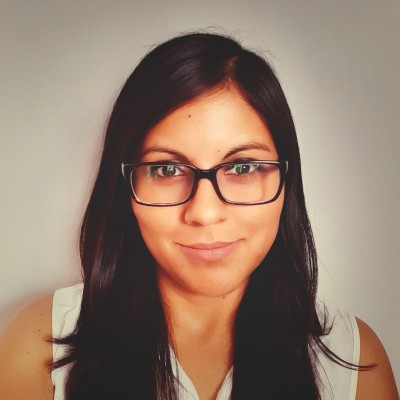
Previously at
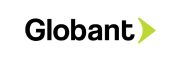
Experienced Backend Developer with 6 years of experience in C#. Proficient in C#, .NET, and Java.Proficient in REST web services and web app development.