How to create a RESTful API in C#?
Creating a RESTful API in C# involves several steps and best practices to ensure that your API is efficient, scalable, and adheres to REST (Representational State Transfer) principles. Here’s a concise guide on how to create a RESTful API in C#:
- Choose a Technology Stack:
Decide on the technology stack for building your API. ASP.NET Core is a popular choice for C# developers due to its cross-platform support, high performance, and extensive ecosystem.
- Set Up Your Development Environment:
Install the necessary tools, including Visual Studio or Visual Studio Code, and the .NET SDK. Create a new ASP.NET Core project with the API template.
- Define API Endpoints:
Identify the resources your API will expose and design the URL structure (routes) for accessing these resources. Each resource should have a unique URL, and HTTP verbs (GET, POST, PUT, DELETE) should be used to perform actions on them.
- Create Controllers:
Implement controllers to handle incoming HTTP requests. In ASP.NET Core, controllers are C# classes that contain action methods responsible for processing requests, interacting with the data, and returning responses.
- Use Attribute Routing:
Decorate your controller actions with attributes like `[HttpGet]`, `[HttpPost]`, `[HttpPut]`, and `[HttpDelete]` to specify the HTTP verb they respond to. Use attribute routing to define custom route patterns.
- Data Access Layer:
Implement a data access layer to interact with your data source, such as a database or external services. You can use Entity Framework Core, Dapper, or other data access libraries for this purpose.
- Serialization and Deserialization:
Ensure that data sent to and received from the API is properly serialized and deserialized. ASP.NET Core includes built-in support for JSON serialization, but you can also handle other formats like XML or custom formats.
- Input Validation:
Implement input validation to protect your API from malicious or invalid data. Use model validation attributes and consider using input validation libraries.
- Authentication and Authorization:
Secure your API by implementing authentication and authorization mechanisms. ASP.NET Core provides authentication middleware and supports various authentication providers, such as JWT (JSON Web Tokens).
- Testing:
Write unit tests and integration tests to verify the functionality and reliability of your API. Test various scenarios, including error handling and edge cases.
- Documentation:
Create comprehensive API documentation to help developers understand how to use your API. Tools like Swagger can assist in generating interactive API documentation.
- Error Handling:
Implement consistent error handling and return meaningful error responses with appropriate HTTP status codes.
- Deployment and Hosting:
Deploy your API to a hosting environment, such as Azure, AWS, or a dedicated server. Configure DNS and security settings as needed.
- Monitoring and Scaling:
Implement monitoring to track API performance, usage, and errors. Consider load balancing and scaling strategies to handle increased traffic.
By following these steps and best practices, you can create a robust and RESTful API in C#. RESTful APIs are widely used for building web services, mobile app backends, and other distributed systems, and adhering to REST principles ensures interoperability and simplicity in your API design.
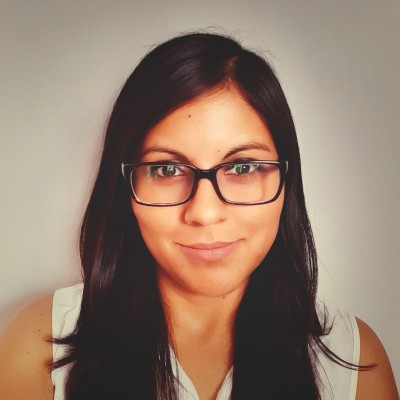
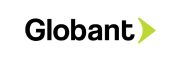