How to create and use enums in C#?
Creating and using enums (enumerations) in C# is a way to define named constant values for a specific set of related items or options. Enums provide clarity and readability to your code by giving meaningful names to integer values. Here’s a step-by-step guide on how to create and use enums in C#:
- Enum Declaration:
To create an enum, use the `enum` keyword followed by a unique identifier for your enum type and a set of named constants enclosed in curly braces. For example:
```csharp public enum DaysOfWeek { Sunday, Monday, Tuesday, Wednesday, Thursday, Friday, Saturday } ```
- Enum Values:
In the enum declaration above, `DaysOfWeek` is the name of the enum, and it contains seven named constants representing the days of the week. These constants are automatically assigned values starting from 0 for the first item (`Sunday`) and incrementing by 1 for each subsequent item.
- Using Enums:
You can use enums in your code by referencing them with the enum type name and the dot notation. For example:
```csharp DaysOfWeek today = DaysOfWeek.Wednesday; Console.WriteLine("Today is " + today); // Output: Today is Wednesday ```
- Enum Values as Integers:
Under the hood, enum values are stored as integers, so you can cast between enum values and integers. For example:
```csharp int dayNumber = (int)DaysOfWeek.Friday; // dayNumber will be 5 ```
- Enum Parsing and Conversion:
You can parse strings to enum values using `Enum.Parse` and convert enum values to strings using `Enum.ToString`. For instance:
```csharp string dayString = "Thursday"; DaysOfWeek parsedDay = (DaysOfWeek)Enum.Parse(typeof(DaysOfWeek), dayString); ```
Enums in C# are valuable for enhancing code readability, making it easier to work with named constants rather than raw numeric values. They are commonly used for defining options, states, or categories in your code, providing meaningful names for specific values.
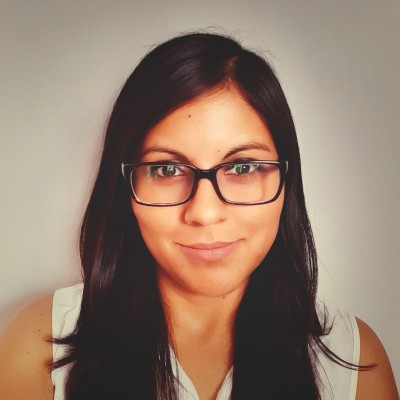
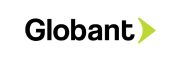